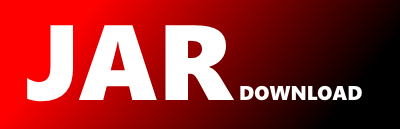
com.github.twitch4j.graphql.internal.FetchPredictionQuery Maven / Gradle / Ivy
The newest version!
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package com.github.twitch4j.graphql.internal;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Query;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.InputFieldMarshaller;
import com.apollographql.apollo.api.internal.InputFieldWriter;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.UnmodifiableMapBuilder;
import com.apollographql.apollo.api.internal.Utils;
import com.github.twitch4j.graphql.internal.type.CustomType;
import com.github.twitch4j.graphql.internal.type.PredictionEventStatus;
import com.github.twitch4j.graphql.internal.type.PredictionOutcomeColor;
import java.io.IOException;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class FetchPredictionQuery implements Query {
public static final String OPERATION_ID = "9716871bdc7625c8664e05fb77368e00580678d05bc01a4454c800cee0205420";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"query fetchPrediction($id: ID!) {\n"
+ " predictionEvent(id: $id) {\n"
+ " __typename\n"
+ " channel {\n"
+ " __typename\n"
+ " id\n"
+ " }\n"
+ " createdAt\n"
+ " endedAt\n"
+ " id\n"
+ " lockedAt\n"
+ " outcomes {\n"
+ " __typename\n"
+ " color\n"
+ " id\n"
+ " title\n"
+ " topPredictors {\n"
+ " __typename\n"
+ " id\n"
+ " points\n"
+ " predictedAt\n"
+ " updatedAt\n"
+ " user {\n"
+ " __typename\n"
+ " id\n"
+ " displayName\n"
+ " login\n"
+ " }\n"
+ " }\n"
+ " totalPoints\n"
+ " totalUsers\n"
+ " }\n"
+ " predictionWindowSeconds\n"
+ " status\n"
+ " title\n"
+ " winningOutcome {\n"
+ " __typename\n"
+ " id\n"
+ " }\n"
+ " }\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "fetchPrediction";
}
};
private final FetchPredictionQuery.Variables variables;
public FetchPredictionQuery(@NotNull String id) {
Utils.checkNotNull(id, "id == null");
variables = new FetchPredictionQuery.Variables(id);
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public FetchPredictionQuery.Data wrapData(FetchPredictionQuery.Data data) {
return data;
}
@Override
public FetchPredictionQuery.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source) throws
IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString) throws
IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
private @NotNull String id;
Builder() {
}
public Builder id(@NotNull String id) {
this.id = id;
return this;
}
public FetchPredictionQuery build() {
Utils.checkNotNull(id, "id == null");
return new FetchPredictionQuery(id);
}
}
public static final class Variables extends Operation.Variables {
private final @NotNull String id;
private final transient Map valueMap = new LinkedHashMap<>();
Variables(@NotNull String id) {
this.id = id;
this.valueMap.put("id", id);
}
public @NotNull String id() {
return id;
}
@Override
public Map valueMap() {
return Collections.unmodifiableMap(valueMap);
}
@Override
public InputFieldMarshaller marshaller() {
return new InputFieldMarshaller() {
@Override
public void marshal(InputFieldWriter writer) throws IOException {
writer.writeCustom("id", com.github.twitch4j.graphql.internal.type.CustomType.ID, id);
}
};
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forObject("predictionEvent", "predictionEvent", new UnmodifiableMapBuilder(1)
.put("id", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "id")
.build())
.build(), true, Collections.emptyList())
};
final @Nullable PredictionEvent predictionEvent;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@Nullable PredictionEvent predictionEvent) {
this.predictionEvent = predictionEvent;
}
/**
* Returns a Prediction Event by its ID.
*/
public @Nullable PredictionEvent predictionEvent() {
return this.predictionEvent;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeObject($responseFields[0], predictionEvent != null ? predictionEvent.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "predictionEvent=" + predictionEvent
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return ((this.predictionEvent == null) ? (that.predictionEvent == null) : this.predictionEvent.equals(that.predictionEvent));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= (predictionEvent == null) ? 0 : predictionEvent.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final PredictionEvent.Mapper predictionEventFieldMapper = new PredictionEvent.Mapper();
@Override
public Data map(ResponseReader reader) {
final PredictionEvent predictionEvent = reader.readObject($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public PredictionEvent read(ResponseReader reader) {
return predictionEventFieldMapper.map(reader);
}
});
return new Data(predictionEvent);
}
}
}
/**
* An Event that users can make Predictions on.
*/
public static class PredictionEvent {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forObject("channel", "channel", null, true, Collections.emptyList()),
ResponseField.forCustomType("createdAt", "createdAt", null, false, CustomType.TIME, Collections.emptyList()),
ResponseField.forCustomType("endedAt", "endedAt", null, true, CustomType.TIME, Collections.emptyList()),
ResponseField.forCustomType("id", "id", null, false, CustomType.ID, Collections.emptyList()),
ResponseField.forCustomType("lockedAt", "lockedAt", null, true, CustomType.TIME, Collections.emptyList()),
ResponseField.forList("outcomes", "outcomes", null, false, Collections.emptyList()),
ResponseField.forInt("predictionWindowSeconds", "predictionWindowSeconds", null, false, Collections.emptyList()),
ResponseField.forString("status", "status", null, false, Collections.emptyList()),
ResponseField.forString("title", "title", null, false, Collections.emptyList()),
ResponseField.forObject("winningOutcome", "winningOutcome", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable Channel channel;
final @NotNull Object createdAt;
final @Nullable Object endedAt;
final @NotNull String id;
final @Nullable Object lockedAt;
final @NotNull List outcomes;
final int predictionWindowSeconds;
final @NotNull PredictionEventStatus status;
final @NotNull String title;
final @Nullable WinningOutcome winningOutcome;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public PredictionEvent(@NotNull String __typename, @Nullable Channel channel,
@NotNull Object createdAt, @Nullable Object endedAt, @NotNull String id,
@Nullable Object lockedAt, @NotNull List outcomes, int predictionWindowSeconds,
@NotNull PredictionEventStatus status, @NotNull String title,
@Nullable WinningOutcome winningOutcome) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.channel = channel;
this.createdAt = Utils.checkNotNull(createdAt, "createdAt == null");
this.endedAt = endedAt;
this.id = Utils.checkNotNull(id, "id == null");
this.lockedAt = lockedAt;
this.outcomes = Utils.checkNotNull(outcomes, "outcomes == null");
this.predictionWindowSeconds = predictionWindowSeconds;
this.status = Utils.checkNotNull(status, "status == null");
this.title = Utils.checkNotNull(title, "title == null");
this.winningOutcome = winningOutcome;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* The channel that the Prediction Event belongs to. Null if this is Event is not tied to a channel.
*/
public @Nullable Channel channel() {
return this.channel;
}
/**
* The timestamp of when the Event was created.
*/
public @NotNull Object createdAt() {
return this.createdAt;
}
/**
* The timestamp of when the Event was resolved or canceled. Null if the Event is not resolved or canceled yet.
*/
public @Nullable Object endedAt() {
return this.endedAt;
}
/**
* Unique identifier of the Prediction Event.
*/
public @NotNull String id() {
return this.id;
}
/**
* The timestamp of when the Event was locked. Null if the Event is still active.
*/
public @Nullable Object lockedAt() {
return this.lockedAt;
}
/**
* The Outcomes available for predicting in this Event.
*/
public @NotNull List outcomes() {
return this.outcomes;
}
/**
* The length of the prediction window (the duration that the Event accepts predictions) in seconds.
*/
public int predictionWindowSeconds() {
return this.predictionWindowSeconds;
}
/**
* The current status of the Event.
*/
public @NotNull PredictionEventStatus status() {
return this.status;
}
/**
* The title of the Event.
*/
public @NotNull String title() {
return this.title;
}
/**
* The Outcome that ended up being selected as the "correct" Outcome. Null if the Event has not been resolved yet.
* This Outcome will also be present as one of the outcomes in the "outcomes" field.
*/
public @Nullable WinningOutcome winningOutcome() {
return this.winningOutcome;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeObject($responseFields[1], channel != null ? channel.marshaller() : null);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[2], createdAt);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[3], endedAt);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[4], id);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[5], lockedAt);
writer.writeList($responseFields[6], outcomes, new ResponseWriter.ListWriter() {
@Override
public void write(List items, ResponseWriter.ListItemWriter listItemWriter) {
for (Object item : items) {
listItemWriter.writeObject(((Outcome) item).marshaller());
}
}
});
writer.writeInt($responseFields[7], predictionWindowSeconds);
writer.writeString($responseFields[8], status.rawValue());
writer.writeString($responseFields[9], title);
writer.writeObject($responseFields[10], winningOutcome != null ? winningOutcome.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "PredictionEvent{"
+ "__typename=" + __typename + ", "
+ "channel=" + channel + ", "
+ "createdAt=" + createdAt + ", "
+ "endedAt=" + endedAt + ", "
+ "id=" + id + ", "
+ "lockedAt=" + lockedAt + ", "
+ "outcomes=" + outcomes + ", "
+ "predictionWindowSeconds=" + predictionWindowSeconds + ", "
+ "status=" + status + ", "
+ "title=" + title + ", "
+ "winningOutcome=" + winningOutcome
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof PredictionEvent) {
PredictionEvent that = (PredictionEvent) o;
return this.__typename.equals(that.__typename)
&& ((this.channel == null) ? (that.channel == null) : this.channel.equals(that.channel))
&& this.createdAt.equals(that.createdAt)
&& ((this.endedAt == null) ? (that.endedAt == null) : this.endedAt.equals(that.endedAt))
&& this.id.equals(that.id)
&& ((this.lockedAt == null) ? (that.lockedAt == null) : this.lockedAt.equals(that.lockedAt))
&& this.outcomes.equals(that.outcomes)
&& this.predictionWindowSeconds == that.predictionWindowSeconds
&& this.status.equals(that.status)
&& this.title.equals(that.title)
&& ((this.winningOutcome == null) ? (that.winningOutcome == null) : this.winningOutcome.equals(that.winningOutcome));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (channel == null) ? 0 : channel.hashCode();
h *= 1000003;
h ^= createdAt.hashCode();
h *= 1000003;
h ^= (endedAt == null) ? 0 : endedAt.hashCode();
h *= 1000003;
h ^= id.hashCode();
h *= 1000003;
h ^= (lockedAt == null) ? 0 : lockedAt.hashCode();
h *= 1000003;
h ^= outcomes.hashCode();
h *= 1000003;
h ^= predictionWindowSeconds;
h *= 1000003;
h ^= status.hashCode();
h *= 1000003;
h ^= title.hashCode();
h *= 1000003;
h ^= (winningOutcome == null) ? 0 : winningOutcome.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Channel.Mapper channelFieldMapper = new Channel.Mapper();
final Outcome.Mapper outcomeFieldMapper = new Outcome.Mapper();
final WinningOutcome.Mapper winningOutcomeFieldMapper = new WinningOutcome.Mapper();
@Override
public PredictionEvent map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Channel channel = reader.readObject($responseFields[1], new ResponseReader.ObjectReader() {
@Override
public Channel read(ResponseReader reader) {
return channelFieldMapper.map(reader);
}
});
final Object createdAt = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[2]);
final Object endedAt = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[3]);
final String id = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[4]);
final Object lockedAt = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[5]);
final List outcomes = reader.readList($responseFields[6], new ResponseReader.ListReader() {
@Override
public Outcome read(ResponseReader.ListItemReader listItemReader) {
return listItemReader.readObject(new ResponseReader.ObjectReader() {
@Override
public Outcome read(ResponseReader reader) {
return outcomeFieldMapper.map(reader);
}
});
}
});
final int predictionWindowSeconds = reader.readInt($responseFields[7]);
final String statusStr = reader.readString($responseFields[8]);
final PredictionEventStatus status;
if (statusStr != null) {
status = PredictionEventStatus.safeValueOf(statusStr);
} else {
status = null;
}
final String title = reader.readString($responseFields[9]);
final WinningOutcome winningOutcome = reader.readObject($responseFields[10], new ResponseReader.ObjectReader() {
@Override
public WinningOutcome read(ResponseReader reader) {
return winningOutcomeFieldMapper.map(reader);
}
});
return new PredictionEvent(__typename, channel, createdAt, endedAt, id, lockedAt, outcomes, predictionWindowSeconds, status, title, winningOutcome);
}
}
}
/**
* A User's place on Twitch.
*/
public static class Channel {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("id", "id", null, false, CustomType.ID, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull String id;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Channel(@NotNull String __typename, @NotNull String id) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.id = Utils.checkNotNull(id, "id == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* The channel's unique identfier.
*/
public @NotNull String id() {
return this.id;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], id);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Channel{"
+ "__typename=" + __typename + ", "
+ "id=" + id
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Channel) {
Channel that = (Channel) o;
return this.__typename.equals(that.__typename)
&& this.id.equals(that.id);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= id.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public Channel map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String id = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
return new Channel(__typename, id);
}
}
}
/**
* A single Outcome that users can choose in a Prediction Event.
*/
public static class Outcome {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forString("color", "color", null, false, Collections.emptyList()),
ResponseField.forCustomType("id", "id", null, false, CustomType.ID, Collections.emptyList()),
ResponseField.forString("title", "title", null, false, Collections.emptyList()),
ResponseField.forList("topPredictors", "topPredictors", null, false, Collections.emptyList()),
ResponseField.forInt("totalPoints", "totalPoints", null, false, Collections.emptyList()),
ResponseField.forInt("totalUsers", "totalUsers", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull PredictionOutcomeColor color;
final @NotNull String id;
final @NotNull String title;
final @NotNull List topPredictors;
final int totalPoints;
final int totalUsers;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Outcome(@NotNull String __typename, @NotNull PredictionOutcomeColor color,
@NotNull String id, @NotNull String title, @NotNull List topPredictors,
int totalPoints, int totalUsers) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.color = Utils.checkNotNull(color, "color == null");
this.id = Utils.checkNotNull(id, "id == null");
this.title = Utils.checkNotNull(title, "title == null");
this.topPredictors = Utils.checkNotNull(topPredictors, "topPredictors == null");
this.totalPoints = totalPoints;
this.totalUsers = totalUsers;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* The color of this Outcome.
*/
public @NotNull PredictionOutcomeColor color() {
return this.color;
}
/**
* The unique identifier of the Prediction Outcome.
*/
public @NotNull String id() {
return this.id;
}
/**
* The title of the Event.
*/
public @NotNull String title() {
return this.title;
}
/**
* The top predictors (sorted by most points spent) of this Outcome. Empty if no users have predicted this Outcome.
*/
public @NotNull List topPredictors() {
return this.topPredictors;
}
/**
* The total number of points that have been spent predicting this Outcome.
*/
public int totalPoints() {
return this.totalPoints;
}
/**
* The total number of users that have predicted this Outcome.
*/
public int totalUsers() {
return this.totalUsers;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeString($responseFields[1], color.rawValue());
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[2], id);
writer.writeString($responseFields[3], title);
writer.writeList($responseFields[4], topPredictors, new ResponseWriter.ListWriter() {
@Override
public void write(List items, ResponseWriter.ListItemWriter listItemWriter) {
for (Object item : items) {
listItemWriter.writeObject(((TopPredictor) item).marshaller());
}
}
});
writer.writeInt($responseFields[5], totalPoints);
writer.writeInt($responseFields[6], totalUsers);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Outcome{"
+ "__typename=" + __typename + ", "
+ "color=" + color + ", "
+ "id=" + id + ", "
+ "title=" + title + ", "
+ "topPredictors=" + topPredictors + ", "
+ "totalPoints=" + totalPoints + ", "
+ "totalUsers=" + totalUsers
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Outcome) {
Outcome that = (Outcome) o;
return this.__typename.equals(that.__typename)
&& this.color.equals(that.color)
&& this.id.equals(that.id)
&& this.title.equals(that.title)
&& this.topPredictors.equals(that.topPredictors)
&& this.totalPoints == that.totalPoints
&& this.totalUsers == that.totalUsers;
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= color.hashCode();
h *= 1000003;
h ^= id.hashCode();
h *= 1000003;
h ^= title.hashCode();
h *= 1000003;
h ^= topPredictors.hashCode();
h *= 1000003;
h ^= totalPoints;
h *= 1000003;
h ^= totalUsers;
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final TopPredictor.Mapper topPredictorFieldMapper = new TopPredictor.Mapper();
@Override
public Outcome map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String colorStr = reader.readString($responseFields[1]);
final PredictionOutcomeColor color;
if (colorStr != null) {
color = PredictionOutcomeColor.safeValueOf(colorStr);
} else {
color = null;
}
final String id = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[2]);
final String title = reader.readString($responseFields[3]);
final List topPredictors = reader.readList($responseFields[4], new ResponseReader.ListReader() {
@Override
public TopPredictor read(ResponseReader.ListItemReader listItemReader) {
return listItemReader.readObject(new ResponseReader.ObjectReader() {
@Override
public TopPredictor read(ResponseReader reader) {
return topPredictorFieldMapper.map(reader);
}
});
}
});
final int totalPoints = reader.readInt($responseFields[5]);
final int totalUsers = reader.readInt($responseFields[6]);
return new Outcome(__typename, color, id, title, topPredictors, totalPoints, totalUsers);
}
}
}
/**
* A single Prediction made by a user on a Prediction Event.
*/
public static class TopPredictor {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("id", "id", null, false, CustomType.ID, Collections.emptyList()),
ResponseField.forInt("points", "points", null, false, Collections.emptyList()),
ResponseField.forCustomType("predictedAt", "predictedAt", null, false, CustomType.TIME, Collections.emptyList()),
ResponseField.forCustomType("updatedAt", "updatedAt", null, false, CustomType.TIME, Collections.emptyList()),
ResponseField.forObject("user", "user", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull String id;
final int points;
final @NotNull Object predictedAt;
final @NotNull Object updatedAt;
final @Nullable User user;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public TopPredictor(@NotNull String __typename, @NotNull String id, int points,
@NotNull Object predictedAt, @NotNull Object updatedAt, @Nullable User user) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.id = Utils.checkNotNull(id, "id == null");
this.points = points;
this.predictedAt = Utils.checkNotNull(predictedAt, "predictedAt == null");
this.updatedAt = Utils.checkNotNull(updatedAt, "updatedAt == null");
this.user = user;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* The unique identifier of the Prediction.
*/
public @NotNull String id() {
return this.id;
}
/**
* The number of points that the user spent on this Prediction.
*/
public int points() {
return this.points;
}
/**
* The timestamp of when the user initially made this Prediction.
*/
public @NotNull Object predictedAt() {
return this.predictedAt;
}
/**
* The timestamp of when the user most recently updated this Prediction.
*/
public @NotNull Object updatedAt() {
return this.updatedAt;
}
/**
* The user that made this Prediction.
*/
public @Nullable User user() {
return this.user;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], id);
writer.writeInt($responseFields[2], points);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[3], predictedAt);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[4], updatedAt);
writer.writeObject($responseFields[5], user != null ? user.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "TopPredictor{"
+ "__typename=" + __typename + ", "
+ "id=" + id + ", "
+ "points=" + points + ", "
+ "predictedAt=" + predictedAt + ", "
+ "updatedAt=" + updatedAt + ", "
+ "user=" + user
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TopPredictor) {
TopPredictor that = (TopPredictor) o;
return this.__typename.equals(that.__typename)
&& this.id.equals(that.id)
&& this.points == that.points
&& this.predictedAt.equals(that.predictedAt)
&& this.updatedAt.equals(that.updatedAt)
&& ((this.user == null) ? (that.user == null) : this.user.equals(that.user));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= id.hashCode();
h *= 1000003;
h ^= points;
h *= 1000003;
h ^= predictedAt.hashCode();
h *= 1000003;
h ^= updatedAt.hashCode();
h *= 1000003;
h ^= (user == null) ? 0 : user.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final User.Mapper userFieldMapper = new User.Mapper();
@Override
public TopPredictor map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String id = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
final int points = reader.readInt($responseFields[2]);
final Object predictedAt = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[3]);
final Object updatedAt = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[4]);
final User user = reader.readObject($responseFields[5], new ResponseReader.ObjectReader() {
@Override
public User read(ResponseReader reader) {
return userFieldMapper.map(reader);
}
});
return new TopPredictor(__typename, id, points, predictedAt, updatedAt, user);
}
}
}
/**
* Twitch user.
*/
public static class User {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("id", "id", null, false, CustomType.ID, Collections.emptyList()),
ResponseField.forString("displayName", "displayName", null, false, Collections.emptyList()),
ResponseField.forString("login", "login", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull String id;
final @NotNull String displayName;
final @NotNull String login;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public User(@NotNull String __typename, @NotNull String id, @NotNull String displayName,
@NotNull String login) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.id = Utils.checkNotNull(id, "id == null");
this.displayName = Utils.checkNotNull(displayName, "displayName == null");
this.login = Utils.checkNotNull(login, "login == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* The user's unique identifier.
*/
public @NotNull String id() {
return this.id;
}
/**
* A user-styled version of their login.
* For international users, this could be the user's login with localized characters.
*/
public @NotNull String displayName() {
return this.displayName;
}
/**
* The user's standard alphanumeric Twitch name.
*/
public @NotNull String login() {
return this.login;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], id);
writer.writeString($responseFields[2], displayName);
writer.writeString($responseFields[3], login);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "User{"
+ "__typename=" + __typename + ", "
+ "id=" + id + ", "
+ "displayName=" + displayName + ", "
+ "login=" + login
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof User) {
User that = (User) o;
return this.__typename.equals(that.__typename)
&& this.id.equals(that.id)
&& this.displayName.equals(that.displayName)
&& this.login.equals(that.login);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= id.hashCode();
h *= 1000003;
h ^= displayName.hashCode();
h *= 1000003;
h ^= login.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public User map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String id = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
final String displayName = reader.readString($responseFields[2]);
final String login = reader.readString($responseFields[3]);
return new User(__typename, id, displayName, login);
}
}
}
/**
* A single Outcome that users can choose in a Prediction Event.
*/
public static class WinningOutcome {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("id", "id", null, false, CustomType.ID, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull String id;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public WinningOutcome(@NotNull String __typename, @NotNull String id) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.id = Utils.checkNotNull(id, "id == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* The unique identifier of the Prediction Outcome.
*/
public @NotNull String id() {
return this.id;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], id);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "WinningOutcome{"
+ "__typename=" + __typename + ", "
+ "id=" + id
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof WinningOutcome) {
WinningOutcome that = (WinningOutcome) o;
return this.__typename.equals(that.__typename)
&& this.id.equals(that.id);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= id.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public WinningOutcome map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String id = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
return new WinningOutcome(__typename, id);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy