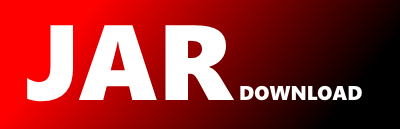
com.github.thinkerou.karate.message.Writer Maven / Gradle / Ivy
package com.github.thinkerou.karate.message;
import java.util.List;
import java.util.logging.Logger;
import com.google.protobuf.InvalidProtocolBufferException;
import com.google.protobuf.Message;
import com.google.protobuf.util.JsonFormat;
import io.grpc.stub.StreamObserver;
/**
* Writer
*
* A StreamObserver which writes the contents of the received messages to an Output.
* The messages are writting in a newline-separated json format.
*
* @author thinkerou
*/
public final class Writer implements StreamObserver {
private static final Logger logger = Logger.getLogger(Writer.class.getName());
private final JsonFormat.Printer jsonPrinter;
private final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy