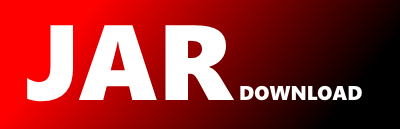
com.unbound.provider.RSASignaturePSS Maven / Gradle / Ivy
package com.unbound.provider;
import com.unbound.common.crypto.SystemProvider;
import java.io.IOException;
import java.security.*;
import java.security.interfaces.RSAPublicKey;
import java.security.spec.AlgorithmParameterSpec;
import java.security.spec.MGF1ParameterSpec;
import java.security.spec.PSSParameterSpec;
public class RSASignaturePSS extends SignatureSpi
{
private Signature pubSignature = null;
private UBRSAPrivateKey prvKey = null;
private MessageDigest md = null;
private PSSParameterSpec pss = null;
private int saltLen = 20;
private HashType hash = HashType.SHA1;
private HashType mgfHash = HashType.SHA1;
// ------------------------- interface ---------------------------------
@Override
protected void engineInitVerify(PublicKey publicKey) throws InvalidKeyException
{
if (!(publicKey instanceof RSAPublicKey)) throw new InvalidKeyException("Invalid key type");
try
{
pubSignature = SystemProvider.Signature.getInstance("RSASSA-PSS");
if (pss==null) pss = new PSSParameterSpec("SHA-1", "MGF1", null, 20, 1);
pubSignature.setParameter(pss);
}
catch (Throwable e) { throw new InvalidKeyException("engineInitVerify failed"); }
pubSignature.initVerify(publicKey);
}
@Override
protected void engineInitSign(PrivateKey privateKey) throws InvalidKeyException
{
if (!(privateKey instanceof UBRSAPrivateKey)) throw new InvalidKeyException("Invalid key type");
prvKey = (UBRSAPrivateKey)privateKey;
md = hash.getMessageDigest();
}
@Override
protected void engineUpdate(byte b) throws SignatureException
{
if (pubSignature!=null)
{
pubSignature.update(b);
return;
}
byte[] in = {b};
engineUpdate(in, 0, 1);
}
@Override
protected void engineUpdate(byte[] in, int inOffset, int inLen) throws SignatureException
{
if (pubSignature!=null)
{
pubSignature.update(in, inOffset, inLen);
return;
}
md.update(in, inOffset, inLen);
}
@Override
protected byte[] engineSign() throws SignatureException
{
byte[] in = md.digest();
try
{
return prvKey.signPss(in, hash.kmipCode, mgfHash.kmipCode, saltLen);
}
catch (IOException e) { throw new ProviderException(e); }
}
@Override
protected boolean engineVerify(byte[] sigBytes) throws SignatureException
{
return pubSignature.verify(sigBytes);
}
@Override
protected void engineSetParameter(String param, Object value) throws InvalidParameterException
{
throw new UnsupportedOperationException("setParameter() not supported");
}
@Override
protected void engineSetParameter(AlgorithmParameterSpec params) throws InvalidAlgorithmParameterException
{
if (params == null) throw new InvalidAlgorithmParameterException("Parameters cannot be null");
if (!(params instanceof PSSParameterSpec)) throw new InvalidAlgorithmParameterException("parameters must be type PSSParameterSpec");
PSSParameterSpec pss = (PSSParameterSpec)params;
if (!(pss.getMGFAlgorithm().equalsIgnoreCase("MGF1"))) throw new InvalidAlgorithmParameterException("Only supports MGF1");
if (pss.getTrailerField() != 1) throw new InvalidAlgorithmParameterException("Only supports TrailerFieldBC(1)");
saltLen = pss.getSaltLength();
mgfHash = hash = HashType.getFromName(pss.getDigestAlgorithm());
AlgorithmParameterSpec mgfParams = pss.getMGFParameters();
if (mgfParams!=null) mgfHash = HashType.getFromName(((MGF1ParameterSpec)mgfParams).getDigestAlgorithm());
this.pss = pss;
}
@Override
protected Object engineGetParameter(String param) throws InvalidParameterException
{
throw new UnsupportedOperationException("getParameter() not supported");
}
@Override
protected AlgorithmParameters engineGetParameters()
{
if (pss == null) throw new ProviderException("Missing required PSS parameters");
try
{
AlgorithmParameters ap = AlgorithmParameters.getInstance("RSASSA-PSS");
ap.init(pss);
return ap;
}
catch (GeneralSecurityException e) { throw new ProviderException(e); }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy