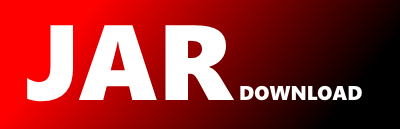
com.github.TKnudsen.ComplexDataObject.model.transformations.dimensionalityReduction.DimensionalityReductions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of complex-data-object Show documentation
Show all versions of complex-data-object Show documentation
A library that models real-world objects in Java, referred to as ComplexDataObjects. Other features: IO and preprocessing of ComplexDataObjects.
The newest version!
package com.github.TKnudsen.ComplexDataObject.model.transformations.dimensionalityReduction;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import com.github.TKnudsen.ComplexDataObject.data.features.AbstractFeatureVector;
import com.github.TKnudsen.ComplexDataObject.data.features.numericalData.NumericalFeatureVector;
import com.github.TKnudsen.ComplexDataObject.model.tools.DataConversion;
import com.github.TKnudsen.ComplexDataObject.model.tools.StatisticsSupport;
/**
*
* Title: DimensionalityReductions
*
*
*
* Description: little helpers to better cope with DimensionalityReduction
* results (mappings from highDim to lowDim).
*
*
* Copyright: Copyright (c) 2012-2020 Juergen Bernard,
* https://github.com/TKnudsen/ComplexDataObject
*
*
* @author Juergen Bernard
* @version 1.03
*/
public class DimensionalityReductions {
public static List mappingToCoordinatesList(Map mapping) {
List data = new ArrayList<>();
for (X x : mapping.keySet()) {
data.add(DataConversion.doublePrimitivesToArray(mapping.get(x).getVector()));
}
return data;
}
public static Map mappingToCoordinatesMap(Map mapping) {
Map data = new LinkedHashMap<>();
for (X x : mapping.keySet()) {
data.put(x, DataConversion.doublePrimitivesToArray(mapping.get(x).getVector()));
}
return data;
}
public static List mappingToNamesList(Map mapping) {
List data = new ArrayList<>();
for (X x : mapping.keySet()) {
data.add(mapping.get(x).getName());
}
return data;
}
/**
* statistics information for every output dimension. Used, e.g., to define axes
* in a scatter plot.
*
* @param mapping
* @return
*/
public static List mappingToStatisticsList(Map mapping) {
List> valuesPerDimension = new ArrayList<>();
for (X x : mapping.keySet()) {
double[] vector = mapping.get(x).getVector();
if (vector == null)
continue;
for (int i = 0; i < vector.length; i++) {
if (valuesPerDimension.size() <= i)
valuesPerDimension.add(new ArrayList<>());
valuesPerDimension.get(i).add(vector[i]);
}
}
List statistics = new ArrayList<>();
for (int i = 0; i < valuesPerDimension.size(); i++)
statistics.add(new StatisticsSupport(valuesPerDimension.get(i)));
return statistics;
}
/**
* normalizes the (low-dimensional) output of a mapping into 2D. uses a global
* min and max across all dimensions to preserve linearity. Modifies the given
* output mapping.
*
* @param mapping
*/
public static void normalizeMapping(Map mapping) {
// normalize feature vectors
double max = Double.NEGATIVE_INFINITY;
double min = Double.POSITIVE_INFINITY;
for (NumericalFeatureVector fv : mapping.values()) {
for (int d = 0; d < fv.getDimensions(); d++) {
min = Math.min(min, fv.getFeature(d).getFeatureValue());
max = Math.max(max, fv.getFeature(d).getFeatureValue());
}
}
double delta = max - min;
for (NumericalFeatureVector fv : mapping.values())
for (int d = 0; d < fv.getDimensions(); d++)
fv.getFeature(d).setFeatureValue((fv.getFeature(d).getFeatureValue() - min) / delta);
}
/**
* adds metadata from an input feature vector to the output feature vector. Also
* sets the input feature vector as the master object, thus providing a back
* link in the data processing chain.
*
* @param inputFeatureVector
* @param outputFeatureVector
*/
public static > void synchronizeFeatureVectorMetadata(X inputFeatureVector,
NumericalFeatureVector outputFeatureVector) {
for (String attribute : inputFeatureVector.keySet())
outputFeatureVector.add(attribute, inputFeatureVector.getAttribute(attribute));
outputFeatureVector.setMaster(inputFeatureVector);
outputFeatureVector.setName(inputFeatureVector.getName());
outputFeatureVector.setDescription(inputFeatureVector.getDescription());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy