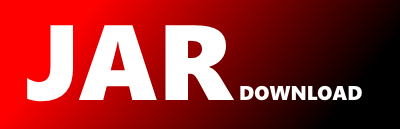
com.unbound.provider.UBECPrivateKey Maven / Gradle / Ivy
package com.unbound.provider;
import com.dyadicsec.provider.KeyParameters;
import com.unbound.common.Converter;
import com.unbound.common.Log;
import com.unbound.common.crypto.EC;
import com.unbound.common.crypto.SHA256;
import com.unbound.common.crypto.SystemProvider;
import com.unbound.provider.kmip.KMIP;
import com.unbound.provider.kmip.attribute.*;
import com.unbound.provider.kmip.object.ManagedObject;
import com.unbound.provider.kmip.object.PrivateKey;
import com.unbound.provider.kmip.request.DeriveRequest;
import com.unbound.provider.kmip.request.SignRequest;
import com.unbound.provider.kmip.request.dy.DyDeriveRequest;
import com.unbound.provider.kmip.response.DeriveResponse;
import com.unbound.provider.kmip.response.GetAttributesResponse;
import com.unbound.provider.kmip.response.GetResponse;
import com.unbound.provider.kmip.response.SignResponse;
import com.unbound.provider.kmip.response.dy.DyDeriveResponse;
import java.io.IOException;
import java.math.BigInteger;
import java.security.KeyFactory;
import java.security.PublicKey;
import java.security.interfaces.ECPrivateKey;
import java.security.interfaces.ECPublicKey;
import java.security.spec.*;
public final class UBECPrivateKey extends UBPrivateKey implements java.security.interfaces.ECPrivateKey
{
UBECPrivateKey(Partition partition)
{
super(partition);
}
@Override
int getKmipAlg()
{
return KMIP.CryptographicAlgorithm.EC;
}
@Override
int getDefaultUsageMask() { return KMIP.CryptographicUsageMask.Sign; }
UBECPrivateKey(Partition partition, long uid, GetAttributesResponse getAttrResp) throws InvalidKeySpecException
{
super(partition, uid, getAttrResp);
}
@Override
PublicKey convertResponseToPublicKey(GetResponse get) throws InvalidKeySpecException
{
PrivateKey kmipManagedObject = (PrivateKey)get.object;
X509EncodedKeySpec spec = new X509EncodedKeySpec(kmipManagedObject.keyBlock.buf);
KeyFactory kf = SystemProvider.KeyFactory.getInstance("EC");
return kf.generatePublic(spec);
}
public ECPublicKey ecPublicKey()
{
return (ECPublicKey)pub;
}
EC.Curve getCurve()
{
return EC.getCurve(ecPublicKey());
}
int size()
{
return getCurve().size;
}
@Override
TemplateAttribute prepareGenerateTemplate(KeyParameters keyParameter, int bitSize, EC.Curve curve)
{
int usage = getDefaultUsageMask();
TemplateAttribute template = new TemplateAttribute();
if (keyParameter!=null)
{
usage = updateKmipKeyUsageMask(keyParameter, usage, false);
updateAttrs(keyParameter, template.attrs);
}
template.attrs.add(new EnumAttribute(KMIP.Tag.CryptographicAlgorithm, KMIP.CryptographicAlgorithm.EC));
template.attrs.add(new EnumAttribute(KMIP.Tag.RecommendedCurve, curve.kmipCode));
template.attrs.add(new IntAttribute(KMIP.Tag.CryptographicUsageMask, usage));
return template;
}
private ManagedObject prepareManagedObjectRaw(ECPrivateKeySpec keySpec) throws InvalidKeySpecException
{
EC.Curve curve = EC.getCurve(keySpec.getParams());
if (curve==null) throw new InvalidKeySpecException("Curve not supported");
PrivateKey mo = new PrivateKey();
mo.keyBlock.formatType = KMIP.KeyFormatType.TransparentECPrivateKey;
mo.keyBlock.D = keySpec.getS();
mo.keyBlock.curveType = curve.kmipCode;
mo.keyBlock.algorithm = getKmipAlg();
return mo;
}
private ManagedObject prepareManagedObjectPkcs8(PKCS8EncodedKeySpec keySpec)
{
PrivateKey mo = new PrivateKey();
mo.keyBlock.formatType = KMIP.KeyFormatType.PKCS_8;
mo.keyBlock.buf = keySpec.getEncoded();
mo.keyBlock.algorithm = getKmipAlg();
return mo;
}
@Override
ManagedObject prepareManagedObject(KeySpec keySpec) throws InvalidKeySpecException
{
if (keySpec instanceof ECPrivateKeySpec) return prepareManagedObjectRaw((ECPrivateKeySpec)keySpec);
if (keySpec instanceof PKCS8EncodedKeySpec) return prepareManagedObjectPkcs8((PKCS8EncodedKeySpec)keySpec);
return null;
}
@Override
ManagedObject prepareManagedObject(java.security.PrivateKey privateKey) throws InvalidKeySpecException
{
if (!(privateKey instanceof ECPrivateKey)) return null;
ECPrivateKey key = (ECPrivateKey)privateKey;
EC.Curve curve = EC.getCurve(key.getParams());
if (curve==null) throw new InvalidKeySpecException("Curve not supported");
PrivateKey mo = new PrivateKey();
mo.keyBlock.formatType = KMIP.KeyFormatType.TransparentECPrivateKey;
mo.keyBlock.D = key.getS();
mo.keyBlock.curveType = curve.kmipCode;
return mo;
}
static long getKeyUid(ECPublicKey pub)
{
EC.Curve curve = EC.getCurve(pub);
byte[] hash = SHA256.hash(curve.toDer(pub.getW()));
return Converter.getBE8(hash, 0);
}
byte[] ecdh(ECPoint otherPub) throws IOException
{
Log log = Log.func("UBECPrivateKey.ecdh").end(); try
{
DyDeriveRequest req = new DyDeriveRequest();
req.uid = uidToStr(uid);
req.data = getCurve().toDer(otherPub);
req.params = new CryptoParams();
req.params.cryptoAlg = KMIP.CryptographicAlgorithm.ECDH;
DyDeriveResponse resp = (DyDeriveResponse) partition.transmit(req);
return resp.data;
}
catch (Exception e) { log.failed(e); throw e; } finally { log.leave(); }
}
// ------------------------- interface -------------------
@Override
public String getAlgorithm() { return "EC"; }
@Override
public String getFormat() { return "N/A"; }
@Override
public byte[] getEncoded()
{
return null;
}
@Override
public BigInteger getS()
{
return null;
}
@Override
public ECParameterSpec getParams()
{
return ecPublicKey().getParams();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy