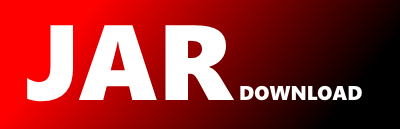
com.unbound.client.pkcs11.PKCS11Client Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.client.pkcs11;
import com.dyadicsec.cryptoki.CKR_Exception;
import com.dyadicsec.cryptoki.Library;
import com.unbound.client.*;
import com.unbound.common.Log;
import com.unbound.provider.UBCryptoProvider;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.math.BigInteger;
import java.security.KeyStore;
import java.security.ProviderException;
import java.security.cert.CertificateException;
import java.security.cert.CertificateFactory;
import java.security.cert.X509Certificate;
public final class PKCS11Client extends Client
{
static
{
try
{
Library.C_Initialize();
int[] slots = Library.C_GetSlotList(true);
for (int slot : slots) PKCS11Partition.register(slot);
UBCryptoProvider.allowPrivateKeyWithoutCertificate(true);
UBCryptoProvider.allowPublicKey(true);
}
catch (CKR_Exception e) { }
}
private static PKCS11Client instance = new PKCS11Client();
public static Client getInstance()
{
return instance;
}
@Override
public X509Certificate selfSign(PrivateKeyObject key, String hashAlg, String subject, BigInteger serialNumber, int days) throws CertificateException
{
Log log = Log.func("PKCS11Client.selfSign").log("subject", subject).end();
try
{
HashType hashType = HashType.SHA256;
if (hashAlg != null && !hashAlg.isEmpty()) hashType = HashType.getFromName(hashAlg);
char[] serialChars = subject.toCharArray();
byte[] subjectBytes = serialNumber == null ? null : serialNumber.toByteArray();
PKCS11Partition pkcs11Partition = (PKCS11Partition)key.getPartition();
PKCS11Session connection = null;
byte[] bytes = null;
try
{
connection = (PKCS11Session)pkcs11Partition.acquireSession();
bytes = Library.DYC_SelfSignX509(connection.getHandle(), ((PKCS11Object) key).handle, hashType.getPkcs11Mech(), serialChars, subjectBytes, days);
}
finally { pkcs11Partition.releaseSession(connection); }
CertificateFactory certFactory = CertificateFactory.getInstance("X.509");
InputStream in = new ByteArrayInputStream(bytes);
return (X509Certificate) certFactory.generateCertificate(in);
}
catch (Exception e) { log.failed(e); throw new ProviderException(e); } finally { log.leave(); }
}
@Override
public CipherOper newCipherOperation() { return new PKCS11CipherOper(); }
@Override
public MacOper newMacOperation() { return new PKCS11MacOper(); }
@Override
public SignatureOper newSignatureOperation() { return new PKCS11SignatureOper(); }
@Override
public DeriveOper newDeriveOperation() { return new PKCS11DeriveOper(); }
@Override
public void initProviders(String[] servers, KeyStore trusted)
{
throw new ProviderException("Clientless configuration is not supported");
}
@Override
public Partition getPartition(String name)
{
return PKCS11Partition.get(name);
}
@Override
public Partition initProvider(String arg)
{
Log log = Log.func("PKCS11Client.initProvider").log("configArg", arg).end();
try
{
if (arg == null || arg.isEmpty()) arg = System.getProperty("ekm.partition");
if (arg == null || arg.isEmpty()) arg = System.getenv().get("EKM_PARTITION");
PKCS11Partition partition = PKCS11Partition.get(arg);
if (partition == null) throw new ProviderException(String.format("Partition %s not found", arg));
return partition;
}
catch (Exception e) { log.failed(e); throw e; } finally { log.leave(); }
}
@Override
public Partition initProvider(KeyStore pfx, String pfxPass)
{
throw new ProviderException("Clientless configuration is not supported");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy