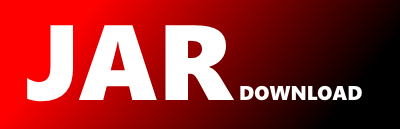
com.github.thorbenkuck.keller.repository.NotPresentHandlerImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of keller-repo Show documentation
Show all versions of keller-repo Show documentation
Keller is a universal base-package
The newest version!
package com.github.thorbenkuck.keller.repository;
import com.github.thorbenkuck.keller.datatypes.interfaces.QueuedAction;
import java.util.Collection;
import java.util.Objects;
import java.util.function.Consumer;
import java.util.function.Supplier;
final class NotPresentHandlerImpl implements NotPresentHandler {
private final ActionStack tActionStack;
NotPresentHandlerImpl(final ActionStack tActionStack) {
this.tActionStack = tActionStack;
}
@Override
public WayPoint throwException(final RuntimeException e) {
Objects.requireNonNull(e);
tActionStack.addIfNotPresent(() -> {throw e;});
return new WayPointImpl<>(tActionStack);
}
@Override
public WayPoint throwError(final Error error) {
Objects.requireNonNull(error);
tActionStack.addIfNotPresent(() -> {throw error;});
return new WayPointImpl<>(tActionStack);
}
@Override
public WayPoint getNullObject(final Supplier t) {
Objects.requireNonNull(t);
tActionStack.addIfNotPresent(() -> tActionStack.setPrimaryMatchingElement(t.get()));
return new WayPointImpl<>(tActionStack);
}
@Override
public WayPoint run(final Runnable runnable) {
Objects.requireNonNull(runnable);
tActionStack.addIfNotPresent(runnable);
return new WayPointImpl<>(tActionStack);
}
@Override
public WayPoint run(final QueuedAction queuedAction) {
Objects.requireNonNull(queuedAction);
tActionStack.addIfNotPresent(() -> {
queuedAction.doBefore();
queuedAction.doAction();
queuedAction.doAfter();
});
return new WayPointImpl<>(tActionStack);
}
@Override
public WayPoint handleAllOfSameType(final Consumer> consumer) {
Objects.requireNonNull(consumer);
tActionStack.addIfNotPresent(() -> {
consumer.accept(tActionStack.getMatchingObjects());
});
return new WayPointImpl<>(tActionStack);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy