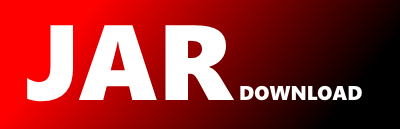
main.cesium.VertexFormat.kt Maven / Gradle / Ivy
The newest version!
// Automatically generated - do not modify!
@file:JsModule("cesium")
package cesium
/**
* A vertex format defines what attributes make up a vertex. A VertexFormat can be provided
* to a [Geometry] to request that certain properties be computed, e.g., just position,
* position and normal, etc.
* ```
* // Create a vertex format with position and 2D texture coordinate attributes.
* const format = new VertexFormat({
* position : true,
* st : true
* });
* ```
* @see Online Documentation
*
* @constructor
* @param [options] An object with boolean properties corresponding to VertexFormat properties as shown in the code example.
* @see Online Documentation
*/
external class VertexFormat(options: Any? = definedExternally) {
/**
* When `true`, the vertex has a 3D position attribute.
*
* 64-bit floating-point (for precision). 3 components per attribute.
* @see Online Documentation
*/
var position: Boolean
/**
* When `true`, the vertex has a normal attribute (normalized), which is commonly used for lighting.
*
* 32-bit floating-point. 3 components per attribute.
* @see Online Documentation
*/
var normal: Boolean
/**
* When `true`, the vertex has a 2D texture coordinate attribute.
*
* 32-bit floating-point. 2 components per attribute
* @see Online Documentation
*/
var st: Boolean
/**
* When `true`, the vertex has a bitangent attribute (normalized), which is used for tangent-space effects like bump mapping.
*
* 32-bit floating-point. 3 components per attribute.
* @see Online Documentation
*/
var bitangent: Boolean
/**
* When `true`, the vertex has a tangent attribute (normalized), which is used for tangent-space effects like bump mapping.
*
* 32-bit floating-point. 3 components per attribute.
* @see Online Documentation
*/
var tangent: Boolean
/**
* When `true`, the vertex has an RGB color attribute.
*
* 8-bit unsigned byte. 3 components per attribute.
* @see Online Documentation
*/
var color: Boolean
companion object : Packable {
/**
* An immutable vertex format with only a position attribute.
* @see Online Documentation
*/
val POSITION_ONLY: VertexFormat
/**
* An immutable vertex format with position and normal attributes.
* This is compatible with per-instance color appearances like [PerInstanceColorAppearance].
* @see Online Documentation
*/
val POSITION_AND_NORMAL: VertexFormat
/**
* An immutable vertex format with position, normal, and st attributes.
* This is compatible with [MaterialAppearance] when [MaterialAppearance.materialSupport]
* is TEXTURED/code>.
* @see Online Documentation
*/
val POSITION_NORMAL_AND_ST: VertexFormat
/**
* An immutable vertex format with position and st attributes.
* This is compatible with [EllipsoidSurfaceAppearance].
* @see Online Documentation
*/
val POSITION_AND_ST: VertexFormat
/**
* An immutable vertex format with position and color attributes.
* @see Online Documentation
*/
val POSITION_AND_COLOR: VertexFormat
/**
* An immutable vertex format with well-known attributes: position, normal, st, tangent, and bitangent.
* @see Online Documentation
*/
val ALL: VertexFormat
/**
* An immutable vertex format with position, normal, and st attributes.
* This is compatible with most appearances and materials; however
* normal and st attributes are not always required. When this is
* known in advance, another `VertexFormat` should be used.
* @see Online Documentation
*/
val DEFAULT: VertexFormat
/**
* The number of elements used to pack the object into an array.
* @see Online Documentation
*/
override val packedLength: Int
/**
* Stores the provided instance into the provided array.
* @param [value] The value to pack.
* @param [array] The array to pack into.
* @param [startingIndex] The index into the array at which to start packing the elements.
* Default value - `0`
* @return The array that was packed into
* @see Online Documentation
*/
override fun pack(
value: VertexFormat,
array: Array,
startingIndex: Int?,
): Array
/**
* Retrieves an instance from a packed array.
* @param [array] The packed array.
* @param [startingIndex] The starting index of the element to be unpacked.
* Default value - `0`
* @param [result] The object into which to store the result.
* @return The modified result parameter or a new VertexFormat instance if one was not provided.
* @see Online Documentation
*/
override fun unpack(
array: Array,
startingIndex: Int?,
result: VertexFormat?,
): VertexFormat
/**
* Duplicates a VertexFormat instance.
* @param [vertexFormat] The vertex format to duplicate.
* @param [result] The object onto which to store the result.
* @return The modified result parameter or a new VertexFormat instance if one was not provided. (Returns undefined if vertexFormat is undefined)
* @see Online Documentation
*/
fun clone(
vertexFormat: VertexFormat,
result: VertexFormat? = definedExternally,
): VertexFormat
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy