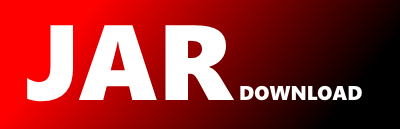
com.unbound.provider.Server Maven / Gradle / Ivy
package com.unbound.provider;
import com.unbound.common.Log;
import com.unbound.provider.kmip.response.ResponseMessage;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.security.*;
import java.time.Clock;
import java.util.*;
class Server
{
private static final int CHECK_DISCONNECTED_TIMEOUT = 3000; // milliseconds
private static final Clock clock = Clock.systemUTC();
private static Server[] servers = null;
private static Random randomPicker = new Random();
URL url;
private String name;
private long checkDisconnectedClock = 0;
//HashMap> connections = new HashMap<>();
private Server(String name) throws MalformedURLException
{
Log log = Log.func("Server").log("Name", name).end(); try
{
this.name = name;
if (name.startsWith("https://"))
{
name = name.substring(8);
}
URL host = new URL("https://" + name);
int port = host.getPort();
if (port<=0) port = 443;
url = new URL("https", host.getHost(), port, "/kmip", null);
}
catch (Exception e) { log.failed(e); throw e; } finally { log.leave(); }
}
static synchronized void initialize(String servers) throws MalformedURLException
{
Log log = Log.func("Servers.initialize").log("servers", servers).end(); try
{
initialize(servers.split(",")); // ep1,ep2,ep3
}
catch (Exception e) { log.failed(e); throw e; } finally { log.leave(); }
}
static synchronized void initialize(String[] serverNames) throws MalformedURLException
{
if (serverNames.length==0) throw new IllegalArgumentException("Server list is empty");
Server[] temp = new Server[serverNames.length];
int index = 0;
for (String name: serverNames)
{
temp[index++] = new Server(name);
}
servers = temp;
}
/*private synchronized Connection getConnection(Partition partition)
{
Queue queue = connections.get(partition.name);
if (queue==null) return null;
return queue.poll();
}
private synchronized void releaseConnection(Connection connection)
{
String partitionName = connection.partition.name;
Queue queue = connections.get(partitionName);
if (queue==null)
{
queue = new ArrayDeque<>();
connections.put(partitionName, queue);
}
queue.add(connection);
}*/
static synchronized Server[] getList()
{
long now = clock.millis();
int count = 0;
for (Server server : servers)
{
if (now < server.checkDisconnectedClock + CHECK_DISCONNECTED_TIMEOUT) continue;
count++;
}
if (count==0)
{
Server server = servers[randomPicker.nextInt(servers.length)];
Server[] list = new Server[1];
list[0] = server;
return list;
}
Server[] list = new Server[count];
count = 0;
for (Server server : servers)
{
if (now < server.checkDisconnectedClock + CHECK_DISCONNECTED_TIMEOUT) continue;
list[count++] = server;
}
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy