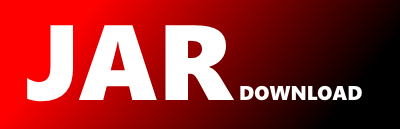
com.unbound.client.kmip.KMIPMacOper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.client.kmip;
import com.unbound.client.MacOper;
import com.unbound.common.Log;
import com.unbound.kmip.attribute.CryptoParams;
import com.unbound.kmip.attribute.MessageExt;
import com.unbound.kmip.request.MACRequest;
import com.unbound.kmip.response.MACResponse;
public class KMIPMacOper extends MacOper
{
private byte[] corr = null;
private long getKeyUid() { return ((KMIPObject)keyObject).uid; }
private KMIPSession getKmipSession() { return (KMIPSession)session; }
@Override
public void reset()
{
corr = null;
super.reset();
}
private void setCryptoParams(MACRequest req)
{
req.params = new CryptoParams();
req.params.cryptoAlg = ((KMIPObject)keyObject).type.getKmipAlg();
if (hashType!=null)
{
req.params.cryptoAlg = mode.getKmipAlg(hashType);
req.params.hashingAlg = hashType.getKmipHashAlg();
}
else
{
req.params.mode = mode.getKmipBlockMode();
}
if (iv!=null)
{
req.ext = new MessageExt();
req.ext.iv = iv;
}
}
private byte[] execMac(byte[] in, boolean doFinal)
{
int outLen = 0;
Log log = Log.func("KMIPCryptoOperation.mac")
.log("doFinal", doFinal)
.logLen("length", in).end(); try
{
MACRequest req = new MACRequest();
req.corr = corr;
req.data = in;
req.initInd = corr==null;
req.finalInd = doFinal;
req.uid = KMIPObject.uidToStr(getKeyUid());
setCryptoParams(req);
MACResponse resp = (MACResponse)getKmipSession().transmit(req);
corr = resp.corr;
return resp.data;
}
catch (Exception e) { log.failed(e); throw e; } finally { log.leavePrint().log("outLen", outLen).end(); }
}
@Override
protected void hwUpdateMac(byte[] in)
{
execMac(in, false);
}
@Override
protected byte[] hwFinalMac(byte[] in)
{
return execMac(in, true);
}
@Override
protected byte[] hwMac(byte[] in)
{
return hwFinalMac(in);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy