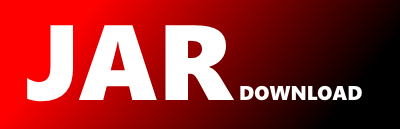
com.github.twitch4j.pubsub.handlers.WhispersHandler Maven / Gradle / Ivy
The newest version!
package com.github.twitch4j.pubsub.handlers;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.JsonNode;
import com.github.twitch4j.common.enums.CommandPermission;
import com.github.twitch4j.common.events.TwitchEvent;
import com.github.twitch4j.common.events.domain.EventUser;
import com.github.twitch4j.common.events.user.PrivateMessageEvent;
import com.github.twitch4j.common.util.TwitchUtils;
import com.github.twitch4j.common.util.TypeConvert;
import com.github.twitch4j.pubsub.domain.WhisperThread;
import com.github.twitch4j.pubsub.events.WhisperThreadUpdateEvent;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
class WhispersHandler implements TopicHandler {
@Override
public String topicName() {
return "whispers";
}
@Override
public TwitchEvent apply(Args args) {
// Whisper data is escaped Json cast into a String
JsonNode msgDataParsed = TypeConvert.jsonToObject(args.getData().asText(), JsonNode.class);
String type = args.getType();
if ("whisper_sent".equals(type) || "whisper_received".equals(type)) {
//TypeReference allows type parameters (unlike Class) and avoids needing @SuppressWarnings("unchecked")
Map tags = TypeConvert.convertValue(msgDataParsed.path("tags"), new TypeReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy