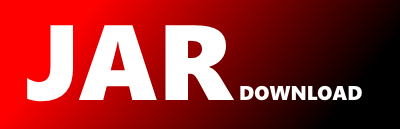
com.dyadicsec.cryptoki.CKR_Exception Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.dyadicsec.cryptoki;
import java.util.HashMap;
public class CKR_Exception extends java.lang.Exception
{
public int errorCode;
public String functionName;
@Override
public String getMessage()
{
String e;
if (functionName == null) e = "Library errorCode=0x" + Integer.toHexString(errorCode);
else e = "Library." + functionName + " failed, errorCode=0x" + Integer.toHexString(errorCode);
String t = textErrors.get(errorCode);
if (t==null) return e;
return e + ": " + t;
}
CKR_Exception(int errorCode, java.lang.Exception src)
{
super(src);
this.errorCode = errorCode;
}
CKR_Exception(int errorCode)
{
this.errorCode = errorCode;
}
CKR_Exception(int errorCode, String functionName)
{
this.errorCode = errorCode;
}
static void check(long rv) throws CKR_Exception
{
int errorCode = (int) (rv >> 32);
if (errorCode != 0) throw new CKR_Exception(errorCode);
}
static void check(long rv, String function) throws CKR_Exception
{
int errorCode = (int) (rv >> 32);
if (errorCode != 0) throw new CKR_Exception(errorCode, function);
}
static final HashMap textErrors;
static
{
textErrors = new HashMap<>();
textErrors.put(CK.CKR_HOST_MEMORY, "Computer has insufficient memory to perform the requested action");
textErrors.put(CK.CKR_DEVICE_ERROR, "UKC error");
textErrors.put(CK.CKR_DEVICE_MEMORY, "UKC has insufficient memory to perform the requested action");
textErrors.put(CK.CKR_DEVICE_REMOVED, "UKC was not connected during the operation");
textErrors.put(CK.CKR_PIN_INCORRECT, "Password is incorrect");
textErrors.put(CK.CKR_PIN_INVALID, "Password must comply with the quality settings");
textErrors.put(CK.CKR_PIN_LEN_RANGE, "Password is too short");
textErrors.put(CK.CKR_PIN_EXPIRED, "Password has expired. Please change password");
textErrors.put(CK.CKR_SESSION_CLOSED, "UKC was not connected during the operation");
textErrors.put(CK.CKR_SESSION_HANDLE_INVALID, "UKC was not connected during the operation");
textErrors.put(CK.CKR_TOKEN_NOT_PRESENT, "UKC connection failed during the operation");
textErrors.put(CK.CKR_TEMPLATE_INCONSISTENT, "Settings are inconsistent");
textErrors.put(CK.CKR_ARGUMENTS_BAD, "Function has inappropriate arguments");
textErrors.put(CK.CKR_FUNCTION_FAILED, "Operation failed");
textErrors.put(CK.CKR_FUNCTION_CANCELED, "Function cancelled");
textErrors.put(CK.CKR_KEY_SIZE_RANGE, "UKC does not support this key size");
textErrors.put(CK.CKR_MECHANISM_INVALID, "Cryptographic mechanism is invalid");
textErrors.put(CK.CKR_MECHANISM_PARAM_INVALID, "Cryptographic mechanism parameter is invalid");
textErrors.put(CK.CKR_KEY_TYPE_INCONSISTENT, "Cryptographic key type is inconsistent with mechanism");
textErrors.put(CK.CKR_ATTRIBUTE_SENSITIVE, "Key is sensitive and cannot be revealed");
textErrors.put(CK.CKR_ENCRYPTED_DATA_INVALID, "Encrypted data is invalid");
textErrors.put(CK.CKR_ENCRYPTED_DATA_LEN_RANGE, "Encrypted data length is out of range");
textErrors.put(CK.CKR_SIGNATURE_INVALID, "Signature is invalid");
textErrors.put(CK.CKR_SIGNATURE_LEN_RANGE, "Signature length is out of range");
textErrors.put(CK.CKR_SLOT_ID_INVALID, "Unknown partition");
textErrors.put(CK.CKR_UNWRAPPING_KEY_SIZE_RANGE, "Unwrapping key size is out of range");
textErrors.put(CK.CKR_UNWRAPPING_KEY_TYPE_INCONSISTENT, "Unwrapping key type is inconsistent with mechanism");
textErrors.put(CK.CKR_USER_ALREADY_LOGGED_IN, "User is already logged in");
textErrors.put(CK.CKR_USER_NOT_LOGGED_IN, "User is not logged in");
textErrors.put(CK.CKR_USER_TYPE_INVALID, "User type is invalid");
textErrors.put(CK.CKR_WRAPPED_KEY_INVALID, "Wrapped key is invalid");
textErrors.put(CK.CKR_WRAPPED_KEY_LEN_RANGE, "Wrapped key length is out of range");
textErrors.put(CK.CKR_WRAPPING_KEY_SIZE_RANGE, "Wrapping key size is out of range");
textErrors.put(CK.CKR_KEY_FUNCTION_NOT_PERMITTED, "Key function is not permitted");
textErrors.put(CK.CKR_KEY_NOT_WRAPPABLE, "Key is not wrappeble");
textErrors.put(CK.CKR_KEY_UNEXTRACTABLE, "Key is unextractable");
textErrors.put(CK.CKR_TEMPLATE_INCOMPLETE, "Function has inappropriate arguments");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy