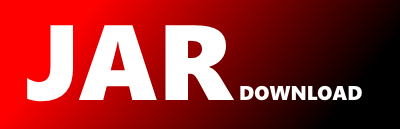
com.discobeard.spriter.dom.AnimationObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Spriter Show documentation
Show all versions of Spriter Show documentation
A generic Java library for Spriter animation files.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vhudson-jaxb-ri-2.1-2
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2013.01.18 at 06:33:53 PM MEZ
//
package com.discobeard.spriter.dom;
import java.math.BigDecimal;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlType;
import com.discobeard.spriter.dom.Entity.ObjectInfo;
/**
* Java class for AnimationObject complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="AnimationObject">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="folder" type="{http://www.w3.org/2001/XMLSchema}int" />
* <attribute name="file" type="{http://www.w3.org/2001/XMLSchema}int" />
* <attribute name="x" type="{http://www.w3.org/2001/XMLSchema}decimal" default="0" />
* <attribute name="y" type="{http://www.w3.org/2001/XMLSchema}decimal" default="0" />
* <attribute name="pivot_x" type="{http://www.w3.org/2001/XMLSchema}decimal" default="0" />
* <attribute name="pivot_y" type="{http://www.w3.org/2001/XMLSchema}decimal" default="1" />
* <attribute name="scale_x" type="{http://www.w3.org/2001/XMLSchema}decimal" default="1" />
* <attribute name="scale_y" type="{http://www.w3.org/2001/XMLSchema}decimal" default="1" />
* <attribute name="angle" type="{http://www.w3.org/2001/XMLSchema}decimal" default="0" />
* <attribute name="a" type="{http://www.w3.org/2001/XMLSchema}decimal" default="1" />
* <attribute name="z_index" type="{http://www.w3.org/2001/XMLSchema}int" default="0" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AnimationObject")
public class AnimationObject {
@XmlAttribute(name = "folder")
protected Integer folder;
@XmlAttribute(name = "file")
protected Integer file;
@XmlAttribute(name = "x")
protected BigDecimal x;
@XmlAttribute(name = "y")
protected BigDecimal y;
@XmlAttribute(name = "pivot_x")
protected BigDecimal pivotX;
@XmlAttribute(name = "pivot_y")
protected BigDecimal pivotY;
@XmlAttribute(name = "scale_x")
protected BigDecimal scaleX;
@XmlAttribute(name = "scale_y")
protected BigDecimal scaleY;
@XmlAttribute(name = "angle")
protected BigDecimal angle;
@XmlAttribute(name = "a")
protected BigDecimal a;
@XmlAttribute(name = "z_index")
protected Integer zIndex;
public ObjectInfo info;
/**
* Gets the value of the folder property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getFolder() {
return folder;
}
/**
* Sets the value of the folder property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setFolder(Integer value) {
this.folder = value;
}
/**
* Gets the value of the file property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getFile() {
return file;
}
/**
* Sets the value of the file property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setFile(Integer value) {
this.file = value;
}
/**
* Gets the value of the x property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getX() {
if (x == null) {
return new BigDecimal("0");
} else {
return x;
}
}
/**
* Sets the value of the x property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setX(BigDecimal value) {
this.x = value;
}
/**
* Gets the value of the y property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getY() {
if (y == null) {
return new BigDecimal("0");
} else {
return y;
}
}
/**
* Sets the value of the y property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setY(BigDecimal value) {
this.y = value;
}
/**
* Gets the value of the pivotX property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getPivotX() {
if (pivotX == null) {
return new BigDecimal("0");
} else {
return pivotX;
}
}
/**
* Sets the value of the pivotX property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setPivotX(BigDecimal value) {
this.pivotX = value;
}
/**
* Gets the value of the pivotY property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getPivotY() {
if (pivotY == null) {
return new BigDecimal("1");
} else {
return pivotY;
}
}
/**
* Sets the value of the pivotY property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setPivotY(BigDecimal value) {
this.pivotY = value;
}
/**
* Gets the value of the scaleX property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getScaleX() {
if (scaleX == null) {
return new BigDecimal("1");
} else {
return scaleX;
}
}
/**
* Sets the value of the scaleX property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setScaleX(BigDecimal value) {
this.scaleX = value;
}
/**
* Gets the value of the scaleY property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getScaleY() {
if (scaleY == null) {
return new BigDecimal("1");
} else {
return scaleY;
}
}
/**
* Sets the value of the scaleY property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setScaleY(BigDecimal value) {
this.scaleY = value;
}
/**
* Gets the value of the angle property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getAngle() {
if (angle == null) {
return new BigDecimal("0");
} else {
return angle;
}
}
/**
* Sets the value of the angle property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setAngle(BigDecimal value) {
this.angle = value;
}
/**
* Gets the value of the a property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getA() {
if (a == null) {
return new BigDecimal("1");
} else {
return a;
}
}
/**
* Sets the value of the a property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setA(BigDecimal value) {
this.a = value;
}
/**
* Gets the value of the zIndex property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public int getZIndex() {
if (zIndex == null) {
return 0;
} else {
return zIndex;
}
}
/**
* Sets the value of the zIndex property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setZIndex(Integer value) {
this.zIndex = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy