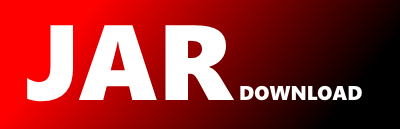
com.unbound.client.kmip.KMIPCipherOper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.client.kmip;
import com.unbound.client.CipherMode;
import com.unbound.client.CipherOper;
import com.unbound.client.KeyObject;
import com.unbound.client.ObjectType;
import com.unbound.common.Log;
import com.unbound.kmip.KMIP;
import com.unbound.kmip.attribute.*;
import com.unbound.kmip.object.KeyBlock;
import com.unbound.kmip.request.*;
import com.unbound.kmip.response.*;
import com.unbound.provider.KeyParameters;
import java.util.ArrayList;
public class KMIPCipherOper extends CipherOper
{
private byte[] corr = null;
private long getKeyUid() { return ((KMIPObject)keyObject).uid; }
private KMIPSession getKmipSession() { return (KMIPSession)session; }
@Override
public void reset()
{
corr = null;
super.reset();
}
private MessageExt setCryptoParams(CryptoParams params)
{
params.cryptoAlg = ((KMIPObject)keyObject).type.getKmipAlg();
if (hashType!=null) params.hashingAlg = hashType.getKmipHashAlg();
params.mode = mode.getKmipBlockCipherMode();
params.padding = mode.getKmipPadding(padding);
MessageExt ext = null;
if (iv!=null)
{
ext = new MessageExt();
ext.iv = iv;
}
if (mode ==CipherMode.GCM)
{
ext = new MessageExt();
ext.iv = iv;
ext.auth = authParam(auth);
params.tagLength = tagLen;
}
if (mode ==CipherMode.CTR)
{
params.counterLength = 32;
}
if (mode ==CipherMode.RSA_OAEP)
{
ext = new MessageExt();
ext.mgf = mgfHashType.getKmipHashAlg();
ext.auth = authParam(oaepLabel);
}
return ext;
}
private byte[] execEnc(byte[] in, boolean wrapPurpose, boolean doFinal)
{
int outLen = 0;
Log log = Log.func("KMIPCryptoOperation.encrypt")
.log("doFinal", doFinal)
.logLen("inLen", in).end(); try
{
EncryptRequest req = new EncryptRequest();
req.corr = corr;
req.data = in;
req.initInd = corr==null;
req.finalInd = doFinal;
req.uid = KMIPObject.uidToStr(getKeyUid());
req.iv = iv;
req.params = new CryptoParams();
req.ext = setCryptoParams(req.params);
if (wrapPurpose) req.ext.encDecForWrapUnwrap = true;
EncryptResponse resp;
resp = (EncryptResponse) getKmipSession().transmit(req);
corr = resp.corr;
outLen = resp.data.length;
return resp.data;
}
catch (Exception e) { log.failed(e); throw e; } finally { log.leavePrint().log("outLen", outLen).end(); }
}
private byte[] execDec(byte[] in, boolean unwrapPurpose, boolean doFinal)
{
int outLen = 0;
Log log = Log.func("KMIPCryptoOperation.decrypt")
.log("doFinal", doFinal)
.logLen("inLen", in).end(); try
{
DecryptRequest req = new DecryptRequest();
req.corr = corr;
req.data = in;
req.initInd = corr==null;
req.finalInd = doFinal;
req.uid = KMIPObject.uidToStr(getKeyUid());
req.iv = iv;
req.params = new CryptoParams();
req.ext = setCryptoParams(req.params);
if (unwrapPurpose) req.ext.encDecForWrapUnwrap = true;
DecryptResponse resp;
resp = (DecryptResponse) getKmipSession().transmit(req);
corr = resp.corr;
outLen = resp.data.length;
return resp.data;
}
catch (Exception e) { log.failed(e); throw e; } finally { log.leavePrint().log("outLen", outLen).end(); }
}
private static ArrayList authParam(byte[] auth)
{
if (auth==null) auth = new byte[0];
ArrayList a = new ArrayList();
a.add(auth);
return a;
}
@Override
protected byte[] hwUpdateEnc(byte[] in)
{
return execEnc(in, false, false);
}
@Override
protected byte[] hwFinalEnc(byte[] in)
{
return execEnc(in, false, true);
}
@Override
protected byte[] hwEnc(byte[] in, boolean wrapPurpose)
{
return execEnc(in, wrapPurpose, true);
}
@Override
protected byte[] hwUpdateDec(byte[] in)
{
return execDec(in, false, false);
}
@Override
protected byte[] hwFinalDec(byte[] in)
{
return execDec(in, false, true);
}
@Override
protected byte[] hwDec(byte[] in, boolean unwrapPurpose)
{
return execDec(in, unwrapPurpose, true);
}
@Override
protected byte[] hwWrap(KeyObject wrappedKey)
{
int outLen = 0;
Log log = Log.func("KMIPCryptoOperation.wrap").end(); try
{
GetRequest req = new GetRequest();
req.uid = ((KMIPObject)wrappedKey).getUidStr();
req.formatType = KMIP.KeyFormatType.Raw;
req.keyWrap = new KeyWrappingSpec();
req.keyWrap.encKey = new KeyWrappingInfo();
req.keyWrap.encKey.uid = KMIPObject.uidToStr(getKeyUid());
req.keyWrap.encKey.params = new CryptoParams();
req.ext = setCryptoParams(req.keyWrap.encKey.params);
GetResponse resp = (GetResponse)getKmipSession().transmit(req);
KeyBlock keyBlock = (resp.objectType == KMIP.ObjectType.PrivateKey) ?
((com.unbound.kmip.object.PrivateKey)resp.object).keyBlock :
((com.unbound.kmip.object.SymmetricKey)resp.object).keyBlock;
outLen = keyBlock.buf.length;
return keyBlock.buf;
}
catch (Exception e) { log.failed(e); throw e; } finally { log.leavePrint().log("outLen", outLen).end(); }
}
@Override
protected KeyObject hwUnwrap(byte[] in, String name, ObjectType type, KeyParameters kp)
{
long uid = 0;
Log log = Log.func("KMIPCryptoOperation.unwrap").end(); try
{
RegisterRequest req = new RegisterRequest();
req.objectType = type.getKmipObjectType();
req.template = KMIPObject.getTemplate(name, type, kp);
KeyBlock keyBlock = new KeyBlock();
keyBlock.keyWrap = new KeyWrappingData();
keyBlock.keyWrap.encKey = new KeyWrappingInfo();
keyBlock.keyWrap.encKey.uid = KMIPObject.uidToStr(getKeyUid());
keyBlock.keyWrap.encKey.params = new CryptoParams();;
keyBlock.keyWrap.iv = iv;
keyBlock.formatType = KMIP.KeyFormatType.Raw;
keyBlock.buf = in;
req.ext = setCryptoParams(keyBlock.keyWrap.encKey.params);
if (type.isPrivateKey())
{
com.unbound.kmip.object.PrivateKey privateKey = new com.unbound.kmip.object.PrivateKey();
req.object = privateKey;
privateKey.keyBlock = keyBlock;
}
else
{
com.unbound.kmip.object.SymmetricKey symmetricKey = new com.unbound.kmip.object.SymmetricKey();
req.object = symmetricKey;
symmetricKey.keyBlock = keyBlock;
}
uid = KMIPObject.register(getKmipSession(), req);
return (KeyObject) KMIPObject.newObject(type, getKmipSession(), uid);
}
catch (Exception e) { log.failed(e); throw e; } finally { log.leavePrint().logHex("uid", uid).end(); }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy