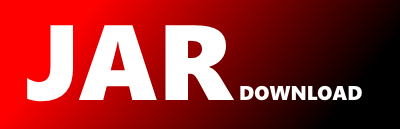
com.github.thinkerou.demo.helloworld.HelloWorldClient Maven / Gradle / Ivy
package com.github.thinkerou.demo.helloworld;
import io.grpc.ManagedChannel;
import io.grpc.ManagedChannelBuilder;
import io.grpc.StatusRuntimeException;
import java.util.List;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import java.util.logging.Level;
import java.util.logging.Logger;
import com.google.gson.Gson;
import com.google.protobuf.InvalidProtocolBufferException;
import com.google.protobuf.util.JsonFormat;
/**
* HelloWorld is a simple client that requests a greeting from the HelloWorldServerMain.
*
* Source from: https://github.com/grpc/grpc-java/tree/master/examples/src/main/java/io/grpc/examples
*
* @author thinkerou
*/
public class HelloWorldClient {
private static final Logger logger = Logger.getLogger(HelloWorldClient.class.getName());
private final ManagedChannel channel;
private final GreeterGrpc.GreeterBlockingStub blockingStub;
/**
* Construct client connecting to HelloWorld server at host:port.
*/
public HelloWorldClient(String host, int port) {
this(ManagedChannelBuilder.forAddress(host, port)
// Channels are secure by default (via SSL/TLS).
// For the example we disable TLS to avoid needing certificates.
.usePlaintext()
.build());
}
/**
* Construct client for accessing HelloWorld server using the existing channel.
*/
HelloWorldClient(ManagedChannel channel) {
this.channel = channel;
blockingStub = GreeterGrpc.newBlockingStub(channel);
}
public void shutdown() throws InterruptedException {
logger.log(Level.INFO, "shutdown grpc channel");
channel.shutdown().awaitTermination(5, TimeUnit.SECONDS);
}
/**
* Say hello to server.
*/
public String greet(String payload) {
Gson gson = new Gson();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy