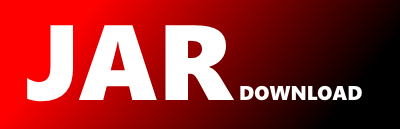
com.github.thorbenkuck.netcom2.logging.NetComLogging Maven / Gradle / Ivy
package com.github.thorbenkuck.netcom2.logging;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
/**
* This class is the central entry point for the NetCom2Logging api.
*
* Setting any logging instance using {@link #setLogging(Logging)}, will override the behaviour of any logger, created
* using {@link Logging#unified()}
*
* @version 1.0
* @see Logging
* @since 1.0
*/
public class NetComLogging implements Logging {
private static Logging logging = Logging.getDefault();
private static Lock loggingLock = new ReentrantLock(true);
public NetComLogging() {
instantiated(this);
}
private static Logging getLogging() {
try {
loggingLock.lock();
return logging;
} finally {
loggingLock.unlock();
}
}
/**
* By calling this method, the {@link Logging#unified()} and any Object using it, will receive the new Logging
*
* @param logging the Logging that should be used universally
*/
public static void setLogging(final Logging logging) {
if (NetComLogging.getLogging() == logging) {
throw new IllegalArgumentException("Cyclic dependency!");
}
try {
loggingLock.lock();
NetComLogging.logging = logging;
} finally {
loggingLock.unlock();
}
}
@Override
public boolean traceEnabled() {
return logging.traceEnabled();
}
@Override
public boolean debugEnabled() {
return logging.debugEnabled();
}
@Override
public boolean infoEnabled() {
return logging.infoEnabled();
}
@Override
public boolean warnEnabled() {
return logging.warnEnabled();
}
@Override
public boolean errorEnabled() {
return logging.errorEnabled();
}
@Override
public boolean fatalEnabled() {
return logging.fatalEnabled();
}
/**
* {@inheritDoc}
*/
@Override
public void trace(final Object o) {
NetComLogging.getLogging().trace(o);
}
/**
* {@inheritDoc}
*/
@Override
public void debug(final Object o) {
NetComLogging.getLogging().debug(o);
}
/**
* {@inheritDoc}
*/
@Override
public void info(final Object o) {
NetComLogging.getLogging().info(o);
}
/**
* {@inheritDoc}
*/
@Override
public void warn(final Object o) {
NetComLogging.getLogging().warn(o);
}
/**
* {@inheritDoc}
*/
@Override
public void error(final Object o) {
NetComLogging.getLogging().error(o);
}
/**
* {@inheritDoc}
*/
@Override
public void fatal(final Object o) {
NetComLogging.getLogging().fatal(o);
}
/**
* {@inheritDoc}
*/
@Override
public void catching(final Throwable throwable) {
NetComLogging.getLogging().catching(throwable);
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return "{Centralized Logging-Mechanism for NetCom2}";
}
}