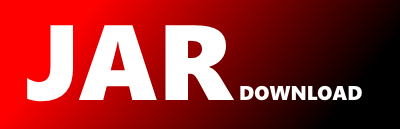
io.github.thunderz99.cosmos.CosmosDatabase Maven / Gradle / Ivy
Show all versions of java-cosmos Show documentation
package io.github.thunderz99.cosmos;
import java.util.List;
import io.github.thunderz99.cosmos.condition.Aggregate;
import io.github.thunderz99.cosmos.condition.Condition;
import io.github.thunderz99.cosmos.dto.CosmosBulkResult;
import io.github.thunderz99.cosmos.dto.PartialUpdateOption;
import io.github.thunderz99.cosmos.v4.PatchOperations;
/**
* Class representing a nosql database interface.
*
*
* Can do document' CRUD and find. Supports cosmosdb and mongodb
*
*/
public interface CosmosDatabase {
/**
* Create a document
*
* @param coll collection name
* @param data data object
* @param partition partition name
* @return CosmosDocument instance
* @throws Exception Cosmos Client Exception
*/
public CosmosDocument create(String coll, Object data, String partition) throws Exception;
/**
* Create a document using default partition
*
* @param coll collection name
* @param data data Object
* @return CosmosDocument instance
* @throws Exception Cosmos client exception
*/
default public CosmosDocument create(String coll, Object data) throws Exception {
return create(coll, data, coll);
}
/**
* @param coll collection name
* @param id id of the document
* @param partition partition name
* @return CosmosDocument instance
* @throws Exception Throw 404 Not Found Exception if object not exist
*/
public CosmosDocument read(String coll, String id, String partition) throws Exception;
/**
* Read a document by coll and id
*
* @param coll collection name
* @param id id of document
* @return CosmosDocument instance
* @throws Exception Throw 404 Not Found Exception if object not exist
*/
default public CosmosDocument read(String coll, String id) throws Exception {
return read(coll, id, coll);
}
/**
* Read a document by coll and id. Return null if object not exist
*
* @param coll collection name
* @param id id of document
* @param partition partition name
* @return CosmosDocument instance
* @throws Exception Cosmos client exception
*/
public CosmosDocument readSuppressing404(String coll, String id, String partition) throws Exception;
/**
* Read a document by coll and id. Return null if object not exist
*
* @param coll collection name
* @param id id of document
* @return CosmosDocument instance
* @throws Exception Cosmos client exception
*/
default public CosmosDocument readSuppressing404(String coll, String id) throws Exception {
return readSuppressing404(coll, id, coll);
}
/**
* Update existing data. if not exist, throw Not Found Exception.
*
* @param coll collection name
* @param data data object
* @param partition partition name
* @return CosmosDocument instance
* @throws Exception Cosmos client exception
*/
public CosmosDocument update(String coll, Object data, String partition) throws Exception;
/**
* Update existing data. if not exist, throw Not Found Exception.
*
* @param coll collection name
* @param data data object
* @return CosmosDocument instance
* @throws Exception Cosmos client exception
*/
default public CosmosDocument update(String coll, Object data) throws Exception {
return update(coll, data, coll);
}
/**
* Partial update existing data(Simple version). Input is a map, and the key/value in the map would be patched to the target document in SET mode.
*
*
* see partial update official docs
*
*
* If you want more complex partial update / patch features, please use patch(TODO) method, which supports ADD / SET / REPLACE / DELETE / INCREMENT and etc.
*
*
* @param coll collection name
* @param id id of document
* @param data data object
* @param partition partition name
* @return CosmosDocument instance
* @throws Exception Cosmos client exception. If not exist, throw Not Found Exception.
*/
default public CosmosDocument updatePartial(String coll, String id, Object data, String partition)
throws Exception {
return updatePartial(coll, id, data, partition, new PartialUpdateOption());
}
/**
* Partial update existing data(Simple version). Input is a map, and the key/value in the map would be patched to the target document in SET mode.
*
*
* see partial update official docs
*
*
* If you want more complex partial update / patch features, please use patch(TODO) method, which supports ADD / SET / REPLACE / DELETE / INCREMENT and etc.
*
*
* @param coll collection name
* @param id id of document
* @param data data object
* @param partition partition name
* @param option partial update option
* @return CosmosDocument instance
* @throws Exception Cosmos client exception. If not exist, throw Not Found Exception.
*/
public CosmosDocument updatePartial(String coll, String id, Object data, String partition, PartialUpdateOption option)
throws Exception;
/**
* Update existing data. Partial update supported(Only 1st json hierarchy supported). If not exist, throw Not Found Exception.
*
*
* see partial update official docs
*
*
* @param coll collection name
* @param id id of document
* @param data data object
* @return CosmosDocument instance
* @throws Exception Cosmos client exception
*/
default public CosmosDocument updatePartial(String coll, String id, Object data) throws Exception {
return updatePartial(coll, id, data, coll);
}
/**
* Update existing data. Create a new one if not exist. "id" field must be contained in data.
*
* @param coll collection name
* @param data data object
* @param partition partition name
* @return CosmosDocument instance
* @throws Exception Cosmos client exception
*/
public CosmosDocument upsert(String coll, Object data, String partition) throws Exception;
/**
* Update existing data. Create a new one if not exist. "id" field must be contained in data.
*
* @param coll collection name
* @param data data object
* @return CosmosDocument instance
* @throws Exception Cosmos client exception
*/
default public CosmosDocument upsert(String coll, Object data) throws Exception {
return upsert(coll, data, coll);
}
/**
* Delete a document. Do nothing if object not exist
*
* @param coll collection name
* @param id id of document
* @param partition partition name
* @return CosmosDatabase instance
* @throws Exception Cosmos client exception
*/
public CosmosDatabase delete(String coll, String id, String partition) throws Exception;
/**
* find data by condition
*
* {@code
* var cond = Condition.filter(
* "id>=", "id010", // id greater or equal to 'id010'
* "lastName", "Banks" // last name equal to Banks
* )
* .order("lastName", "ASC") //optional order
* .offset(0) //optional offset
* .limit(100); //optional limit
*
* var users = db.find("Collection1", cond, "Users").toList(User.class);
*
* }
*
* @param coll collection name
* @param cond condition to find
* @param partition partition name
* @return CosmosDocumentList
* @throws Exception Cosmos client exception
*/
public CosmosDocumentList find(String coll, Condition cond, String partition) throws Exception;
/**
* find data by condition (partition is default to the same name as the coll or ignored when crossPartition is true)
*
* {@code
* var cond = Condition.filter(
* "id>=", "id010", // id greater or equal to 'id010'
* "lastName", "Banks" // last name equal to Banks
* )
* .order("lastName", "ASC") //optional order
* .offset(0) //optional offset
* .limit(100); //optional limit
*
* var users = db.find("Collection1", cond).toList(User.class);
*
* }
*
* @param coll collection name
* @param cond condition to find
* @return CosmosDocumentList
* @throws Exception Cosmos client exception
*/
default public CosmosDocumentList find(String coll, Condition cond) throws Exception {
return find(coll, cond, coll);
}
/**
* do an aggregate query by Aggregate and Condition
*
* {@code
*
* var aggregate = Aggregate.function("COUNT(1) AS facetCount").groupBy("location", "gender");
* var cond = Condition.filter(
* "age>=", "20",
* );
*
* var result = db.aggregate("Collection1", aggregate, cond, "Users").toMap();
*
* }
*
* @param coll collection name
* @param aggregate Aggregate function and groupBys
* @param cond condition to find
* @param partition partition name
* @return CosmosDocumentList
* @throws Exception Cosmos client exception
*/
public CosmosDocumentList aggregate(String coll, Aggregate aggregate, Condition cond, String partition) throws Exception;
/**
* do an aggregate query by Aggregate and empty condition
*
* {@code
*
* var aggregate = Aggregate.function("COUNT(1) AS facetCount").groupBy("location", "gender");
* var result = db.aggregate("Collection1", aggregate, "Users").toMap();
*
* }
*
* @param coll collection name
* @param aggregate Aggregate function and groupBys
* @param partition partition name
* @return CosmosDocumentList
* @throws Exception Cosmos client exception
*/
default public CosmosDocumentList aggregate(String coll, Aggregate aggregate, String partition) throws Exception {
return aggregate(coll, aggregate, Condition.filter(), partition);
}
/**
* do an aggregate query by Aggregate and Condition (partition default to the same as coll or ignored when crossPartition is true)
*
* {@code
*
* var aggregate = Aggregate.function("COUNT(1) AS facetCount").groupBy("location", "gender");
* var cond = Condition.filter(
* "age>=", "20",
* );
*
* var result = db.aggregate("Collection1", aggregate, cond).toMap();
*
* }
*
* @param coll collection name
* @param aggregate Aggregate function and groupBys
* @param cond condition to find
* @return CosmosDocumentList
* @throws Exception Cosmos client exception
*/
default public CosmosDocumentList aggregate(String coll, Aggregate aggregate, Condition cond) throws Exception {
return aggregate(coll, aggregate, cond, coll);
}
/**
* count data by condition
*
* {@code
* var cond = Condition.filter(
* "id>=", "id010", // id greater or equal to 'id010'
* "lastName", "Banks" // last name equal to Banks
* );
*
* var count = db.count("Collection1", cond, "Users");
*
* }
*
* @param coll collection name
* @param cond condition to find
* @param partition partition name
* @return count of documents
* @throws Exception Cosmos client exception
*/
public int count(String coll, Condition cond, String partition) throws Exception;
/**
* Increment a number field of a document using json path format(e.g. "/count")
*
*
* see json patch format: json path
*
* see details of increment: supported operations: increment
*
*
* @param coll collection
* @param id item id
* @param path json path
* @param value amount of increment
* @param partition partition for item
* @return result item
* @throws Exception CosmosException doing increment
*/
public CosmosDocument increment(String coll, String id, String path, int value, String partition) throws Exception;
/**
* Patch data using JSON-Patch format. (max operations is 10)
*
*
* {@code
* //例:
* var operations = CosmosPatchOperations.create()
* // set or replace a new field
* .set("/contents/sex", "Male");
* // insert an item at index 1 for a field of array type
* .add("/skills/1", "TypeScript")
* var data = service.patch(host, id, operations);
* }
*
* supported operations
*
*
* @param coll collection
* @param id id of item
* @param operations operation list of JSON Patch
* @param partition partition
* @return CosmosDocument after patch
* @throws Exception CosmosException or other
*/
public CosmosDocument patch(String coll, String id, PatchOperations operations, String partition) throws Exception;
/**
* Get cosmos db account instance associated with this instance.
*
* @return cosmosAccount
*/
public Cosmos getCosmosAccount();
/**
* Get cosmos database name associated with this instance.
*
* @return database name
*/
public String getDatabaseName();
/**
* Create batch documents in a single transaction.
* Note: the maximum number of operations is 100.
*
* @param coll collection name
* @param data data object
* @param partition partition name
* @return CosmosDocument instances
* @throws Exception CosmosException
*/
public List batchCreate(String coll, List> data, String partition) throws Exception;
/**
* Upsert batch documents in a single transaction.
* Note: the maximum number of operations is 100.
*
* @param coll collection name
* @param data data object
* @param partition partition name
* @return CosmosDocument instances
* @throws Exception CosmosException
*/
public List batchUpsert(String coll, List> data, String partition) throws Exception;
/**
* Delete batch documents in a single transaction.
* Note: the maximum number of operations is 100.
*
* @param coll collection name
* @param data data object
* @param partition partition name
* @return CosmosDocument instances (only id)
* @throws Exception CosmosException
*/
public List batchDelete(String coll, List> data, String partition) throws Exception;
/**
* Bulk create documents.
* Note: Non-transaction. Have no number limit in theoretically.
*
* @param coll collection name
* @param data data object
* @param partition partition name
* @return CosmosBulkResult
*/
public CosmosBulkResult bulkCreate(String coll, List> data, String partition) throws Exception;
/**
* Bulk upsert documents
* Note: Non-transaction. Have no number limit in theoretically.
*
* @param coll collection name
* @param data data object
* @param partition partition name
* @return CosmosBulkResult
*/
public CosmosBulkResult bulkUpsert(String coll, List> data, String partition) throws Exception;
/**
* Bulk delete documents
* Note: Non-transaction. Have no number limit in theoretically.
*
* @param coll collection name
* @param data data object
* @param partition partition name
* @return CosmosBulkResult
*/
public CosmosBulkResult bulkDelete(String coll, List> data, String partition) throws Exception;
}