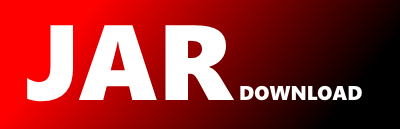
com.unbound.provider.ECDSASignature Maven / Gradle / Ivy
package com.unbound.provider;
import com.unbound.common.crypto.EC;
import com.unbound.provider.kmip.KMIP;
import java.io.IOException;
import java.security.*;
import java.security.interfaces.ECPublicKey;
import java.util.Arrays;
public class ECDSASignature extends SignatureSpi
{
private Signature pubSignature = null;
private HashType hash;
private MessageDigest md = null;
private byte[] buffer = new byte[66]; // max curve size
private int bufferOffset = 0;
private UBECPrivateKey prvKey = null;
ECDSASignature(HashType hash)
{
this.hash = hash;
}
// ------------------------------ interface ----------------------
@Override
protected void engineInitVerify(PublicKey publicKey) throws InvalidKeyException
{
if (!(publicKey instanceof ECPublicKey)) throw new InvalidKeyException("Invalid key type");
try
{
String hashName = hash==null ? "NONE" : hash.name;
pubSignature = Signature.getInstance(hashName+"withECDSA", "SunEC");
}
catch (Exception e) { throw new InvalidKeyException("engineInitVerify failed"); }
pubSignature.initVerify(publicKey);
}
@Override
protected void engineInitSign(PrivateKey privateKey) throws InvalidKeyException
{
if (!(privateKey instanceof UBECPrivateKey)) throw new InvalidKeyException("Invalid key type");
prvKey = (UBECPrivateKey)privateKey;
bufferOffset = 0;
md = hash==null ? null : hash.getMessageDigest();
}
@Override
protected void engineUpdate(byte b) throws SignatureException
{
if (pubSignature!=null)
{
pubSignature.update(b);
return;
}
byte[] in = {b};
engineUpdate(in, 0, 1);
}
@Override
protected void engineUpdate(byte[] in, int inOffset, int inLen) throws SignatureException
{
if (pubSignature!=null)
{
pubSignature.update(in, inOffset, inLen);
return;
}
if (md==null)
{
int size = prvKey.size();
if (bufferOffset+inLen > size) inLen = size - bufferOffset;
System.arraycopy(in, inOffset, buffer, bufferOffset, inLen);
bufferOffset += inLen;
}
else md.update(in, inOffset, inLen);
}
@Override
protected byte[] engineSign() throws SignatureException
{
EC.Curve curve = prvKey.getCurve();
byte[] in;
if (md==null)
{
in = Arrays.copyOfRange(buffer, 0, bufferOffset);
}
else
{
in = md.digest();
if (in.length>curve.size) in = Arrays.copyOfRange(in,0, curve.size);
}
int kmipAlg = hash==null ? 0 : hash.kmipEcdsaSignatureCode;
try
{
byte[] bin = prvKey.sign(in, KMIP.CryptographicAlgorithm.EC, kmipAlg);
return curve.sigBinToDer(bin);
}
catch (IOException e) { throw new ProviderException(e); }
}
@Override
protected boolean engineVerify(byte[] sigBytes) throws SignatureException
{
return pubSignature.verify(sigBytes);
}
@Override
protected void engineSetParameter(String param, Object value) throws InvalidParameterException
{
throw new UnsupportedOperationException("setParameter() not supported");
}
@Override
protected Object engineGetParameter(String param) throws InvalidParameterException
{
throw new UnsupportedOperationException("getParameter() not supported");
}
public static final class Raw extends ECDSASignature { public Raw() { super(null); } }
public static final class SHA1 extends ECDSASignature { public SHA1() { super(HashType.SHA1); } }
public static final class SHA256 extends ECDSASignature { public SHA256() { super(HashType.SHA256); } }
public static final class SHA384 extends ECDSASignature { public SHA384() { super(HashType.SHA384); } }
public static final class SHA512 extends ECDSASignature { public SHA512() { super(HashType.SHA512); } }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy