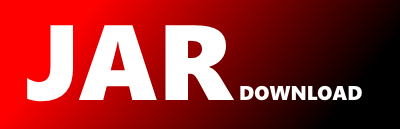
main.cesium.Entity.kt Maven / Gradle / Ivy
The newest version!
// Automatically generated - do not modify!
@file:JsModule("cesium")
@file:Suppress(
"NON_EXTERNAL_DECLARATION_IN_INAPPROPRIATE_FILE",
)
package cesium
/**
* Entity instances aggregate multiple forms of visualization into a single high-level object.
* They can be created manually and added to [Viewer.entities] or be produced by
* data sources, such as [CzmlDataSource] and [GeoJsonDataSource].
* @see Online Documentation
*/
external class Entity {
/**
* Gets or sets the entity collection that this entity belongs to.
* @see Online Documentation
*/
var entityCollection: EntityCollection
/**
* The availability, if any, associated with this object.
* If availability is undefined, it is assumed that this object's
* other properties will return valid data for any provided time.
* If availability exists, the objects other properties will only
* provide valid data if queried within the given interval.
* @see Online Documentation
*/
var availability: TimeIntervalCollection?
/**
* Gets the unique ID associated with this object.
* @see Online Documentation
*/
var id: String
/**
* Gets the event that is raised whenever a property or sub-property is changed or modified.
* @see Online Documentation
*/
val definitionChanged: Event
/**
* Gets or sets the name of the object. The name is intended for end-user
* consumption and does not need to be unique.
* @see Online Documentation
*/
var name: String?
/**
* Gets or sets whether this entity should be displayed. When set to true,
* the entity is only displayed if the parent entity's show property is also true.
* @see Online Documentation
*/
var show: Boolean
/**
* Gets whether this entity is being displayed, taking into account
* the visibility of any ancestor entities.
* @see Online Documentation
*/
var isShowing: Boolean
/**
* Gets or sets the parent object.
* @see Online Documentation
*/
var parent: Entity?
/**
* Gets the names of all properties registered on this instance.
* @see Online Documentation
*/
var propertyNames: Array
/**
* Gets or sets the billboard.
* @see Online Documentation
*/
var billboard: BillboardGraphics?
/**
* Gets or sets the box.
* @see Online Documentation
*/
var box: BoxGraphics?
/**
* Gets or sets the corridor.
* @see Online Documentation
*/
var corridor: CorridorGraphics?
/**
* Gets or sets the cylinder.
* @see Online Documentation
*/
var cylinder: CylinderGraphics?
/**
* Gets or sets the description.
* @see Online Documentation
*/
var description: Property?
/**
* Gets or sets the ellipse.
* @see Online Documentation
*/
var ellipse: EllipseGraphics?
/**
* Gets or sets the ellipsoid.
* @see Online Documentation
*/
var ellipsoid: EllipsoidGraphics?
/**
* Gets or sets the label.
* @see Online Documentation
*/
var label: LabelGraphics?
/**
* Gets or sets the model.
* @see Online Documentation
*/
var model: ModelGraphics?
/**
* Gets or sets the tileset.
* @see Online Documentation
*/
var tileset: Cesium3DTilesetGraphics?
/**
* Gets or sets the orientation.
* @see Online Documentation
*/
var orientation: Property?
/**
* Gets or sets the path.
* @see Online Documentation
*/
var path: PathGraphics?
/**
* Gets or sets the plane.
* @see Online Documentation
*/
var plane: PlaneGraphics?
/**
* Gets or sets the point graphic.
* @see Online Documentation
*/
var point: PointGraphics?
/**
* Gets or sets the polygon.
* @see Online Documentation
*/
var polygon: PolygonGraphics?
/**
* Gets or sets the polyline.
* @see Online Documentation
*/
var polyline: PolylineGraphics?
/**
* Gets or sets the polyline volume.
* @see Online Documentation
*/
var polylineVolume: PolylineVolumeGraphics?
/**
* Gets or sets the bag of arbitrary properties associated with this entity.
* @see Online Documentation
*/
var properties: PropertyBag?
/**
* Gets or sets the position.
* @see Online Documentation
*/
var position: PositionProperty?
/**
* Gets or sets the rectangle.
* @see Online Documentation
*/
var rectangle: RectangleGraphics?
/**
* Gets or sets the suggested initial offset when tracking this object.
* The offset is typically defined in the east-north-up reference frame,
* but may be another frame depending on the object's velocity.
* @see Online Documentation
*/
var viewFrom: Property?
/**
* Gets or sets the wall.
* @see Online Documentation
*/
var wall: WallGraphics?
/**
* Given a time, returns true if this object should have data during that time.
* @param [time] The time to check availability for.
* @return true if the object should have data during the provided time, false otherwise.
* @see Online Documentation
*/
fun isAvailable(time: JulianDate): Boolean
/**
* Adds a property to this object. Once a property is added, it can be
* observed with [Entity.definitionChanged] and composited
* with [CompositeEntityCollection]
* @param [propertyName] The name of the property to add.
* @see Online Documentation
*/
fun addProperty(propertyName: String)
/**
* Removed a property previously added with addProperty.
* @param [propertyName] The name of the property to remove.
* @see Online Documentation
*/
fun removeProperty(propertyName: String)
/**
* Assigns each unassigned property on this object to the value
* of the same property on the provided source object.
* @param [source] The object to be merged into this object.
* @see Online Documentation
*/
fun merge(source: Entity)
/**
* Computes the model matrix for the entity's transform at specified time. Returns undefined if orientation or position
* are undefined.
* @param [time] The time to retrieve model matrix for.
* @param [result] The object onto which to store the result.
* @return The modified result parameter or a new Matrix4 instance if one was not provided. Result is undefined if position or orientation are undefined.
* @see Online Documentation
*/
fun computeModelMatrix(
time: JulianDate,
result: Matrix4? = definedExternally,
): Matrix4
companion object {
/**
* Checks if the given Scene supports materials besides Color on Entities draped on terrain or 3D Tiles.
* If this feature is not supported, Entities with non-color materials but no `height` will
* instead be rendered as if height is 0.
* @param [scene] The current scene.
* @return Whether or not the current scene supports materials for entities on terrain.
* @see Online Documentation
*/
fun supportsMaterialsforEntitiesOnTerrain(scene: Scene): Boolean
/**
* Checks if the given Scene supports polylines clamped to terrain or 3D Tiles.
* If this feature is not supported, Entities with PolylineGraphics will be rendered with vertices at
* the provided heights and using the `arcType` parameter instead of clamped to the ground.
* @param [scene] The current scene.
* @return Whether or not the current scene supports polylines on terrain or 3D TIles.
* @see Online Documentation
*/
fun supportsPolylinesOnTerrain(scene: Scene): Boolean
}
}
inline fun Entity(
block: Entity.() -> Unit,
): Entity =
Entity().apply(block)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy