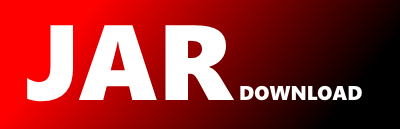
com.unbound.provider.UBSecretKeyCipher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.provider;
import com.unbound.client.*;
import javax.crypto.*;
import javax.crypto.spec.GCMParameterSpec;
import javax.crypto.spec.IvParameterSpec;
import java.security.*;
import java.security.spec.AlgorithmParameterSpec;
import java.security.spec.InvalidParameterSpecException;
public class UBSecretKeyCipher extends CipherSpi
{
private final CipherOper oper = Client.getInstance().newCipherOperation();
private final ObjectType keyType;
private KeyParameters unwrapKeyParameter = null;
private AlgorithmParameterSpec paramSpec = null;
UBSecretKeyCipher(ObjectType keyType)
{
this.keyType = keyType;
}
// ------------------------ interface ----------------------------
@Override
protected void engineSetMode(String modeName) throws NoSuchAlgorithmException
{
oper.mode = CipherMode.getByName(modeName);
}
@Override
protected void engineSetPadding(String padding) throws NoSuchPaddingException
{
if (padding.equalsIgnoreCase("NOPADDING")) oper.padding = false;
else if (padding.equalsIgnoreCase("PKCS5PADDING"))
{
if (oper.mode != CipherMode.CBC) throw new NoSuchPaddingException("padding not supported");
oper.padding = true;
}
else throw new NoSuchPaddingException("padding not supported");
}
@Override
protected int engineGetBlockSize() { return keyType.getBlockSize(); }
@Override
protected int engineGetOutputSize(int inputLen) { return inputLen + (oper.encMode ? 16 : 0); }
@Override
protected byte[] engineGetIV() { return oper.iv; }
@Override
protected AlgorithmParameters engineGetParameters()
{
if (paramSpec==null) return null;
try
{
AlgorithmParameters params = AlgorithmParameters.getInstance("AES", "SunJCE");
params.init(paramSpec);
return params;
}
catch (GeneralSecurityException e) { throw new ProviderException("Could not encode parameters", e); }
}
@Override
protected void engineInit(int opmode, Key key, AlgorithmParameterSpec algorithmParameterSpec, SecureRandom secureRandom) throws InvalidKeyException, InvalidAlgorithmParameterException
{
if (algorithmParameterSpec instanceof KeyGenSpec)
{
if (opmode!=Cipher.UNWRAP_MODE) throw new InvalidAlgorithmParameterException("KeyParameter is supported only in UNWRAP_MODE");
unwrapKeyParameter = ((KeyGenSpec)algorithmParameterSpec).getKeyParams();
algorithmParameterSpec = ((KeyGenSpec)algorithmParameterSpec).getOriginal();
}
else unwrapKeyParameter = null;
paramSpec = algorithmParameterSpec;
switch (opmode)
{
case Cipher.WRAP_MODE: case Cipher.ENCRYPT_MODE: oper.encMode = true; break;
case Cipher.UNWRAP_MODE: case Cipher.DECRYPT_MODE: oper.encMode = false; break;
default: throw new InvalidParameterException("Invalid mode");
}
if (!(key instanceof UBSecretKey)) throw new InvalidKeyException("Invalid key type");
oper.keyObject = ((UBSecretKey)key).object;
if (oper.keyObject.getType()!= keyType) throw new InvalidKeyException("Invalid key type");
oper.mode.setParams(oper, paramSpec);
}
@Override
protected void engineInit(int opmode, Key key, AlgorithmParameters algorithmParameters, SecureRandom secureRandom) throws InvalidKeyException, InvalidAlgorithmParameterException
{
AlgorithmParameterSpec spec = null;
Class extends AlgorithmParameterSpec> clazz = IvParameterSpec.class;
if (oper.mode==CipherMode.GCM) clazz = GCMParameterSpec.class;
if (algorithmParameters!=null)
{
try { spec = algorithmParameters.getParameterSpec(clazz); }
catch (InvalidParameterSpecException ipse) { throw new InvalidAlgorithmParameterException("Wrong parameter"); }
}
engineInit(opmode, key, spec, secureRandom);
}
@Override
protected void engineInit(int opmode, Key key, SecureRandom secureRandom) throws InvalidKeyException
{
try { engineInit(opmode, key, (AlgorithmParameterSpec)null, secureRandom); }
catch (InvalidAlgorithmParameterException e) { throw new InvalidKeyException(e); }
}
@Override
protected void engineUpdateAAD(byte[] in, int inOffset, int inLen) throws IllegalStateException, UnsupportedOperationException
{
oper.updateAuth(in, inOffset, inLen);
}
@Override
protected byte[] engineUpdate(byte[] in, int inOffset, int inLen)
{
return oper.update(in, inOffset, inLen);
}
@Override
protected byte[] engineDoFinal(byte[] in, int inOffset, int inLen) throws IllegalBlockSizeException, BadPaddingException
{
return oper.finalEncDec(in, inOffset, inLen);
}
@Override
protected int engineUpdate(byte[] in, int inOffset, int inLen, byte[] out, int outOffset) throws ShortBufferException
{
return oper.update(in, inOffset, inLen, out, outOffset);
}
@Override
protected int engineDoFinal(byte[] in, int inOffset, int inLen, byte[] out, int outOffset) throws ShortBufferException, IllegalBlockSizeException, BadPaddingException
{
return oper.finalEncDec(in, inOffset, inLen, out, outOffset);
}
@Override
protected byte[] engineWrap(Key key) throws IllegalBlockSizeException, InvalidKeyException
{
if (key instanceof UBSecretKey) return oper.wrap(((UBSecretKey)key).object);
if (key instanceof UBRSAPrivateKey) return oper.wrap(((UBRSAPrivateKey)key).object);
if (key instanceof UBECPrivateKey) return oper.wrap(((UBECPrivateKey)key).object);
return oper.swWrap(key);
}
@Override
protected Key engineUnwrap(byte[] wrappedKey, String algorithm, int wrappedKeyType) throws InvalidKeyException, NoSuchAlgorithmException
{
if (unwrapKeyParameter==null || !unwrapKeyParameter.isToken()) return oper.swUnwrap(wrappedKey, algorithm, wrappedKeyType);
KeyObject keyObject = oper.unwrap(wrappedKey, null, ObjectType.get(algorithm), unwrapKeyParameter);
if (wrappedKeyType == Cipher.SECRET_KEY) return new UBSecretKey((SecretKeyObject) keyObject);
if (algorithm.equalsIgnoreCase("RSA")) return new UBRSAPrivateKey((RSAPrivateKeyObject) keyObject);
if (algorithm.equalsIgnoreCase("EC")) return new UBECPrivateKey((ECPrivateKeyObject) keyObject);
throw new ProviderException("Unsupported wrapped key type");
}
// ----------------------------- Sub-classes -----------------------
public static final class AES extends UBSecretKeyCipher
{
public AES() { super(ObjectType.AES); }
}
public static final class DES3 extends UBSecretKeyCipher
{
public DES3() { super(ObjectType.DES3); }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy