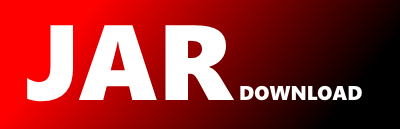
org.jungrapht.visualization.layout.algorithms.sugiyama.DFSCycleRemoval Maven / Gradle / Ivy
The newest version!
package org.jungrapht.visualization.layout.algorithms.sugiyama;
import java.util.*;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import org.jgrapht.Graph;
import org.jgrapht.Graphs;
import org.jgrapht.graph.builder.GraphTypeBuilder;
public class DFSCycleRemoval { //implements Function, Collection> {
private Graph graph;
Collection edges;
Set marked = new HashSet<>();
Stack stack = new Stack<>();
Set feedbackArcs = new HashSet<>();
Predicate edgePredicate;
Comparator comparator;
public DFSCycleRemoval(Graph graph) {
this(graph, (v1, v2) -> 0);
}
public DFSCycleRemoval(Graph graph, Comparator comparator) {
// make a copy of the graph
this.graph = GraphTypeBuilder.forGraph(graph).buildGraph();
Graphs.addGraph(this.graph, graph); // dest, source
this.edges = graph.edgeSet();
this.comparator = comparator;
removeCycles();
}
public void removeCycles() {
List vertices = graph.vertexSet().stream().sorted(comparator).collect(Collectors.toList());
// Collections.reverse(vertices);
for (V vertex : vertices) {
this.dfsRemove(vertex);
}
}
public void setEdgePredicate(Predicate edgePredicate) {
this.edgePredicate = edgePredicate;
}
private void dfsRemove(V vertex) {
if (this.marked.contains(vertex)) {
return;
}
this.marked.add(vertex);
this.stack.add(vertex);
List outgoingEdges =
graph
.outgoingEdgesOf(vertex)
.stream()
// .sorted(comparator)
.collect(Collectors.toList());
// Collections.reverse(outgoingEdges);
for (E edge : outgoingEdges) {
V target = graph.getEdgeTarget(edge);
if (this.stack.contains(target)) {
graph.removeEdge(edge);
feedbackArcs.add(edge);
} else if (!this.marked.contains(target)) {
this.dfsRemove(target);
}
}
this.stack.remove(vertex);
}
public Collection getFeedbackArcs() {
return this.feedbackArcs;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy