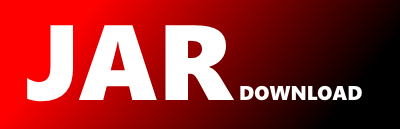
com.github.tsavo.apiomatic.controller.AbstractServiceDescriptionController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apiomatic Show documentation
Show all versions of apiomatic Show documentation
Round trip API tooling for Java with Spring and Hibernate
package com.github.tsavo.apiomatic.controller;
import java.util.ArrayList;
import java.util.List;
import java.util.Map.Entry;
import org.springframework.aop.framework.Advised;
import org.springframework.aop.support.AopUtils;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.stereotype.Controller;
import org.springframework.util.ClassUtils;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.OPTIONS;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
public class AbstractServiceDescriptionController implements ApplicationContextAware {
@Autowired
ApplicationContext context;
@Override
public void setApplicationContext(ApplicationContext aContext) throws BeansException {
context = aContext;
}
@RequestMapping(method = { RequestMethod.GET, RequestMethod.OPTIONS })
@Path("/")
@GET
@OPTIONS
public @ResponseBody
List getServiceList() throws Exception {
List results = new ArrayList<>();
for (Entry e : context.getBeansWithAnnotation(Path.class).entrySet()) {
results.add(new ServiceDescription(e.getKey(), ClassUtils.getUserClass(unwrapProxy(e.getValue()).getClass())));
}
return results;
}
@RequestMapping(value = "/{name}", method = { RequestMethod.GET, RequestMethod.OPTIONS })
@Path("/{name}")
@GET
@OPTIONS
public @ResponseBody
ControllerDescription getRestControllerForClass(@PathVariable("name") @PathParam("name") String aName) throws Exception {
return new ControllerDescription(ClassUtils.getUserClass(unwrapProxy(context.getBean(aName)).getClass()));
}
public static final Object unwrapProxy(Object bean) throws Exception {
if (bean instanceof Advised) {
Advised advised = (Advised) bean;
return unwrapProxy(advised.getTargetSource().getTarget());
}
return bean;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy