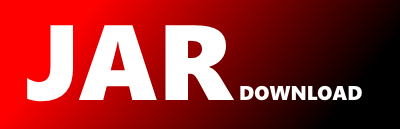
org.jungrapht.visualization.layout.algorithms.sugiyama.Unaligned Maven / Gradle / Ivy
The newest version!
package org.jungrapht.visualization.layout.algorithms.sugiyama;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import org.jungrapht.visualization.layout.model.Point;
import org.jungrapht.visualization.layout.model.Rectangle;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/** does not vertically align articulated edges. Centers each row according to the max row width */
public class Unaligned {
private static final Logger log = LoggerFactory.getLogger(Unaligned.class);
public static void centerPoints(
LV[][] layers,
Function vertexShapeFunction,
int horizontalOffset,
int verticalOffset) {
// ,
// Map, Point> vertexPointMap) {
Map rowWidthMap = new HashMap<>();
Map rowMaxHeightMap = new HashMap<>();
int layerIndex = 0;
for (LV[] layer : layers) {
int width = horizontalOffset;
int maxHeight = 0;
for (LV sugiyamaVertex : layer) {
if (!(sugiyamaVertex instanceof SyntheticLV)) {
Rectangle bounds = vertexShapeFunction.apply(sugiyamaVertex.getVertex());
width += bounds.width + horizontalOffset;
maxHeight = Math.max(maxHeight, (int) bounds.height);
} else {
width += horizontalOffset;
}
}
rowWidthMap.put(layerIndex, width);
rowMaxHeightMap.put(layerIndex, maxHeight);
layerIndex++;
}
int widestRowWidth = rowWidthMap.values().stream().mapToInt(v -> v).max().getAsInt();
int x = 0; //horizontalOffset;
int y = verticalOffset;
layerIndex = 0;
if (log.isTraceEnabled()) {
log.trace("layerMaxHeights {}", rowMaxHeightMap);
}
for (LV[] layer : layers) {
int previousVertexWidth = 0;
// offset against widest row
x += (widestRowWidth - rowWidthMap.get(layerIndex)) / 2;
y += rowMaxHeightMap.get(layerIndex) / 2;
if (layerIndex > 0) {
y += rowMaxHeightMap.get(layerIndex - 1) / 2;
}
for (LV sugiyamaVertex : layer) {
int vertexWidth = 0;
if (!(sugiyamaVertex instanceof SyntheticLV)) {
vertexWidth = (int) vertexShapeFunction.apply(sugiyamaVertex.getVertex()).width;
}
x += previousVertexWidth / 2 + vertexWidth / 2 + horizontalOffset;
log.trace("layerIndex {} y is {}", layerIndex, y);
sugiyamaVertex.setPoint(Point.of(x, y));
previousVertexWidth = vertexWidth;
}
x = horizontalOffset;
y += verticalOffset;
layerIndex++;
}
}
public static void setPoints(
LV[][] layers,
Function vertexShapeFunction,
int horizontalOffset,
int verticalOffset,
Map, Point> vertexPointMap) {
int layerIndex = 0;
int x = horizontalOffset;
int y = verticalOffset;
layerIndex = 0;
for (LV[] layer : layers) {
for (LV sugiyamaVertex : layer) {
x += horizontalOffset;
log.trace("layerIndex {} y is {}", layerIndex, y);
sugiyamaVertex.setPoint(Point.of(x, y));
if (vertexPointMap.containsKey(sugiyamaVertex)) {
vertexPointMap.put(sugiyamaVertex, sugiyamaVertex.getPoint());
}
}
x = horizontalOffset;
y += verticalOffset;
layerIndex++;
}
for (int i = 0; i < layers.length; i++) {
for (int j = 0; j < layers[i].length; j++) {
LV sugiyamaVertex = layers[i][j];
vertexPointMap.put(sugiyamaVertex, sugiyamaVertex.getPoint());
}
}
}
public static void setPoints(
List>> layers,
Function vertexShapeFunction,
int horizontalOffset,
int verticalOffset,
Map, Point> vertexPointMap) {
Map rowWidthMap = new HashMap<>();
Map rowMaxHeightMap = new HashMap<>();
int layerIndex = 0;
for (List> layer : layers) {
int width = horizontalOffset;
int maxHeight = 0;
for (LV sugiyamaVertex : layer) {
if (!(sugiyamaVertex instanceof SyntheticLV)) {
Rectangle bounds = vertexShapeFunction.apply(sugiyamaVertex.getVertex());
width += bounds.width + horizontalOffset;
maxHeight = Math.max(maxHeight, (int) bounds.height);
} else {
width += horizontalOffset;
}
}
rowWidthMap.put(layerIndex, width);
rowMaxHeightMap.put(layerIndex, maxHeight);
layerIndex++;
}
int widestRowWidth = rowWidthMap.values().stream().mapToInt(v -> v).max().getAsInt();
int x = 0; //horizontalOffset;
int y = verticalOffset;
layerIndex = 0;
if (log.isTraceEnabled()) {
log.trace("layerMaxHeights {}", rowMaxHeightMap);
}
for (List> layer : layers) {
int previousVertexWidth = 0;
// offset against widest row
x += (widestRowWidth - rowWidthMap.get(layerIndex)) / 2;
y += rowMaxHeightMap.get(layerIndex) / 2;
if (layerIndex > 0) {
y += rowMaxHeightMap.get(layerIndex - 1) / 2;
}
for (LV sugiyamaVertex : layer) {
int vertexWidth = 0;
if (!(sugiyamaVertex instanceof SyntheticLV)) {
vertexWidth = (int) vertexShapeFunction.apply(sugiyamaVertex.getVertex()).width;
}
x += previousVertexWidth / 2 + vertexWidth / 2 + horizontalOffset;
log.trace("layerIndex {} y is {}", layerIndex, y);
sugiyamaVertex.setPoint(Point.of(x, y));
if (vertexPointMap.containsKey(sugiyamaVertex)) {
vertexPointMap.put(sugiyamaVertex, sugiyamaVertex.getPoint());
}
previousVertexWidth = vertexWidth;
}
x = horizontalOffset;
y += verticalOffset;
layerIndex++;
}
for (int i = 0; i < layers.size(); i++) {
for (int j = 0; j < layers.get(i).size(); j++) {
LV sugiyamaVertex = layers.get(i).get(j);
vertexPointMap.put(sugiyamaVertex, sugiyamaVertex.getPoint());
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy