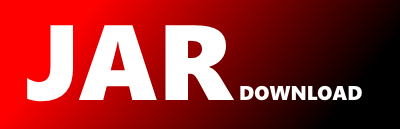
com.github.twitch4j.graphql.internal.CreatePollMutation Maven / Gradle / Ivy
The newest version!
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package com.github.twitch4j.graphql.internal;
import com.apollographql.apollo.api.Mutation;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.InputFieldMarshaller;
import com.apollographql.apollo.api.internal.InputFieldWriter;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.UnmodifiableMapBuilder;
import com.apollographql.apollo.api.internal.Utils;
import com.github.twitch4j.graphql.internal.type.CreatePollErrorCode;
import com.github.twitch4j.graphql.internal.type.CreatePollInput;
import com.github.twitch4j.graphql.internal.type.CustomType;
import com.github.twitch4j.graphql.internal.type.PollStatus;
import java.io.IOException;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class CreatePollMutation implements Mutation {
public static final String OPERATION_ID = "e7d2e264cdbfd68492bcb390f3150c3b61fe728c6cf98a95aa31f26db23f2709";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"mutation createPoll($input: CreatePollInput!) {\n"
+ " createPoll(input: $input) {\n"
+ " __typename\n"
+ " error {\n"
+ " __typename\n"
+ " code\n"
+ " }\n"
+ " poll {\n"
+ " __typename\n"
+ " id\n"
+ " choices {\n"
+ " __typename\n"
+ " id\n"
+ " title\n"
+ " }\n"
+ " startedAt\n"
+ " status\n"
+ " title\n"
+ " remainingDurationMilliseconds\n"
+ " }\n"
+ " }\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "createPoll";
}
};
private final CreatePollMutation.Variables variables;
public CreatePollMutation(@NotNull CreatePollInput input) {
Utils.checkNotNull(input, "input == null");
variables = new CreatePollMutation.Variables(input);
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public CreatePollMutation.Data wrapData(CreatePollMutation.Data data) {
return data;
}
@Override
public CreatePollMutation.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source) throws
IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString) throws
IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
private @NotNull CreatePollInput input;
Builder() {
}
public Builder input(@NotNull CreatePollInput input) {
this.input = input;
return this;
}
public CreatePollMutation build() {
Utils.checkNotNull(input, "input == null");
return new CreatePollMutation(input);
}
}
public static final class Variables extends Operation.Variables {
private final @NotNull CreatePollInput input;
private final transient Map valueMap = new LinkedHashMap<>();
Variables(@NotNull CreatePollInput input) {
this.input = input;
this.valueMap.put("input", input);
}
public @NotNull CreatePollInput input() {
return input;
}
@Override
public Map valueMap() {
return Collections.unmodifiableMap(valueMap);
}
@Override
public InputFieldMarshaller marshaller() {
return new InputFieldMarshaller() {
@Override
public void marshal(InputFieldWriter writer) throws IOException {
writer.writeObject("input", input.marshaller());
}
};
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forObject("createPoll", "createPoll", new UnmodifiableMapBuilder(1)
.put("input", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "input")
.build())
.build(), true, Collections.emptyList())
};
final @Nullable CreatePoll createPoll;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@Nullable CreatePoll createPoll) {
this.createPoll = createPoll;
}
/**
* createPoll creates a poll.
*/
public @Nullable CreatePoll createPoll() {
return this.createPoll;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeObject($responseFields[0], createPoll != null ? createPoll.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "createPoll=" + createPoll
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return ((this.createPoll == null) ? (that.createPoll == null) : this.createPoll.equals(that.createPoll));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= (createPoll == null) ? 0 : createPoll.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final CreatePoll.Mapper createPollFieldMapper = new CreatePoll.Mapper();
@Override
public Data map(ResponseReader reader) {
final CreatePoll createPoll = reader.readObject($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public CreatePoll read(ResponseReader reader) {
return createPollFieldMapper.map(reader);
}
});
return new Data(createPoll);
}
}
}
/**
* Outputs from the create poll mutation.
*/
public static class CreatePoll {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forObject("error", "error", null, true, Collections.emptyList()),
ResponseField.forObject("poll", "poll", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable Error error;
final @Nullable Poll poll;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public CreatePoll(@NotNull String __typename, @Nullable Error error, @Nullable Poll poll) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.error = error;
this.poll = poll;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* If present, there was an error with the request.
*/
public @Nullable Error error() {
return this.error;
}
/**
* The created poll.
*/
public @Nullable Poll poll() {
return this.poll;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeObject($responseFields[1], error != null ? error.marshaller() : null);
writer.writeObject($responseFields[2], poll != null ? poll.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "CreatePoll{"
+ "__typename=" + __typename + ", "
+ "error=" + error + ", "
+ "poll=" + poll
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof CreatePoll) {
CreatePoll that = (CreatePoll) o;
return this.__typename.equals(that.__typename)
&& ((this.error == null) ? (that.error == null) : this.error.equals(that.error))
&& ((this.poll == null) ? (that.poll == null) : this.poll.equals(that.poll));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (error == null) ? 0 : error.hashCode();
h *= 1000003;
h ^= (poll == null) ? 0 : poll.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Error.Mapper errorFieldMapper = new Error.Mapper();
final Poll.Mapper pollFieldMapper = new Poll.Mapper();
@Override
public CreatePoll map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Error error = reader.readObject($responseFields[1], new ResponseReader.ObjectReader() {
@Override
public Error read(ResponseReader reader) {
return errorFieldMapper.map(reader);
}
});
final Poll poll = reader.readObject($responseFields[2], new ResponseReader.ObjectReader() {
@Override
public Poll read(ResponseReader reader) {
return pollFieldMapper.map(reader);
}
});
return new CreatePoll(__typename, error, poll);
}
}
}
/**
* Vote in poll error.
*/
public static class Error {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forString("code", "code", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull CreatePollErrorCode code;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Error(@NotNull String __typename, @NotNull CreatePollErrorCode code) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.code = Utils.checkNotNull(code, "code == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* Code describing the error.
*/
public @NotNull CreatePollErrorCode code() {
return this.code;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeString($responseFields[1], code.rawValue());
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Error{"
+ "__typename=" + __typename + ", "
+ "code=" + code
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Error) {
Error that = (Error) o;
return this.__typename.equals(that.__typename)
&& this.code.equals(that.code);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= code.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public Error map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String codeStr = reader.readString($responseFields[1]);
final CreatePollErrorCode code;
if (codeStr != null) {
code = CreatePollErrorCode.safeValueOf(codeStr);
} else {
code = null;
}
return new Error(__typename, code);
}
}
}
/**
* A poll users can vote in.
*/
public static class Poll {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("id", "id", null, false, CustomType.ID, Collections.emptyList()),
ResponseField.forList("choices", "choices", null, false, Collections.emptyList()),
ResponseField.forCustomType("startedAt", "startedAt", null, false, CustomType.TIME, Collections.emptyList()),
ResponseField.forString("status", "status", null, false, Collections.emptyList()),
ResponseField.forString("title", "title", null, false, Collections.emptyList()),
ResponseField.forInt("remainingDurationMilliseconds", "remainingDurationMilliseconds", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull String id;
final @NotNull List choices;
final @NotNull Object startedAt;
final @NotNull PollStatus status;
final @NotNull String title;
final int remainingDurationMilliseconds;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Poll(@NotNull String __typename, @NotNull String id, @NotNull List choices,
@NotNull Object startedAt, @NotNull PollStatus status, @NotNull String title,
int remainingDurationMilliseconds) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.id = Utils.checkNotNull(id, "id == null");
this.choices = Utils.checkNotNull(choices, "choices == null");
this.startedAt = Utils.checkNotNull(startedAt, "startedAt == null");
this.status = Utils.checkNotNull(status, "status == null");
this.title = Utils.checkNotNull(title, "title == null");
this.remainingDurationMilliseconds = remainingDurationMilliseconds;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* ID of poll.
*/
public @NotNull String id() {
return this.id;
}
/**
* A list of choices users can vote for.
*/
public @NotNull List choices() {
return this.choices;
}
/**
* Time when poll started.
*/
public @NotNull Object startedAt() {
return this.startedAt;
}
/**
* The status of the poll.
*/
public @NotNull PollStatus status() {
return this.status;
}
/**
* Title of poll.
*/
public @NotNull String title() {
return this.title;
}
/**
* Amount of milliseconds before the poll ends.
* 0 when the polls is ended.
*/
public int remainingDurationMilliseconds() {
return this.remainingDurationMilliseconds;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], id);
writer.writeList($responseFields[2], choices, new ResponseWriter.ListWriter() {
@Override
public void write(List items, ResponseWriter.ListItemWriter listItemWriter) {
for (Object item : items) {
listItemWriter.writeObject(((Choice) item).marshaller());
}
}
});
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[3], startedAt);
writer.writeString($responseFields[4], status.rawValue());
writer.writeString($responseFields[5], title);
writer.writeInt($responseFields[6], remainingDurationMilliseconds);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Poll{"
+ "__typename=" + __typename + ", "
+ "id=" + id + ", "
+ "choices=" + choices + ", "
+ "startedAt=" + startedAt + ", "
+ "status=" + status + ", "
+ "title=" + title + ", "
+ "remainingDurationMilliseconds=" + remainingDurationMilliseconds
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Poll) {
Poll that = (Poll) o;
return this.__typename.equals(that.__typename)
&& this.id.equals(that.id)
&& this.choices.equals(that.choices)
&& this.startedAt.equals(that.startedAt)
&& this.status.equals(that.status)
&& this.title.equals(that.title)
&& this.remainingDurationMilliseconds == that.remainingDurationMilliseconds;
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= id.hashCode();
h *= 1000003;
h ^= choices.hashCode();
h *= 1000003;
h ^= startedAt.hashCode();
h *= 1000003;
h ^= status.hashCode();
h *= 1000003;
h ^= title.hashCode();
h *= 1000003;
h ^= remainingDurationMilliseconds;
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Choice.Mapper choiceFieldMapper = new Choice.Mapper();
@Override
public Poll map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String id = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
final List choices = reader.readList($responseFields[2], new ResponseReader.ListReader() {
@Override
public Choice read(ResponseReader.ListItemReader listItemReader) {
return listItemReader.readObject(new ResponseReader.ObjectReader() {
@Override
public Choice read(ResponseReader reader) {
return choiceFieldMapper.map(reader);
}
});
}
});
final Object startedAt = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[3]);
final String statusStr = reader.readString($responseFields[4]);
final PollStatus status;
if (statusStr != null) {
status = PollStatus.safeValueOf(statusStr);
} else {
status = null;
}
final String title = reader.readString($responseFields[5]);
final int remainingDurationMilliseconds = reader.readInt($responseFields[6]);
return new Poll(__typename, id, choices, startedAt, status, title, remainingDurationMilliseconds);
}
}
}
/**
* A choice in a poll that users can vote for.
*/
public static class Choice {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("id", "id", null, false, CustomType.ID, Collections.emptyList()),
ResponseField.forString("title", "title", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull String id;
final @NotNull String title;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Choice(@NotNull String __typename, @NotNull String id, @NotNull String title) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.id = Utils.checkNotNull(id, "id == null");
this.title = Utils.checkNotNull(title, "title == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* ID of choice.
*/
public @NotNull String id() {
return this.id;
}
/**
* The title of the choice.
*/
public @NotNull String title() {
return this.title;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], id);
writer.writeString($responseFields[2], title);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Choice{"
+ "__typename=" + __typename + ", "
+ "id=" + id + ", "
+ "title=" + title
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Choice) {
Choice that = (Choice) o;
return this.__typename.equals(that.__typename)
&& this.id.equals(that.id)
&& this.title.equals(that.title);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= id.hashCode();
h *= 1000003;
h ^= title.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public Choice map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String id = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
final String title = reader.readString($responseFields[2]);
return new Choice(__typename, id, title);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy