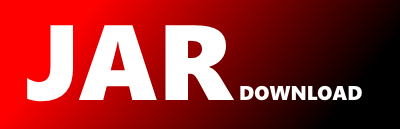
io.github.thunderz99.cosmos.util.MapUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-cosmos Show documentation
Show all versions of java-cosmos Show documentation
A lightweight Azure CosmosDB client for Java
package io.github.thunderz99.cosmos.util;
import java.util.LinkedHashMap;
import java.util.Map;
import org.apache.commons.lang3.StringUtils;
/**
* A simple util operate maps. e.g. convert a nested map to a flat map for partial update
*/
public class MapUtil {
/**
* Convert a traditional java map to a "/key1/key2/key3" : value format map. Used by v4 patch method.
*
*
* {@code
* // input:
* {
* "id": "ID001",
* "contents": {
* "name": "Tom",
* "age": 25
* }
* }
*
* // outpput:
* {
* "/id": "ID001",
* "/contents/name": "Tom",
* "/contents/age": 25
* }
* }
*
*
*
* @param map map to convert
* @return map flattened
*/
public static Map toFlatMap(Map map) {
return toFlatMap("", map, true);
}
/**
* Convert a traditional java map to a "key1.key2.key3" : value format map. Used by updatePartial in mongodb method.
*
*
* {@code
* // input:
* {
* "id": "ID001",
* "contents": {
* "name": "Tom",
* "age": 25
* }
* }
*
* // outpput:
* {
* "/id": "ID001",
* "/contents/name": "Tom",
* "/contents/age": 25
* }
* }
*
*
*
* @param map map to convert
* @return map flattened
*/
public static Map toFlatMapWithPeriod(Map map) {
return toFlatMap("", map, false);
}
static Map toFlatMap(String baseKey, Map map, boolean useSlash) {
if (map == null) {
return null;
}
if (map.isEmpty()) {
return new LinkedHashMap<>();
}
var ret = new LinkedHashMap();
for (var entry : map.entrySet()) {
var key = entry.getKey();
if (StringUtils.isEmpty(key)) {
// we do not support empty key at present
continue;
}
var value = entry.getValue();
var subKey = "";
if (useSlash) {
subKey = baseKey + "/" + key;
} else {
//use period
subKey = StringUtils.isEmpty(baseKey) ? key : baseKey + "." + key;
}
if (value instanceof Map) {
var subMap = (Map) value;
var flatSubMap = toFlatMap(subKey, subMap, useSlash);
ret.putAll(flatSubMap);
} else {
ret.put(subKey, value);
}
}
return ret;
}
/**
* Convert "/address/country/city" JSON Patch format to "address.country.city" mongo format
*
* @param path
* @return converted key
*/
public static String toPeriodKey(String path) {
if (path == null || path.isEmpty()) {
throw new IllegalArgumentException("Path cannot be null or empty");
}
// remove the heading slash and replace / to .
return path.startsWith("/") ? path.substring(1).replace("/", ".")
: path.replace("/", ".");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy