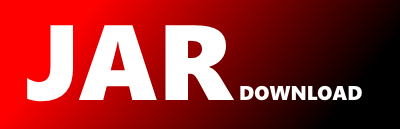
com.github.tommyettinger.ds.ObjectBag Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdkgdxds Show documentation
Show all versions of jdkgdxds Show documentation
Making libGDX's data structures implement JDK interfaces.
The newest version!
/*
* Copyright (c) 2023-2025 See AUTHORS file.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.tommyettinger.ds;
import com.github.tommyettinger.ds.support.util.ByteIterator;
import org.checkerframework.checker.nullness.qual.Nullable;
import java.util.Collection;
import java.util.Iterator;
/**
* An unordered List of T items. This allows efficient iteration via a reused iterator or via index.
* This class avoids a memory copy when removing elements (the last element is moved to the removed element's position).
* Items are permitted to change position in the ordering when any item is removed or added.
* Although this won't keep an order during modifications, you can {@link #sort()} the bag to ensure,
* if no modifications are made later, that the iteration will happen in sorted order.
*/
public class ObjectBag extends ObjectList {
/**
* Returns true if this implementation retains order, which it does not.
*
* @return false
*/
@Override
public boolean keepsOrder () {
return false;
}
/**
* Constructs an empty bag with an initial capacity of 10.
*/
public ObjectBag () {
super();
}
/**
* Constructs an empty bag with the specified initial capacity.
*
* @param initialCapacity the initial capacity of the bag
* @throws IllegalArgumentException if the specified initial capacity
* is negative
*/
public ObjectBag (int initialCapacity) {
super(initialCapacity);
}
/**
* Constructs a bag containing the elements of the specified
* collection, in the order they are returned by the collection's
* iterator.
*
* @param c the collection whose elements are to be placed into this bag
* @throws NullPointerException if the specified collection is null
*/
public ObjectBag (Collection extends T> c) {
super(c);
}
public ObjectBag (T[] a) {
super(a);
}
public ObjectBag (T[] a, int offset, int count) {
super(a, offset, count);
}
/**
* Creates a new instance containing the items in the specified iterator.
*
* @param coll an iterator that will have its remaining contents added to this
*/
public ObjectBag (Iterator extends T> coll) {
this();
addAll(coll);
}
/**
* Creates a new bag by copying {@code count} items from the given Ordered, starting at {@code offset} in that Ordered,
* into this.
*
* @param other another Ordered of the same type
* @param offset the first index in other's ordering to draw an item from
* @param count how many items to copy from other
*/
public ObjectBag (Ordered other, int offset, int count) {
super(other, offset, count);
}
/**
* This always adds {@code element} to the end of this bag's ordering; {@code index} is ignored.
*
* @param index ignored
* @param element element to be inserted
*/
@Override
public void add (int index, @Nullable T element) {
super.add(element);
}
/**
* This always adds {@code element} to the end of this bag's ordering; {@code index} is ignored.
* This is an alias for {@link #add(int, Object)} to improve compatibility with primitive lists.
*
* @param index ignored
* @param element element to be inserted
*/
@Override
public void insert (int index, @Nullable T element) {
super.add(element);
}
/**
* This removes the item at the given index and returns it, but also changes the ordering.
*
* @param index the index of the element to be removed, which must be non-negative and less than {@link #size()}
* @return the removed item
* @throws IndexOutOfBoundsException if the bag is empty
*/
@Override
public @Nullable T remove (int index) {
int size = size();
T value = super.set(index, get(size - 1));
super.remove(size - 1);
return value;
}
/**
* This removes the item at the given index and returns it, but also changes the ordering.
* This is an alias for {@link #remove(int)} to make the API the same for primitive lists.
*
* @param index must be non-negative and less than {@link #size()}
* @return the previously-held item at the given index
* @throws IndexOutOfBoundsException if the bag is empty
*/
@Override
public @Nullable T removeAt (int index) {
int size = size();
T value = super.set(index, get(size - 1));
super.remove(size - 1);
return value;
}
/**
* Uses == for comparison of the bags; does not compare their items.
*/
public boolean equalsIdentity (Object object) {
return object == this;
}
@Override
public int hashCode () {
int h = 1, n = size();
for (int i = 0; i < n; i++) {
h += get(i).hashCode();
}
return h;
}
/**
* Constructs an empty bag given the type as a generic type argument.
* This is usually less useful than just using the constructor, but can be handy
* in some code-generation scenarios when you don't know how many arguments you will have.
*
* @param the type of items; must be given explicitly
* @return a new bag containing nothing
*/
public static ObjectBag with () {
return new ObjectBag<>(0);
}
/**
* Creates a new ObjectBag that holds only the given item, but can be resized.
* @param item one T item
* @return a new ObjectBag that holds the given item
* @param the type of item, typically inferred
*/
public static ObjectBag with (T item) {
ObjectBag bag = new ObjectBag<>(1);
bag.add(item);
return bag;
}
/**
* Creates a new ObjectBag that holds only the given items, but can be resized.
* @param item0 a T item
* @param item1 a T item
* @return a new ObjectBag that holds the given items
* @param the type of item, typically inferred
*/
public static ObjectBag with (T item0, T item1) {
ObjectBag bag = new ObjectBag<>(2);
bag.add(item0, item1);
return bag;
}
/**
* Creates a new ObjectBag that holds only the given items, but can be resized.
* @param item0 a T item
* @param item1 a T item
* @param item2 a T item
* @return a new ObjectBag that holds the given items
* @param the type of item, typically inferred
*/
public static ObjectBag with (T item0, T item1, T item2) {
ObjectBag bag = new ObjectBag<>(3);
bag.add(item0, item1, item2);
return bag;
}
/**
* Creates a new ObjectBag that holds only the given items, but can be resized.
* @param item0 a T item
* @param item1 a T item
* @param item2 a T item
* @param item3 a T item
* @return a new ObjectBag that holds the given items
* @param the type of item, typically inferred
*/
public static ObjectBag with (T item0, T item1, T item2, T item3) {
ObjectBag bag = new ObjectBag<>(4);
bag.add(item0, item1, item2, item3);
return bag;
}
/**
* Creates a new ObjectBag that holds only the given items, but can be resized.
* @param item0 a T item
* @param item1 a T item
* @param item2 a T item
* @param item3 a T item
* @param item4 a T item
* @return a new ObjectBag that holds the given items
* @param the type of item, typically inferred
*/
public static ObjectBag with (T item0, T item1, T item2, T item3, T item4) {
ObjectBag bag = new ObjectBag<>(5);
bag.add(item0, item1, item2, item3);
bag.add(item4);
return bag;
}
/**
* Creates a new ObjectBag that holds only the given items, but can be resized.
* @param item0 a T item
* @param item1 a T item
* @param item2 a T item
* @param item3 a T item
* @param item4 a T item
* @param item5 a T item
* @return a new ObjectBag that holds the given items
* @param the type of item, typically inferred
*/
public static ObjectBag with (T item0, T item1, T item2, T item3, T item4, T item5) {
ObjectBag bag = new ObjectBag<>(6);
bag.add(item0, item1, item2, item3);
bag.add(item4, item5);
return bag;
}
/**
* Creates a new ObjectBag that holds only the given items, but can be resized.
* @param item0 a T item
* @param item1 a T item
* @param item2 a T item
* @param item3 a T item
* @param item4 a T item
* @param item5 a T item
* @param item6 a T item
* @return a new ObjectBag that holds the given items
* @param the type of item, typically inferred
*/
public static ObjectBag with (T item0, T item1, T item2, T item3, T item4, T item5, T item6) {
ObjectBag bag = new ObjectBag<>(7);
bag.add(item0, item1, item2, item3);
bag.add(item4, item5, item6);
return bag;
}
/**
* Creates a new ObjectBag that holds only the given items, but can be resized.
* @param item0 a T item
* @param item1 a T item
* @param item2 a T item
* @param item3 a T item
* @param item4 a T item
* @param item5 a T item
* @param item6 a T item
* @return a new ObjectBag that holds the given items
* @param the type of item, typically inferred
*/
public static ObjectBag with (T item0, T item1, T item2, T item3, T item4, T item5, T item6, T item7) {
ObjectBag bag = new ObjectBag<>(8);
bag.add(item0, item1, item2, item3);
bag.add(item4, item5, item6, item7);
return bag;
}
/**
* Creates a new ObjectBag that will hold the items in the given array or varargs.
* This overload will only be used when an array is supplied and the type of the
* items requested is the component type of the array, or if varargs are used and
* there are 9 or more arguments.
* @param varargs either 0 or more T items, or an array of T
* @return a new ObjectBag that holds the given T items
* @param the type of items, typically inferred by all the items being the same type
*/
@SafeVarargs
public static ObjectBag with (T... varargs) {
return new ObjectBag<>(varargs);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy