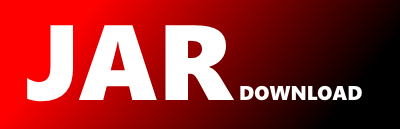
com.github.twitch4j.graphql.internal.UpdateClipMutation Maven / Gradle / Ivy
The newest version!
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package com.github.twitch4j.graphql.internal;
import com.apollographql.apollo.api.Mutation;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.InputFieldMarshaller;
import com.apollographql.apollo.api.internal.InputFieldWriter;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.UnmodifiableMapBuilder;
import com.apollographql.apollo.api.internal.Utils;
import com.github.twitch4j.graphql.internal.type.CustomType;
import com.github.twitch4j.graphql.internal.type.UpdateClipInput;
import java.io.IOException;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class UpdateClipMutation implements Mutation {
public static final String OPERATION_ID = "d7639d3210d2b766f2d0211376a087ebd725f1a07e5d6b9745052bfbdeb3b4eb";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"mutation updateClip($updateClipInput: UpdateClipInput!) {\n"
+ " updateClip(input: $updateClipInput) {\n"
+ " __typename\n"
+ " clip {\n"
+ " __typename\n"
+ " id\n"
+ " title\n"
+ " }\n"
+ " error {\n"
+ " __typename\n"
+ " message\n"
+ " }\n"
+ " }\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "updateClip";
}
};
private final UpdateClipMutation.Variables variables;
public UpdateClipMutation(@NotNull UpdateClipInput updateClipInput) {
Utils.checkNotNull(updateClipInput, "updateClipInput == null");
variables = new UpdateClipMutation.Variables(updateClipInput);
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public UpdateClipMutation.Data wrapData(UpdateClipMutation.Data data) {
return data;
}
@Override
public UpdateClipMutation.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source) throws
IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString) throws
IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
private @NotNull UpdateClipInput updateClipInput;
Builder() {
}
public Builder updateClipInput(@NotNull UpdateClipInput updateClipInput) {
this.updateClipInput = updateClipInput;
return this;
}
public UpdateClipMutation build() {
Utils.checkNotNull(updateClipInput, "updateClipInput == null");
return new UpdateClipMutation(updateClipInput);
}
}
public static final class Variables extends Operation.Variables {
private final @NotNull UpdateClipInput updateClipInput;
private final transient Map valueMap = new LinkedHashMap<>();
Variables(@NotNull UpdateClipInput updateClipInput) {
this.updateClipInput = updateClipInput;
this.valueMap.put("updateClipInput", updateClipInput);
}
public @NotNull UpdateClipInput updateClipInput() {
return updateClipInput;
}
@Override
public Map valueMap() {
return Collections.unmodifiableMap(valueMap);
}
@Override
public InputFieldMarshaller marshaller() {
return new InputFieldMarshaller() {
@Override
public void marshal(InputFieldWriter writer) throws IOException {
writer.writeObject("updateClipInput", updateClipInput.marshaller());
}
};
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forObject("updateClip", "updateClip", new UnmodifiableMapBuilder(1)
.put("input", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "updateClipInput")
.build())
.build(), true, Collections.emptyList())
};
final @Nullable UpdateClip updateClip;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@Nullable UpdateClip updateClip) {
this.updateClip = updateClip;
}
/**
* updateClip allows a user to update the metadata of a clip.
*/
public @Nullable UpdateClip updateClip() {
return this.updateClip;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeObject($responseFields[0], updateClip != null ? updateClip.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "updateClip=" + updateClip
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return ((this.updateClip == null) ? (that.updateClip == null) : this.updateClip.equals(that.updateClip));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= (updateClip == null) ? 0 : updateClip.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final UpdateClip.Mapper updateClipFieldMapper = new UpdateClip.Mapper();
@Override
public Data map(ResponseReader reader) {
final UpdateClip updateClip = reader.readObject($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public UpdateClip read(ResponseReader reader) {
return updateClipFieldMapper.map(reader);
}
});
return new Data(updateClip);
}
}
}
/**
* UpdateClipPayload returns the updated clip.
*/
public static class UpdateClip {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forObject("clip", "clip", null, false, Collections.emptyList()),
ResponseField.forObject("error", "error", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull Clip clip;
final @Nullable Error error;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public UpdateClip(@NotNull String __typename, @NotNull Clip clip, @Nullable Error error) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.clip = Utils.checkNotNull(clip, "clip == null");
this.error = error;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* The clip with its updated properties.
*/
public @NotNull Clip clip() {
return this.clip;
}
/**
* The error when the clip fails to update a clip.
*/
public @Nullable Error error() {
return this.error;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeObject($responseFields[1], clip.marshaller());
writer.writeObject($responseFields[2], error != null ? error.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "UpdateClip{"
+ "__typename=" + __typename + ", "
+ "clip=" + clip + ", "
+ "error=" + error
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof UpdateClip) {
UpdateClip that = (UpdateClip) o;
return this.__typename.equals(that.__typename)
&& this.clip.equals(that.clip)
&& ((this.error == null) ? (that.error == null) : this.error.equals(that.error));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= clip.hashCode();
h *= 1000003;
h ^= (error == null) ? 0 : error.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Clip.Mapper clipFieldMapper = new Clip.Mapper();
final Error.Mapper errorFieldMapper = new Error.Mapper();
@Override
public UpdateClip map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Clip clip = reader.readObject($responseFields[1], new ResponseReader.ObjectReader() {
@Override
public Clip read(ResponseReader reader) {
return clipFieldMapper.map(reader);
}
});
final Error error = reader.readObject($responseFields[2], new ResponseReader.ObjectReader() {
@Override
public Error read(ResponseReader reader) {
return errorFieldMapper.map(reader);
}
});
return new UpdateClip(__typename, clip, error);
}
}
}
/**
* A recorded, replayable part of a live broadcast.
*/
public static class Clip {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("id", "id", null, false, CustomType.ID, Collections.emptyList()),
ResponseField.forString("title", "title", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull String id;
final @NotNull String title;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Clip(@NotNull String __typename, @NotNull String id, @NotNull String title) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.id = Utils.checkNotNull(id, "id == null");
this.title = Utils.checkNotNull(title, "title == null");
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* The clip's unique identifier.
*/
public @NotNull String id() {
return this.id;
}
/**
* The title of the clip.
*/
public @NotNull String title() {
return this.title;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], id);
writer.writeString($responseFields[2], title);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Clip{"
+ "__typename=" + __typename + ", "
+ "id=" + id + ", "
+ "title=" + title
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Clip) {
Clip that = (Clip) o;
return this.__typename.equals(that.__typename)
&& this.id.equals(that.id)
&& this.title.equals(that.title);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= id.hashCode();
h *= 1000003;
h ^= title.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public Clip map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String id = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
final String title = reader.readString($responseFields[2]);
return new Clip(__typename, id, title);
}
}
}
/**
* UpdateClipError is an error associated with the updateClip mutation.
*/
public static class Error {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forString("message", "message", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable String message;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Error(@NotNull String __typename, @Nullable String message) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.message = message;
}
public @NotNull String __typename() {
return this.__typename;
}
/**
* The error when the clip fails to update a clip.
*/
public @Nullable String message() {
return this.message;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeString($responseFields[1], message);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Error{"
+ "__typename=" + __typename + ", "
+ "message=" + message
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Error) {
Error that = (Error) o;
return this.__typename.equals(that.__typename)
&& ((this.message == null) ? (that.message == null) : this.message.equals(that.message));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (message == null) ? 0 : message.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public Error map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String message = reader.readString($responseFields[1]);
return new Error(__typename, message);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy