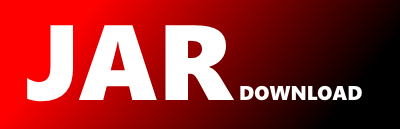
com.unbound.client.pkcs11.PKCS11Session Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.client.pkcs11;
import com.dyadicsec.cryptoki.*;
import com.unbound.client.*;
import com.unbound.common.crypto.EC;
import com.unbound.provider.*;
import java.security.cert.X509Certificate;
import java.security.interfaces.ECPrivateKey;
import java.security.interfaces.RSAPrivateCrtKey;
import java.security.interfaces.RSAPublicKey;
import java.util.ArrayList;
public final class PKCS11Session implements Session
{
private final PKCS11Partition partition;
private CK_SESSION_HANDLE handle;
private boolean operationInProgress = false;
static PKCS11Session open(PKCS11Partition partition, int slotId) throws CKR_Exception
{
CK_SESSION_HANDLE session = Library.C_OpenSession(slotId, CK.CKF_RW_SESSION | CK.CKF_SERIAL_SESSION);
return new PKCS11Session(partition, session);
}
public boolean isOperationInProgress() { return operationInProgress; }
public void setOperationInProgress(boolean operationInProgress) { this.operationInProgress = operationInProgress; }
public CK_SESSION_HANDLE getHandle() { return handle; }
private PKCS11Session(PKCS11Partition partition, CK_SESSION_HANDLE handle)
{
this.partition = partition;
this.handle = handle;
}
void close()
{
if (handle !=null)
{
try { Library.C_CloseSession(handle); }
catch (CKR_Exception e) { }
}
handle = null;
}
@Override
public Partition getPartition()
{
return partition;
}
@Override
public void release() { partition.releaseSession(this); }
@Override
public SecretKeyObject generateSecretKey(String name, ObjectType type, int bitSize, KeyParameters kp)
{
return PKCS11SecretKey.generate(this, name, type, bitSize, kp);
}
@Override
public ECPRFKey generateEcprfKey(String name, KeyParameters kp)
{
return PKCS11ECPRFKey.generate(this, name, kp);
}
@Override
public SecretKeyObject importSecretKey(String name, ObjectType type, byte[] keyValue, KeyParameters kp)
{
return PKCS11SecretKey.importKey(this, name, type, keyValue, kp);
}
@Override
public EDDSAPrivateKeyObject generateEddsaKey(String name, KeyParameters kp)
{
return PKCS11EDDSAPrivateKey.generate(this, name, kp);
}
@Override
public RSAPrivateKeyObject generateRsaKey(String name, int bitSize, KeyParameters kp)
{
return PKCS11RSAPrivateKey.generate(this, name, bitSize, kp);
}
@Override
public RSAPrivateKeyObject importRsaKey(String name, RSAPrivateCrtKey keyValue, KeyParameters kp)
{
return PKCS11RSAPrivateKey.importKey(this, name, keyValue, kp);
}
@Override
public ECPrivateKeyObject generateEcKey(String name, EC.Curve curve, KeyParameters kp)
{
return PKCS11ECPrivateKey.generate(this, name, curve, kp);
}
@Override
public RSAPublicKeyObject importPubRsaKey(String name, RSAPublicKey keyValue, KeyParameters kp)
{
return PKCS11RSAPublicKey.importKey(this, name, keyValue, kp);
}
@Override
public ECPrivateKeyObject importEcKey(String name, ECPrivateKey keyValue, KeyParameters kp)
{
return PKCS11ECPrivateKey.importKey(this, name, keyValue, kp);
}
@Override
public CertObject importCert(String name, X509Certificate cert)
{
return PKCS11Cert.importCert(this, name, cert);
}
@Override
public BaseObject locate(ObjectType type, LocateParams params)
{
return PKCS11Object.locate(this, type, params);
}
@Override
public ArrayList locate(ObjectType type)
{
return PKCS11Object.locate(this, type);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy