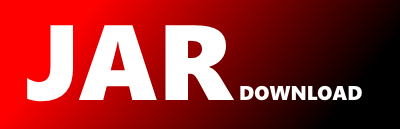
com.unbound.client.pkcs11.PKCS11CipherOper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.client.pkcs11;
import com.dyadicsec.cryptoki.*;
import com.unbound.client.CipherOper;
import com.unbound.client.KeyObject;
import com.unbound.client.ObjectType;
import com.unbound.common.Converter;
import com.unbound.provider.KeyParameters;
import java.security.ProviderException;
import java.util.ArrayList;
public final class PKCS11CipherOper extends CipherOper
{
private CK_MECHANISM mechanism = null;
private int getKeyHandle() { return ((PKCS11Object)keyObject).handle; }
private CK_SESSION_HANDLE getSessionHandle() { return ((PKCS11Session)session).getHandle(); }
private void markOperationStarted() { ((PKCS11Session)session).setOperationInProgress(true); }
private void markOperationFinished() { ((PKCS11Session)session).setOperationInProgress(false); }
@Override
public void reset()
{
mechanism = null;
super.reset();
}
private void checkMechanism()
{
if (mechanism!=null) return;
Object parameter = null;
ObjectType objectType = ((PKCS11Object)keyObject).objectType;
int mech = mode.getPkcs11Mech(objectType, padding);
switch (mech)
{
case CK.CKM_AES_CBC_PAD:
case CK.CKM_AES_CBC:
case CK.CKM_DES3_CBC:
case CK.CKM_AES_OFB:
case CK.CKM_AES_CFB128:
case CK.DYCKM_AES_XTS:
case CK.CKM_DES3_CBC_PAD:
case CK.CKM_DES_CFB64:
case CK.CKM_DES_OFB64:
case CK.CKM_AES_KEY_WRAP:
case CK.CKM_AES_KEY_WRAP_PAD:
parameter = iv;
break;
case CK.CKM_AES_CTR:
{
CK_AES_CTR_PARAMS param = new CK_AES_CTR_PARAMS();
param.ulCounterBits = 32;
param.cb = iv;
parameter = param;
}
break;
case CK.CKM_AES_GCM:
{
CK_GCM_PARAMS param = new CK_GCM_PARAMS();
param.pIv = iv;
param.pAAD = auth;
param.ulTagBits = 96;
parameter = param;
}
break;
case CK.CKM_AES_CCM:
{
CK_CCM_PARAMS param = new CK_CCM_PARAMS();
param.pAAD = auth;
param.pNonce = iv;
param.ulDataLen = dataLen;
param.ulMACLen = tagLen;
parameter = param;
}
break;
case CK.DYCKM_AES_SIV:
{
DYCK_AES_SIV_PARAMS param = new DYCK_AES_SIV_PARAMS();
if (siv_auth!=null && !siv_auth.isEmpty())
{
param.pAuthData = new byte[siv_auth.size()][];
siv_auth.toArray(param.pAuthData);
}
parameter = param;
}
break;
case CK.CKM_RSA_PKCS_OAEP:
{
CK_RSA_PKCS_OAEP_PARAMS param = new CK_RSA_PKCS_OAEP_PARAMS();
param.pSourceData = oaepLabel;
param.hashAlg = hashType.getPkcs11Mech();
param.mgf = mgfHashType.getPkcs11Mgf();
parameter = param;
}
break;
case CK.DYCKM_FPE:
{
DYCK_FPE_PARAMS param = new DYCK_FPE_PARAMS();
param.ulMode = fpeMode;
param.pFormat = fpeFormat==null ? null : fpeFormat.toCharArray();
param.ulMaxLen = fpeMaxLen;
parameter = param;
}
break;
case CK.DYCKM_SPE:
{
DYCK_SPE_PARAMS param = new DYCK_SPE_PARAMS();
param.ulBits = speBits;
parameter = param;
}
break;
case CK.CKM_AES_ECB:
case CK.CKM_DES3_ECB:
case CK.CKM_RSA_X_509:
case CK.CKM_RSA_PKCS:
case CK.DYCKM_OPE:
break;
default:
throw new ProviderException("Unsupported cipher mechanism");
}
mechanism = new CK_MECHANISM(mech, parameter);
}
private void checkCipher()
{
if (((PKCS11Session)session).isOperationInProgress()) return;
try
{
checkMechanism();
if (encMode) Library.C_EncryptInit(getSessionHandle(), mechanism, getKeyHandle());
else Library.C_DecryptInit(getSessionHandle(), mechanism, getKeyHandle());
markOperationStarted();
}
catch (CKR_Exception e) { throw new ProviderException(e); }
}
@Override
protected byte[] hwUpdateEnc(byte[] in)
{
try
{
checkCipher();
return Library.C_EncryptUpdate(getSessionHandle(), in);
}
catch (CKR_Exception e) { throw new ProviderException(e); }
}
@Override
protected byte[] hwFinalEnc(byte[] in)
{
if (mechanism==null) return hwEnc(in, false);
try
{
checkCipher();
byte[] out1 = null;
if (in!=null && in.length>0) out1 = hwUpdateEnc(in);
byte[] out2 = Library.C_EncryptFinal(getSessionHandle());
markOperationFinished();
return Converter.concat(out1, out2);
}
catch (CKR_Exception e) { throw new ProviderException(e); }
}
@Override
protected byte[] hwEnc(byte[] in, boolean wrapPurpose)
{
try
{
byte[] out = null;
if (!wrapPurpose)
{
checkCipher();
out = Library.C_Encrypt(getSessionHandle(), in);
}
else
{
checkMechanism();
int tempHandle = Library.C_CreateObject(getSessionHandle(), new CK_ATTRIBUTE[]
{
new CK_ATTRIBUTE(CK.CKA_TOKEN, false),
new CK_ATTRIBUTE(CK.CKA_CLASS, CK.CKO_SECRET_KEY),
new CK_ATTRIBUTE(CK.CKA_KEY_TYPE, CK.CKK_GENERIC_SECRET),
new CK_ATTRIBUTE(CK.CKA_SENSITIVE, false),
new CK_ATTRIBUTE(CK.CKA_VALUE, in),
});
markOperationStarted();
out = Library.C_WrapKey(getSessionHandle(), mechanism, getKeyHandle(), tempHandle);
Library.C_DestroyObject(getSessionHandle(), tempHandle);
}
markOperationFinished();
return out;
}
catch (CKR_Exception e) { throw new ProviderException(e); }
}
@Override
protected byte[] hwUpdateDec(byte[] in)
{
try
{
checkCipher();
return Library.C_DecryptUpdate(getSessionHandle(), in);
}
catch (CKR_Exception e) { throw new ProviderException(e); }
}
@Override
protected byte[] hwFinalDec(byte[] in)
{
if (mechanism==null) return hwDec(in, false);
try
{
checkCipher();
byte[] out1 = null;
if (in!=null && in.length>0) out1 = hwUpdateDec(in);
byte[] out2 = Library.C_DecryptFinal(getSessionHandle());
markOperationFinished();
return Converter.concat(out1, out2);
}
catch (CKR_Exception e) { throw new ProviderException(e); }
}
@Override
protected byte[] hwDec(byte[] in, boolean unwrapPurpose)
{
try
{
byte[] out = null;
if (!unwrapPurpose)
{
checkCipher();
out = Library.C_Decrypt(getSessionHandle(), in);
}
else
{
checkMechanism();
int tempHandle = Library.C_UnwrapKey(getSessionHandle(), mechanism, getKeyHandle(), in, new CK_ATTRIBUTE[]
{
new CK_ATTRIBUTE(CK.CKA_TOKEN, false),
new CK_ATTRIBUTE(CK.CKA_CLASS, CK.CKO_SECRET_KEY),
new CK_ATTRIBUTE(CK.CKA_KEY_TYPE, CK.CKK_GENERIC_SECRET),
new CK_ATTRIBUTE(CK.CKA_SENSITIVE, false),
});
markOperationStarted();
CK_ATTRIBUTE[] v = { new CK_ATTRIBUTE(CK.CKA_VALUE) };
Library.C_GetAttributeValue(getSessionHandle(), tempHandle, v);
out = (byte[])v[0].pValue;
Library.C_DestroyObject(getSessionHandle(), tempHandle);
}
markOperationFinished();
return out;
}
catch (CKR_Exception e) { throw new ProviderException(e); }
}
@Override
protected byte[] hwWrap(KeyObject wrappedKey)
{
try
{
checkMechanism();
byte[] out = Library.C_WrapKey(getSessionHandle(), mechanism, getKeyHandle(), ((PKCS11Object)wrappedKey).handle);
markOperationFinished();
return out;
}
catch (CKR_Exception e) { throw new ProviderException(e); }
}
@Override
protected KeyObject hwUnwrap(byte[] in, String name, ObjectType type, KeyParameters kp)
{
try
{
checkMechanism();
ArrayList t = PKCS11Object.getNewTemplate(name, type, kp);
int unwrappedKeyHandle = Library.C_UnwrapKey(getSessionHandle(), mechanism, getKeyHandle(), in,
PKCS11Object.getAttrs(t));
markOperationFinished();
return (KeyObject) PKCS11Object.newObject(type, (PKCS11Session)session, unwrappedKeyHandle);
}
catch (CKR_Exception e) { throw new ProviderException(e); }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy