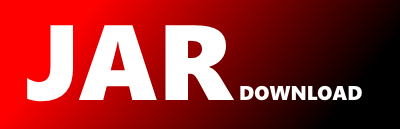
com.github.thorbenkuck.netcom2.utility.AsynchronousIterator Maven / Gradle / Ivy
package com.github.thorbenkuck.netcom2.utility;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.Queue;
/**
* This is an asynchronous Iterator. It works by making a copy of the specified collection,
* and only working on that.
*
* @param The type of the elements of this iterator
* @version 1.0
* @since 1.0
*/
final class AsynchronousIterator implements Iterator {
private final Queue core;
private final Collection source;
private final boolean removeAllowed;
private T currentElement;
AsynchronousIterator(final Collection collection) {
this(collection, false);
}
AsynchronousIterator(final Collection collection, boolean removeAllowed) {
NetCom2Utils.parameterNotNull(collection);
core = new LinkedList<>(collection);
this.source = collection;
this.removeAllowed = removeAllowed;
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasNext() {
return core.peek() != null;
}
/**
* {@inheritDoc}
*/
@Override
public T next() {
currentElement = core.poll();
return currentElement;
}
/**
* {@inheritDoc}
*/
@Override
public void remove() {
if (removeAllowed) {
source.remove(currentElement);
} else {
throw new UnsupportedOperationException("remove");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy