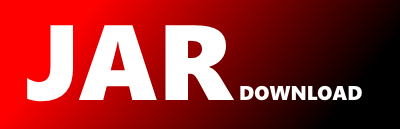
org.jungrapht.visualization.layout.algorithms.sugiyama.ConstructiveCycleRemoval Maven / Gradle / Ivy
The newest version!
package org.jungrapht.visualization.layout.algorithms.sugiyama;
import java.util.*;
import org.jgrapht.Graph;
import org.jgrapht.graph.DefaultGraphType;
import org.jgrapht.graph.DirectedAcyclicGraph;
import org.jgrapht.graph.builder.GraphBuilder;
import org.jgrapht.graph.builder.GraphTypeBuilder;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Remove cycles in the graph and place them in a collection of feedback edges. A later operation
* will reverse the direction of the feedback arcs for DAG processing. Once complete, the feedback
* arcs will be restored to their original direction.
*
* @param vertex type
* @param edge type
*/
public class ConstructiveCycleRemoval {
private static final Logger log = LoggerFactory.getLogger(ConstructiveCycleRemoval.class);
Collection feedbackArcs = new HashSet<>();
Graph graph;
Graph dag;
Comparator comparator;
public ConstructiveCycleRemoval(Graph graph) {
this(graph, (v1, v2) -> 0);
}
public ConstructiveCycleRemoval(Graph graph, Comparator comparator) {
this.graph = graph;
this.comparator = comparator;
this.dag = GraphTypeBuilder.forGraphType(DefaultGraphType.dag()).buildGraph();
GraphBuilder> builder =
(GraphBuilder>) DirectedAcyclicGraph.createBuilder(Object::new);
Graph dag2 = builder.build();
graph.vertexSet().forEach(dag::addVertex);
graph.vertexSet().forEach(dag2::addVertex);
List edgeList = new ArrayList<>(graph.edgeSet());
edgeList.sort(comparator);
for (E edge : edgeList) {
V source = graph.getEdgeSource(edge);
V target = graph.getEdgeTarget(edge);
try {
dag.addEdge(source, target, edge);
dag2.addEdge(source, target, edge);
} catch (Exception ex) {
feedbackArcs.add(edge);
}
}
if (log.isTraceEnabled()) {
log.trace("graph is {}", graph);
log.trace("dag is {}", dag);
log.trace(
"graph vertex sets are the same: {}", graph.vertexSet().containsAll(dag.vertexSet()));
log.trace("graph edge sets are the same: {}", graph.edgeSet().containsAll(dag.edgeSet()));
}
}
public Collection getFeedbackArcs() {
return this.feedbackArcs;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy