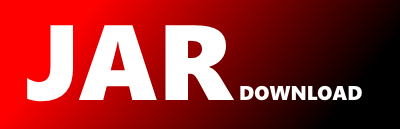
com.github.triceo.robozonky.app.management.Delinquency Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2017 The RoboZonky Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.triceo.robozonky.app.management;
import java.time.Duration;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
import java.util.Map;
import java.util.SortedMap;
import java.util.TreeMap;
import java.util.stream.Collectors;
import com.github.triceo.robozonky.api.notifications.Event;
import com.github.triceo.robozonky.api.notifications.LoanNoLongerDelinquentEvent;
import com.github.triceo.robozonky.api.notifications.LoanNowDelinquentEvent;
import com.github.triceo.robozonky.internal.api.Defaults;
class Delinquency implements DelinquencyMBean {
private final SortedMap delinquents = new TreeMap<>();
private OffsetDateTime lastInvestmentRunTimestamp;
@Override
public OffsetDateTime getLatestUpdatedDateTime() {
return this.lastInvestmentRunTimestamp;
}
void handle(final LoanNowDelinquentEvent event) {
delinquents.put(event.getLoan().getId(), event.getDelinquentSince());
handle((Event) event);
}
void handle(final LoanNoLongerDelinquentEvent event) {
delinquents.remove(event.getLoan().getId());
handle((Event) event);
}
private synchronized void handle(final Event event) {
this.lastInvestmentRunTimestamp = event.getCreatedOn();
}
@Override
public Map getAll() {
return getOlderThan(0);
}
private Map getOlderThan(final int thresholdInDays) {
final ZoneId zone = Defaults.ZONE_ID;
final ZonedDateTime now = LocalDate.now().atStartOfDay(zone);
final Duration expected = Duration.ofDays(thresholdInDays);
return delinquents.entrySet().stream()
.filter(e -> {
final ZonedDateTime since = e.getValue().atStartOfDay(zone);
final Duration actual = Duration.between(since, now);
return (actual.compareTo(expected) >= 0);
}).collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
}
@Override
public Map get10Plus() {
return getOlderThan(10);
}
@Override
public Map get30Plus() {
return getOlderThan(30);
}
@Override
public Map get60Plus() {
return getOlderThan(60);
}
@Override
public Map get90Plus() {
return getOlderThan(90);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy