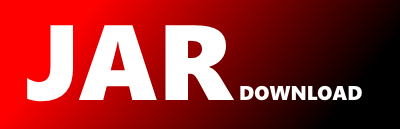
com.github.thinkerou.demo.helloworld.GreeterGrpc Maven / Gradle / Ivy
package com.github.thinkerou.demo.helloworld;
import static io.grpc.MethodDescriptor.generateFullMethodName;
import static io.grpc.stub.ClientCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ClientCalls.asyncClientStreamingCall;
import static io.grpc.stub.ClientCalls.asyncServerStreamingCall;
import static io.grpc.stub.ClientCalls.asyncUnaryCall;
import static io.grpc.stub.ClientCalls.blockingServerStreamingCall;
import static io.grpc.stub.ClientCalls.blockingUnaryCall;
import static io.grpc.stub.ClientCalls.futureUnaryCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall;
/**
*
* The greeting service definition.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.13.1)",
comments = "Source: demo/helloworld.proto")
public final class GreeterGrpc {
private GreeterGrpc() {}
public static final String SERVICE_NAME = "helloworld.Greeter";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getSayHelloMethod;
public static io.grpc.MethodDescriptor getSayHelloMethod() {
io.grpc.MethodDescriptor getSayHelloMethod;
if ((getSayHelloMethod = GreeterGrpc.getSayHelloMethod) == null) {
synchronized (GreeterGrpc.class) {
if ((getSayHelloMethod = GreeterGrpc.getSayHelloMethod) == null) {
GreeterGrpc.getSayHelloMethod = getSayHelloMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"helloworld.Greeter", "SayHello"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.HelloReply.getDefaultInstance()))
.setSchemaDescriptor(new GreeterMethodDescriptorSupplier("SayHello"))
.build();
}
}
}
return getSayHelloMethod;
}
private static volatile io.grpc.MethodDescriptor getAgainSayHelloMethod;
public static io.grpc.MethodDescriptor getAgainSayHelloMethod() {
io.grpc.MethodDescriptor getAgainSayHelloMethod;
if ((getAgainSayHelloMethod = GreeterGrpc.getAgainSayHelloMethod) == null) {
synchronized (GreeterGrpc.class) {
if ((getAgainSayHelloMethod = GreeterGrpc.getAgainSayHelloMethod) == null) {
GreeterGrpc.getAgainSayHelloMethod = getAgainSayHelloMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"helloworld.Greeter", "AgainSayHello"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.AgainHelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.AgainHelloReply.getDefaultInstance()))
.setSchemaDescriptor(new GreeterMethodDescriptorSupplier("AgainSayHello"))
.build();
}
}
}
return getAgainSayHelloMethod;
}
private static volatile io.grpc.MethodDescriptor getSayHelloBiStreamingMethod;
public static io.grpc.MethodDescriptor getSayHelloBiStreamingMethod() {
io.grpc.MethodDescriptor getSayHelloBiStreamingMethod;
if ((getSayHelloBiStreamingMethod = GreeterGrpc.getSayHelloBiStreamingMethod) == null) {
synchronized (GreeterGrpc.class) {
if ((getSayHelloBiStreamingMethod = GreeterGrpc.getSayHelloBiStreamingMethod) == null) {
GreeterGrpc.getSayHelloBiStreamingMethod = getSayHelloBiStreamingMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(
"helloworld.Greeter", "SayHelloBiStreaming"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.HelloReply.getDefaultInstance()))
.setSchemaDescriptor(new GreeterMethodDescriptorSupplier("SayHelloBiStreaming"))
.build();
}
}
}
return getSayHelloBiStreamingMethod;
}
private static volatile io.grpc.MethodDescriptor getSayHelloClientStreamingMethod;
public static io.grpc.MethodDescriptor getSayHelloClientStreamingMethod() {
io.grpc.MethodDescriptor getSayHelloClientStreamingMethod;
if ((getSayHelloClientStreamingMethod = GreeterGrpc.getSayHelloClientStreamingMethod) == null) {
synchronized (GreeterGrpc.class) {
if ((getSayHelloClientStreamingMethod = GreeterGrpc.getSayHelloClientStreamingMethod) == null) {
GreeterGrpc.getSayHelloClientStreamingMethod = getSayHelloClientStreamingMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.CLIENT_STREAMING)
.setFullMethodName(generateFullMethodName(
"helloworld.Greeter", "SayHelloClientStreaming"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.HelloReply.getDefaultInstance()))
.setSchemaDescriptor(new GreeterMethodDescriptorSupplier("SayHelloClientStreaming"))
.build();
}
}
}
return getSayHelloClientStreamingMethod;
}
private static volatile io.grpc.MethodDescriptor getSayHelloServerStreamingMethod;
public static io.grpc.MethodDescriptor getSayHelloServerStreamingMethod() {
io.grpc.MethodDescriptor getSayHelloServerStreamingMethod;
if ((getSayHelloServerStreamingMethod = GreeterGrpc.getSayHelloServerStreamingMethod) == null) {
synchronized (GreeterGrpc.class) {
if ((getSayHelloServerStreamingMethod = GreeterGrpc.getSayHelloServerStreamingMethod) == null) {
GreeterGrpc.getSayHelloServerStreamingMethod = getSayHelloServerStreamingMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(
"helloworld.Greeter", "SayHelloServerStreaming"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.HelloRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.HelloReply.getDefaultInstance()))
.setSchemaDescriptor(new GreeterMethodDescriptorSupplier("SayHelloServerStreaming"))
.build();
}
}
}
return getSayHelloServerStreamingMethod;
}
private static volatile io.grpc.MethodDescriptor getGetFeatureMethod;
public static io.grpc.MethodDescriptor getGetFeatureMethod() {
io.grpc.MethodDescriptor getGetFeatureMethod;
if ((getGetFeatureMethod = GreeterGrpc.getGetFeatureMethod) == null) {
synchronized (GreeterGrpc.class) {
if ((getGetFeatureMethod = GreeterGrpc.getGetFeatureMethod) == null) {
GreeterGrpc.getGetFeatureMethod = getGetFeatureMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"helloworld.Greeter", "GetFeature"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.Point.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.Feature.getDefaultInstance()))
.setSchemaDescriptor(new GreeterMethodDescriptorSupplier("GetFeature"))
.build();
}
}
}
return getGetFeatureMethod;
}
private static volatile io.grpc.MethodDescriptor getListFeaturesMethod;
public static io.grpc.MethodDescriptor getListFeaturesMethod() {
io.grpc.MethodDescriptor getListFeaturesMethod;
if ((getListFeaturesMethod = GreeterGrpc.getListFeaturesMethod) == null) {
synchronized (GreeterGrpc.class) {
if ((getListFeaturesMethod = GreeterGrpc.getListFeaturesMethod) == null) {
GreeterGrpc.getListFeaturesMethod = getListFeaturesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(
"helloworld.Greeter", "ListFeatures"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.Rectangle.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.Feature.getDefaultInstance()))
.setSchemaDescriptor(new GreeterMethodDescriptorSupplier("ListFeatures"))
.build();
}
}
}
return getListFeaturesMethod;
}
private static volatile io.grpc.MethodDescriptor getRecordRouteMethod;
public static io.grpc.MethodDescriptor getRecordRouteMethod() {
io.grpc.MethodDescriptor getRecordRouteMethod;
if ((getRecordRouteMethod = GreeterGrpc.getRecordRouteMethod) == null) {
synchronized (GreeterGrpc.class) {
if ((getRecordRouteMethod = GreeterGrpc.getRecordRouteMethod) == null) {
GreeterGrpc.getRecordRouteMethod = getRecordRouteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.CLIENT_STREAMING)
.setFullMethodName(generateFullMethodName(
"helloworld.Greeter", "RecordRoute"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.Point.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.RouteSummary.getDefaultInstance()))
.setSchemaDescriptor(new GreeterMethodDescriptorSupplier("RecordRoute"))
.build();
}
}
}
return getRecordRouteMethod;
}
private static volatile io.grpc.MethodDescriptor getRouteChatMethod;
public static io.grpc.MethodDescriptor getRouteChatMethod() {
io.grpc.MethodDescriptor getRouteChatMethod;
if ((getRouteChatMethod = GreeterGrpc.getRouteChatMethod) == null) {
synchronized (GreeterGrpc.class) {
if ((getRouteChatMethod = GreeterGrpc.getRouteChatMethod) == null) {
GreeterGrpc.getRouteChatMethod = getRouteChatMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(
"helloworld.Greeter", "RouteChat"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.RouteNote.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.github.thinkerou.demo.helloworld.RouteNote.getDefaultInstance()))
.setSchemaDescriptor(new GreeterMethodDescriptorSupplier("RouteChat"))
.build();
}
}
}
return getRouteChatMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static GreeterStub newStub(io.grpc.Channel channel) {
return new GreeterStub(channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static GreeterBlockingStub newBlockingStub(
io.grpc.Channel channel) {
return new GreeterBlockingStub(channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static GreeterFutureStub newFutureStub(
io.grpc.Channel channel) {
return new GreeterFutureStub(channel);
}
/**
*
* The greeting service definition.
*
*/
public static abstract class GreeterImplBase implements io.grpc.BindableService {
/**
*
* Sends a greeting
*
*/
public void sayHello(com.github.thinkerou.demo.helloworld.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getSayHelloMethod(), responseObserver);
}
/**
*/
public void againSayHello(com.github.thinkerou.demo.helloworld.AgainHelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getAgainSayHelloMethod(), responseObserver);
}
/**
*
* Streams a many greetings
*
*/
public io.grpc.stub.StreamObserver sayHelloBiStreaming(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(getSayHelloBiStreamingMethod(), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver sayHelloClientStreaming(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(getSayHelloClientStreamingMethod(), responseObserver);
}
/**
*/
public void sayHelloServerStreaming(com.github.thinkerou.demo.helloworld.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getSayHelloServerStreamingMethod(), responseObserver);
}
/**
*
* A simple RPC.
* Obtains the feature at a given position.
* A feature with an empty name is returned if there's no feature at the given
* position.
*
*/
public void getFeature(com.github.thinkerou.demo.helloworld.Point request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetFeatureMethod(), responseObserver);
}
/**
*
* A server-to-client streaming RPC.
* Obtains the Features available within the given Rectangle. Results are
* streamed rather than returned at once (e.g. in a response message with a
* repeated field), as the rectangle may cover a large area and contain a
* huge number of features.
*
*/
public void listFeatures(com.github.thinkerou.demo.helloworld.Rectangle request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getListFeaturesMethod(), responseObserver);
}
/**
*
* A client-to-server streaming RPC.
* Accepts a stream of Points on a route being traversed, returning a
* RouteSummary when traversal is completed.
*
*/
public io.grpc.stub.StreamObserver recordRoute(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(getRecordRouteMethod(), responseObserver);
}
/**
*
* A Bidirectional streaming RPC.
* Accepts a stream of RouteNotes sent while a route is being traversed,
* while receiving other RouteNotes (e.g. from other users).
*
*/
public io.grpc.stub.StreamObserver routeChat(
io.grpc.stub.StreamObserver responseObserver) {
return asyncUnimplementedStreamingCall(getRouteChatMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getSayHelloMethod(),
asyncUnaryCall(
new MethodHandlers<
com.github.thinkerou.demo.helloworld.HelloRequest,
com.github.thinkerou.demo.helloworld.HelloReply>(
this, METHODID_SAY_HELLO)))
.addMethod(
getAgainSayHelloMethod(),
asyncUnaryCall(
new MethodHandlers<
com.github.thinkerou.demo.helloworld.AgainHelloRequest,
com.github.thinkerou.demo.helloworld.AgainHelloReply>(
this, METHODID_AGAIN_SAY_HELLO)))
.addMethod(
getSayHelloBiStreamingMethod(),
asyncBidiStreamingCall(
new MethodHandlers<
com.github.thinkerou.demo.helloworld.HelloRequest,
com.github.thinkerou.demo.helloworld.HelloReply>(
this, METHODID_SAY_HELLO_BI_STREAMING)))
.addMethod(
getSayHelloClientStreamingMethod(),
asyncClientStreamingCall(
new MethodHandlers<
com.github.thinkerou.demo.helloworld.HelloRequest,
com.github.thinkerou.demo.helloworld.HelloReply>(
this, METHODID_SAY_HELLO_CLIENT_STREAMING)))
.addMethod(
getSayHelloServerStreamingMethod(),
asyncServerStreamingCall(
new MethodHandlers<
com.github.thinkerou.demo.helloworld.HelloRequest,
com.github.thinkerou.demo.helloworld.HelloReply>(
this, METHODID_SAY_HELLO_SERVER_STREAMING)))
.addMethod(
getGetFeatureMethod(),
asyncUnaryCall(
new MethodHandlers<
com.github.thinkerou.demo.helloworld.Point,
com.github.thinkerou.demo.helloworld.Feature>(
this, METHODID_GET_FEATURE)))
.addMethod(
getListFeaturesMethod(),
asyncServerStreamingCall(
new MethodHandlers<
com.github.thinkerou.demo.helloworld.Rectangle,
com.github.thinkerou.demo.helloworld.Feature>(
this, METHODID_LIST_FEATURES)))
.addMethod(
getRecordRouteMethod(),
asyncClientStreamingCall(
new MethodHandlers<
com.github.thinkerou.demo.helloworld.Point,
com.github.thinkerou.demo.helloworld.RouteSummary>(
this, METHODID_RECORD_ROUTE)))
.addMethod(
getRouteChatMethod(),
asyncBidiStreamingCall(
new MethodHandlers<
com.github.thinkerou.demo.helloworld.RouteNote,
com.github.thinkerou.demo.helloworld.RouteNote>(
this, METHODID_ROUTE_CHAT)))
.build();
}
}
/**
*
* The greeting service definition.
*
*/
public static final class GreeterStub extends io.grpc.stub.AbstractStub {
private GreeterStub(io.grpc.Channel channel) {
super(channel);
}
private GreeterStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GreeterStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new GreeterStub(channel, callOptions);
}
/**
*
* Sends a greeting
*
*/
public void sayHello(com.github.thinkerou.demo.helloworld.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getSayHelloMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void againSayHello(com.github.thinkerou.demo.helloworld.AgainHelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getAgainSayHelloMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Streams a many greetings
*
*/
public io.grpc.stub.StreamObserver sayHelloBiStreaming(
io.grpc.stub.StreamObserver responseObserver) {
return asyncBidiStreamingCall(
getChannel().newCall(getSayHelloBiStreamingMethod(), getCallOptions()), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver sayHelloClientStreaming(
io.grpc.stub.StreamObserver responseObserver) {
return asyncClientStreamingCall(
getChannel().newCall(getSayHelloClientStreamingMethod(), getCallOptions()), responseObserver);
}
/**
*/
public void sayHelloServerStreaming(com.github.thinkerou.demo.helloworld.HelloRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(getSayHelloServerStreamingMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* A simple RPC.
* Obtains the feature at a given position.
* A feature with an empty name is returned if there's no feature at the given
* position.
*
*/
public void getFeature(com.github.thinkerou.demo.helloworld.Point request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetFeatureMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* A server-to-client streaming RPC.
* Obtains the Features available within the given Rectangle. Results are
* streamed rather than returned at once (e.g. in a response message with a
* repeated field), as the rectangle may cover a large area and contain a
* huge number of features.
*
*/
public void listFeatures(com.github.thinkerou.demo.helloworld.Rectangle request,
io.grpc.stub.StreamObserver responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(getListFeaturesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* A client-to-server streaming RPC.
* Accepts a stream of Points on a route being traversed, returning a
* RouteSummary when traversal is completed.
*
*/
public io.grpc.stub.StreamObserver recordRoute(
io.grpc.stub.StreamObserver responseObserver) {
return asyncClientStreamingCall(
getChannel().newCall(getRecordRouteMethod(), getCallOptions()), responseObserver);
}
/**
*
* A Bidirectional streaming RPC.
* Accepts a stream of RouteNotes sent while a route is being traversed,
* while receiving other RouteNotes (e.g. from other users).
*
*/
public io.grpc.stub.StreamObserver routeChat(
io.grpc.stub.StreamObserver responseObserver) {
return asyncBidiStreamingCall(
getChannel().newCall(getRouteChatMethod(), getCallOptions()), responseObserver);
}
}
/**
*
* The greeting service definition.
*
*/
public static final class GreeterBlockingStub extends io.grpc.stub.AbstractStub {
private GreeterBlockingStub(io.grpc.Channel channel) {
super(channel);
}
private GreeterBlockingStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GreeterBlockingStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new GreeterBlockingStub(channel, callOptions);
}
/**
*
* Sends a greeting
*
*/
public com.github.thinkerou.demo.helloworld.HelloReply sayHello(com.github.thinkerou.demo.helloworld.HelloRequest request) {
return blockingUnaryCall(
getChannel(), getSayHelloMethod(), getCallOptions(), request);
}
/**
*/
public com.github.thinkerou.demo.helloworld.AgainHelloReply againSayHello(com.github.thinkerou.demo.helloworld.AgainHelloRequest request) {
return blockingUnaryCall(
getChannel(), getAgainSayHelloMethod(), getCallOptions(), request);
}
/**
*/
public java.util.Iterator sayHelloServerStreaming(
com.github.thinkerou.demo.helloworld.HelloRequest request) {
return blockingServerStreamingCall(
getChannel(), getSayHelloServerStreamingMethod(), getCallOptions(), request);
}
/**
*
* A simple RPC.
* Obtains the feature at a given position.
* A feature with an empty name is returned if there's no feature at the given
* position.
*
*/
public com.github.thinkerou.demo.helloworld.Feature getFeature(com.github.thinkerou.demo.helloworld.Point request) {
return blockingUnaryCall(
getChannel(), getGetFeatureMethod(), getCallOptions(), request);
}
/**
*
* A server-to-client streaming RPC.
* Obtains the Features available within the given Rectangle. Results are
* streamed rather than returned at once (e.g. in a response message with a
* repeated field), as the rectangle may cover a large area and contain a
* huge number of features.
*
*/
public java.util.Iterator listFeatures(
com.github.thinkerou.demo.helloworld.Rectangle request) {
return blockingServerStreamingCall(
getChannel(), getListFeaturesMethod(), getCallOptions(), request);
}
}
/**
*
* The greeting service definition.
*
*/
public static final class GreeterFutureStub extends io.grpc.stub.AbstractStub {
private GreeterFutureStub(io.grpc.Channel channel) {
super(channel);
}
private GreeterFutureStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GreeterFutureStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new GreeterFutureStub(channel, callOptions);
}
/**
*
* Sends a greeting
*
*/
public com.google.common.util.concurrent.ListenableFuture sayHello(
com.github.thinkerou.demo.helloworld.HelloRequest request) {
return futureUnaryCall(
getChannel().newCall(getSayHelloMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture againSayHello(
com.github.thinkerou.demo.helloworld.AgainHelloRequest request) {
return futureUnaryCall(
getChannel().newCall(getAgainSayHelloMethod(), getCallOptions()), request);
}
/**
*
* A simple RPC.
* Obtains the feature at a given position.
* A feature with an empty name is returned if there's no feature at the given
* position.
*
*/
public com.google.common.util.concurrent.ListenableFuture getFeature(
com.github.thinkerou.demo.helloworld.Point request) {
return futureUnaryCall(
getChannel().newCall(getGetFeatureMethod(), getCallOptions()), request);
}
}
private static final int METHODID_SAY_HELLO = 0;
private static final int METHODID_AGAIN_SAY_HELLO = 1;
private static final int METHODID_SAY_HELLO_SERVER_STREAMING = 2;
private static final int METHODID_GET_FEATURE = 3;
private static final int METHODID_LIST_FEATURES = 4;
private static final int METHODID_SAY_HELLO_BI_STREAMING = 5;
private static final int METHODID_SAY_HELLO_CLIENT_STREAMING = 6;
private static final int METHODID_RECORD_ROUTE = 7;
private static final int METHODID_ROUTE_CHAT = 8;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final GreeterImplBase serviceImpl;
private final int methodId;
MethodHandlers(GreeterImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_SAY_HELLO:
serviceImpl.sayHello((com.github.thinkerou.demo.helloworld.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_AGAIN_SAY_HELLO:
serviceImpl.againSayHello((com.github.thinkerou.demo.helloworld.AgainHelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SAY_HELLO_SERVER_STREAMING:
serviceImpl.sayHelloServerStreaming((com.github.thinkerou.demo.helloworld.HelloRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_FEATURE:
serviceImpl.getFeature((com.github.thinkerou.demo.helloworld.Point) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_FEATURES:
serviceImpl.listFeatures((com.github.thinkerou.demo.helloworld.Rectangle) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_SAY_HELLO_BI_STREAMING:
return (io.grpc.stub.StreamObserver) serviceImpl.sayHelloBiStreaming(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_SAY_HELLO_CLIENT_STREAMING:
return (io.grpc.stub.StreamObserver) serviceImpl.sayHelloClientStreaming(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_RECORD_ROUTE:
return (io.grpc.stub.StreamObserver) serviceImpl.recordRoute(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_ROUTE_CHAT:
return (io.grpc.stub.StreamObserver) serviceImpl.routeChat(
(io.grpc.stub.StreamObserver) responseObserver);
default:
throw new AssertionError();
}
}
}
private static abstract class GreeterBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
GreeterBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.github.thinkerou.demo.helloworld.HelloWorldProto.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("Greeter");
}
}
private static final class GreeterFileDescriptorSupplier
extends GreeterBaseDescriptorSupplier {
GreeterFileDescriptorSupplier() {}
}
private static final class GreeterMethodDescriptorSupplier
extends GreeterBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
GreeterMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (GreeterGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new GreeterFileDescriptorSupplier())
.addMethod(getSayHelloMethod())
.addMethod(getAgainSayHelloMethod())
.addMethod(getSayHelloBiStreamingMethod())
.addMethod(getSayHelloClientStreamingMethod())
.addMethod(getSayHelloServerStreamingMethod())
.addMethod(getGetFeatureMethod())
.addMethod(getListFeaturesMethod())
.addMethod(getRecordRouteMethod())
.addMethod(getRouteChatMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy