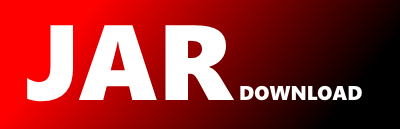
org.parse4j.operation.AddOperation Maven / Gradle / Ivy
The newest version!
package org.parse4j.operation;
import java.util.ArrayList;
import java.util.Collection;
import org.json.JSONException;
import org.json.JSONObject;
import org.parse4j.ParseObject;
import org.parse4j.encode.ParseObjectEncodingStrategy;
import org.parse4j.util.ParseEncoder;
public class AddOperation implements ParseFieldOperation {
protected final ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy