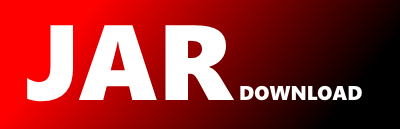
com.badlogic.gdx.backends.gwt.preloader.Preloader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gdx-backend-gwt Show documentation
Show all versions of gdx-backend-gwt Show documentation
Android/Desktop/iOS/HTML5 game development framework.
/*******************************************************************************
* Copyright 2011 See AUTHORS file.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package com.badlogic.gdx.backends.gwt.preloader;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileFilter;
import java.io.FilenameFilter;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import com.badlogic.gdx.Files.FileType;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.backends.gwt.GwtFileHandle;
import com.badlogic.gdx.backends.gwt.preloader.AssetDownloader.AssetLoaderListener;
import com.badlogic.gdx.backends.gwt.preloader.AssetFilter.AssetType;
import com.badlogic.gdx.files.FileHandle;
import com.badlogic.gdx.utils.Array;
import com.badlogic.gdx.utils.GdxRuntimeException;
import com.badlogic.gdx.utils.ObjectMap;
import com.google.gwt.core.client.GWT;
import com.google.gwt.dom.client.ImageElement;
public class Preloader {
private final AssetDownloader loader = new AssetDownloader();
public interface PreloaderCallback {
public void update(PreloaderState state);
public void error (String file);
}
public ObjectMap directories = new ObjectMap();
public ObjectMap images = new ObjectMap();
public ObjectMap audio = new ObjectMap();
public ObjectMap texts = new ObjectMap();
public ObjectMap binaries = new ObjectMap();
private ObjectMap stillToFetchAssets = new ObjectMap();
public static class Asset {
public Asset (String url, AssetType type, long size, String mimeType) {
this.url = url;
this.type = type;
this.size = size;
this.mimeType = mimeType;
}
public boolean succeed;
public boolean failed;
public boolean downloadStarted;
public long loaded;
public final String url;
public final AssetType type;
public final long size;
public final String mimeType;
}
public static class PreloaderState {
public PreloaderState(Array assets) {
this.assets = assets;
}
public long getDownloadedSize() {
long size = 0;
for (int i = 0; i < assets.size; i++) {
Asset asset = assets.get(i);
size += (asset.succeed || asset.failed) ? asset.size : Math.min(asset.size, asset.loaded);
}
return size;
}
public long getTotalSize() {
long size = 0;
for (int i = 0; i < assets.size; i++) {
Asset asset = assets.get(i);
size += asset.size;
}
return size;
}
public float getProgress() {
long total = getTotalSize();
return total == 0 ? 1 : (getDownloadedSize() / (float) total);
}
public boolean hasEnded() {
return getDownloadedSize() == getTotalSize();
}
public final Array assets;
}
public final String baseUrl;
public Preloader (String newBaseURL) {
baseUrl = newBaseURL;
// trigger copying of assets and creation of assets.txt
GWT.create(PreloaderBundle.class);
}
public void preload (final String assetFileUrl, final PreloaderCallback callback) {
loader.loadText(baseUrl + assetFileUrl, new AssetLoaderListener() {
@Override
public void onProgress (double amount) {
}
@Override
public void onFailure () {
callback.error(assetFileUrl);
}
@Override
public void onSuccess (String result) {
String[] lines = result.split("\n");
Array assets = new Array(lines.length);
for (String line : lines) {
String[] tokens = line.split(":");
if (tokens.length != 5) {
throw new GdxRuntimeException("Invalid assets description file.");
}
AssetType type = AssetType.Text;
if (tokens[0].equals("i")) type = AssetType.Image;
if (tokens[0].equals("b")) type = AssetType.Binary;
if (tokens[0].equals("a")) type = AssetType.Audio;
if (tokens[0].equals("d")) type = AssetType.Directory;
long size = Long.parseLong(tokens[2]);
if (type == AssetType.Audio && !loader.isUseBrowserCache()) {
size = 0;
}
Asset asset = new Asset(tokens[1].trim(), type, size, tokens[3]);
if (tokens[4].equals("1") || asset.url.startsWith("com/badlogic/"))
assets.add(asset);
else
stillToFetchAssets.put(asset.url, asset);
}
final PreloaderState state = new PreloaderState(assets);
for (int i = 0; i < assets.size; i++) {
final Asset asset = assets.get(i);
if (contains(asset.url)) {
asset.loaded = asset.size;
asset.succeed = true;
continue;
}
asset.downloadStarted = true;
loader.load(baseUrl + asset.url, asset.type, asset.mimeType, new AssetLoaderListener
© 2015 - 2025 Weber Informatics LLC | Privacy Policy