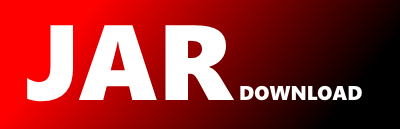
com.unbound.client.pkcs11.PKCS11DeriveOper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.client.pkcs11;
import com.dyadicsec.cryptoki.*;
import com.unbound.client.DeriveOper;
import com.unbound.client.ObjectType;
import com.unbound.client.SecretKeyObject;
import com.unbound.common.crypto.EC;
import com.unbound.provider.KeyParameters;
import java.security.ProviderException;
import java.util.ArrayList;
public final class PKCS11DeriveOper extends DeriveOper
{
public PKCS11DeriveOper()
{
}
public PKCS11DeriveOper(PKCS11Session session)
{
isExternalSession = true;
this.session = session;
}
private boolean isExternalSession = false;
private int getKeyHandle() { return ((PKCS11Object)keyObject).handle; }
private CK_SESSION_HANDLE getSessionHandle() { return ((PKCS11Session)session).getHandle(); }
private void markOperationStarted() { ((PKCS11Session)session).setOperationInProgress(true); }
private void markOperationFinished() { ((PKCS11Session)session).setOperationInProgress(false); }
private CK_MECHANISM getMechanism()
{
Object parameter = null;
int mech = mode.getPkcs11Mech();
switch (mech)
{
case CK.CKM_ECDH1_DERIVE:
{
CK_ECDH1_DERIVE_PARAMS ecdh = new CK_ECDH1_DERIVE_PARAMS();
EC.Curve curve = ((PKCS11ECPrivateKey)keyObject).getCurve();
ecdh.pPublicData = curve.toDer(ecdhPubKey);
ecdh.kdf = CK.CKD_NULL;
parameter = ecdh;
}
break;
case CK.DYCKM_PRF:
{
DYCK_PRF_PARAMS params = new DYCK_PRF_PARAMS();
params.ulPurpose = prfPurpose;
params.pTweak = prfTweak;
params.ulSecretLen = resultLen;
parameter = params;
}
break;
default:
throw new ProviderException("Unsupported derivation mechanism");
}
return new CK_MECHANISM(mech, parameter);
}
@Override
protected byte[] hwDerive()
{
try
{
int tempHandle = Library.C_DeriveKey(getSessionHandle(), getMechanism(), getKeyHandle(),
new CK_ATTRIBUTE[]
{
new CK_ATTRIBUTE(CK.CKA_TOKEN, false),
new CK_ATTRIBUTE(CK.CKA_CLASS, CK.CKO_SECRET_KEY),
new CK_ATTRIBUTE(CK.CKA_KEY_TYPE, CK.CKK_GENERIC_SECRET),
new CK_ATTRIBUTE(CK.CKA_SENSITIVE, false),
});
markOperationStarted();
CK_ATTRIBUTE[] v = { new CK_ATTRIBUTE(CK.CKA_VALUE) };
Library.C_GetAttributeValue(getSessionHandle(), tempHandle, v);
Library.C_DestroyObject(getSessionHandle(), tempHandle);
markOperationFinished();
if (isExternalSession) session = null;
return (byte[])v[0].pValue;
}
catch (CKR_Exception e) { throw new ProviderException(e); }
}
@Override
protected SecretKeyObject hwDeriveKey(ObjectType objectType, String name, KeyParameters kp)
{
try
{
ArrayList t = PKCS11SecretKey.getNewTemplate(name, objectType, kp);
int derivedKeyHandle = Library.C_DeriveKey(getSessionHandle(), getMechanism(), getKeyHandle(), PKCS11Object.getAttrs(t));
PKCS11SecretKey derivedKey = new PKCS11SecretKey(objectType, (PKCS11Session) session, derivedKeyHandle);
if (isExternalSession) session = null;
return derivedKey;
}
catch (CKR_Exception e) { throw new ProviderException(e); }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy