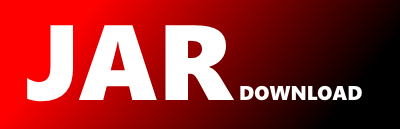
com.github.TKnudsen.ComplexDataObject.model.transformations.dimensionalityReduction.DimensionalityReduction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of complex-data-object Show documentation
Show all versions of complex-data-object Show documentation
A library that models real-world objects in Java, referred to as ComplexDataObjects. Other features: IO and preprocessing of ComplexDataObjects.
The newest version!
package com.github.TKnudsen.ComplexDataObject.model.transformations.dimensionalityReduction;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.function.ToDoubleBiFunction;
import com.github.TKnudsen.ComplexDataObject.data.features.numericalData.NumericalFeatureVector;
import com.github.TKnudsen.ComplexDataObject.model.processors.complexDataObject.DataTransformationCategory;
/**
*
* Basis for dimensionality reduction algorithms. Maintains generalizable data
* structures.
*
*
* Copyright: Copyright (c) 2012-2020 Juergen Bernard,
* https://github.com/TKnudsen/ComplexDataObject
*
*
* @author Juergen Bernard
* @version 1.05
*/
public abstract class DimensionalityReduction implements IDimensionalityReduction {
/**
* feature vectors for the model creation and dimensionality reduction
*/
protected List extends X> featureVectors;
/**
* used by many routines to calculate pairwise distances
*/
protected ToDoubleBiFunction super X, ? super X> distanceMeasure;
/**
* the dimensionality of the manifold to be learned
*/
protected int outputDimensionality;
protected Map mapping;
@Override
public DataTransformationCategory getDataTransformationCategory() {
return DataTransformationCategory.DIMENSION_REDUCTION;
}
@Override
public Map getMapping() {
return mapping;
}
@Override
public List transform(X inputObject) {
if (getMapping() == null)
throw new NullPointerException("mapping is null: dimensionality-reduction model not calculated yet?");
if (getMapping().get(inputObject) != null)
return Arrays.asList(new NumericalFeatureVector[] { getMapping().get(inputObject) });
System.err.println(
"DimensionalityRedutcion: feature vector identified that was not used for model building. null value added.");
List lst = new ArrayList<>();
lst.add(inputObject);
return transform(lst);
}
/**
* Uses the model to transform given data to the mapped space. Does not build a
* new model!
*/
@Override
public List transform(List inputObjects) {
if (inputObjects == null)
throw new NullPointerException("DimensionalityReduction: input objects must not be null");
if (inputObjects.size() == 0)
throw new IllegalArgumentException("DimensionalityReduction: input objects' size was 0");
if (getMapping() == null)
throw new NullPointerException("mapping is null: dimensionality-reduction model not calculated yet?");
List output = new ArrayList<>();
for (X x : inputObjects)
if (getMapping().containsKey(x))
output.add(getMapping().get(x));
else {
output.add(null);
System.err.println(
"DimensionalityRedutcion: input object identified that was not used for model building. null value added.");
}
return output;
}
@Override
public int getOutputDimensionality() {
return outputDimensionality;
}
public void setOutputDimensionality(int outputDimensionality) {
this.outputDimensionality = outputDimensionality;
this.mapping = null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy