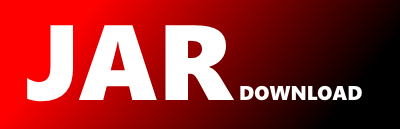
com.unbound.provider.UBRSASignaturePSS Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.provider;
import com.unbound.client.*;
import com.unbound.common.crypto.SystemProvider;
import java.security.*;
import java.security.interfaces.RSAPublicKey;
import java.security.spec.AlgorithmParameterSpec;
import java.security.spec.MGF1ParameterSpec;
import java.security.spec.PSSParameterSpec;
public final class UBRSASignaturePSS extends SignatureSpi
{
private final SignatureOper oper = Client.getInstance().newSignatureOperation();
private PSSParameterSpec pss = null;
public UBRSASignaturePSS()
{
oper.mode = SignatureMode.RSA_PSS;
oper.pssSaltLen = 20;
oper.hashType = HashType.SHA1;
oper.mgfHashType = HashType.SHA1;
}
// ------------------------- interface ---------------------------------
@Override
protected void engineInitVerify(PublicKey publicKey) throws InvalidKeyException
{
if (!(publicKey instanceof RSAPublicKey)) throw new InvalidKeyException("Invalid key type");
oper.reset();
try
{
oper.swSignature = SystemProvider.Signature.getInstance("RSASSA-PSS");
if (pss==null) pss = new PSSParameterSpec("SHA-1", "MGF1", null, 20, 1);
oper.swSignature.setParameter(pss);
oper.swSignature.initVerify(publicKey);
}
catch (InvalidAlgorithmParameterException e) { throw new InvalidKeyException(e); }
}
@Override
protected void engineInitSign(PrivateKey privateKey) throws InvalidKeyException
{
if (!(privateKey instanceof UBRSAPrivateKey)) throw new InvalidKeyException("Invalid key type");
oper.reset();
oper.keyObject = ((UBRSAPrivateKey)privateKey).object;
}
@Override
protected void engineUpdate(byte b) throws SignatureException
{
oper.update(b);
}
@Override
protected void engineUpdate(byte[] in, int inOffset, int inLen) throws SignatureException
{
oper.update(in, inOffset, inLen);
}
@Override
protected byte[] engineSign() throws SignatureException
{
return oper.finalSign();
}
@Override
protected boolean engineVerify(byte[] sigBytes) throws SignatureException
{
return oper.finalVerify(sigBytes);
}
@Override
protected void engineSetParameter(String param, Object value) throws InvalidParameterException
{
throw new UnsupportedOperationException("setParameter() not supported");
}
@Override
protected void engineSetParameter(AlgorithmParameterSpec params) throws InvalidAlgorithmParameterException
{
if (params == null) throw new InvalidAlgorithmParameterException("Parameters cannot be null");
if (!(params instanceof PSSParameterSpec)) throw new InvalidAlgorithmParameterException("parameters must be type PSSParameterSpec");
PSSParameterSpec pss = (PSSParameterSpec)params;
if (!(pss.getMGFAlgorithm().equalsIgnoreCase("MGF1"))) throw new InvalidAlgorithmParameterException("Only supports MGF1");
if (pss.getTrailerField() != 1) throw new InvalidAlgorithmParameterException("Only supports TrailerFieldBC(1)");
oper.pssSaltLen = pss.getSaltLength();
oper.mgfHashType = oper.hashType = HashType.getFromName(pss.getDigestAlgorithm());
AlgorithmParameterSpec mgfParams = pss.getMGFParameters();
if (mgfParams!=null) oper.mgfHashType = HashType.getFromName(((MGF1ParameterSpec)mgfParams).getDigestAlgorithm());
this.pss = pss;
}
@Override
protected Object engineGetParameter(String param) throws InvalidParameterException
{
throw new UnsupportedOperationException("getParameter() not supported");
}
@Override
protected AlgorithmParameters engineGetParameters()
{
if (pss == null) throw new ProviderException("Missing required PSS parameters");
try
{
AlgorithmParameters ap = AlgorithmParameters.getInstance("RSASSA-PSS");
ap.init(pss);
return ap;
}
catch (GeneralSecurityException e) { throw new ProviderException(e); }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy