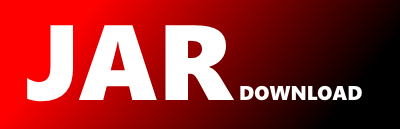
com.unbound.client.kmip.KMIPObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.client.kmip;
import com.dyadicsec.cryptoki.CK;
import com.dyadicsec.cryptoki.CKR_Exception;
import com.dyadicsec.cryptoki.CK_ATTRIBUTE;
import com.unbound.client.*;
import com.unbound.common.HEX;
import com.unbound.common.Log;
import com.unbound.kmip.KMIP;
import com.unbound.kmip.attribute.*;
import com.unbound.kmip.object.ManagedObject;
import com.unbound.kmip.request.*;
import com.unbound.kmip.response.*;
import com.unbound.provider.KeyParameters;
import javax.security.auth.x500.X500Principal;
import java.security.ProviderException;
import java.security.cert.CertificateEncodingException;
import java.util.ArrayList;
import java.util.Date;
import static com.unbound.common.Converter.setBE8;
public class KMIPObject implements BaseObject
{
protected final ObjectType type;
protected final long uid;
protected long replacedUid = 0;
protected KMIPPartition partition = null;
protected long initialDate = 0;
protected String name = null;
static long strToUid(String str)
{
if (str.length() == 16) str = "0x00" + str;
if (str.length() == 20 && str.charAt(0) == '0' && str.charAt(1) == 'x' && str.charAt(2) == '0' && str.charAt(3) == '0')
{
long uid = 0;
for (int i = 4; i < 20; i += 2)
{
int hi = HEX.from(str.charAt(i));
int lo = HEX.from(str.charAt(i + 1));
if (lo < 0 || hi < 0) return 0;
uid = (uid << 8) | (hi << 4) | lo;
}
return uid;
}
return 0;
}
static String uidToStr(long uid)
{
byte[] cka_id = new byte[9];
cka_id[0] = 0;
setBE8(cka_id, 1, uid);
char[] out = new char[20];
out[0] = '0';
out[1] = 'x';
for (int i = 0; i < cka_id.length; i++)
{
out[2 + i * 2 + 0] = HEX.chars[(cka_id[i] >> 4) & 0x0f];
out[2 + i * 2 + 1] = HEX.chars[cka_id[i] & 0x0f];
}
return new String(out);
}
void acceptManagedObject(ManagedObject managedObject) {}
public static KMIPObject newObject(ObjectType type, KMIPSession session, long uid)
{
switch (type.getKmipObjectType())
{
case KMIP.ObjectType.Certificate: return new KMIPCert(session, uid);
case KMIP.ObjectType.SymmetricKey: return new KMIPSecretKey(type, session, uid);
case KMIP.ObjectType.PublicKey:
switch (type.getKmipAlg())
{
case KMIP.CryptographicAlgorithm.RSA: return new KMIPRSAPublicKey(session, uid);
}
break;
case KMIP.ObjectType.PrivateKey:
switch (type.getKmipAlg())
{
case KMIP.CryptographicAlgorithm.DyPRF: return new KMIPECPRFKey(session, uid);
case KMIP.CryptographicAlgorithm.EC: return new KMIPECPrivateKey(session, uid);
case KMIP.CryptographicAlgorithm.RSA: return new KMIPRSAPrivateKey(session, uid);
}
break;
}
throw new ProviderException("Unsupported object type");
}
KMIPObject(ObjectType type, long uid)
{
this.uid = uid;
this.type = type;
}
protected void read(KMIPSession session)
{
Log log = Log.func("KMIPObject.read").logHex("uid", uid).log("type", type.getAlgName()).end(); try
{
partition = (KMIPPartition) session.getPartition();
boolean getManagedObject = false;
switch (type.getKmipObjectType())
{
case KMIP.ObjectType.Certificate:
case KMIP.ObjectType.PrivateKey:
case KMIP.ObjectType.PublicKey:
getManagedObject = true;
break;
}
RequestMessage reqMsg = new RequestMessage();
GetAttributesRequest getAttr = new GetAttributesRequest();
getAttr.uid = uidToStr(uid);
//getAttr.names.add("Object Type");
getAttr.names.add("Name");
getAttr.names.add("Initial Date");
getAttr.names.add("Link");
reqMsg.batch.add(getAttr);
if (getManagedObject)
{
GetRequest getReq = new GetRequest();
getReq.formatType = KMIP.KeyFormatType.X_509;
reqMsg.batch.add(getReq);
}
ResponseMessage respMsg = session.transmit(reqMsg);
GetAttributesResponse getAttrResp = (GetAttributesResponse)respMsg.batch.get(0);
name = ((Name)getAttrResp.attrs.get(0)).value;
initialDate = ((DateAttribute)getAttrResp.attrs.get(1)).value;
for (int i=2; i locate(KMIPSession session, ObjectType type)
{
int kmipType = type.getKmipObjectType();
int kmipAlg = type.getKmipAlg();
ArrayList list = new ArrayList();
Log log = Log.func("KMIPObject.locate").
log("objectType", kmipType).
log("alg", kmipAlg).end(); try
{
LocateRequest req = new LocateRequest();
req.maxItems = 1024;
req.attrs.add(new EnumAttribute(KMIP.Tag.State, KMIP.State.Active));
req.attrs.add(new EnumAttribute(KMIP.Tag.ObjectType, kmipType));
if (kmipAlg!=-1) req.attrs.add(new EnumAttribute(KMIP.Tag.CryptographicAlgorithm, kmipAlg));
LocateResponse resp = (LocateResponse)session.transmit(req);
for (String s: resp.list)
{
list.add(newObject(type, session, strToUid(s)));
}
return list;
}
catch (Exception e) { log.failed(e); throw new ProviderException(e); } finally { log.leave(); }
}
static void makeExportLevel(TemplateAttribute t, KeyParameters kp)
{
switch (kp.getExportProtection())
{
case -1:
case KeyParameters.EXPORT_NONE:
break;
case KeyParameters.EXPORT_WRAP:
t.attrs.add(new BoolAttribute(KMIP.Tag.CKA_EXTRACTABLE, true));
break;
case KeyParameters.EXPORT_WRAP_WITH_TRUSTED:
t.attrs.add(new BoolAttribute(KMIP.Tag.CKA_EXTRACTABLE, true));
t.attrs.add(new BoolAttribute(KMIP.Tag.CKA_WRAP_WITH_TRUSTED, true));
break;
case KeyParameters.EXPORT_PLAIN:
t.attrs.add(new BoolAttribute(KMIP.Tag.CKA_SENSITIVE, true));
break;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy