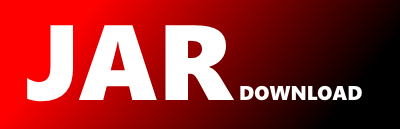
java-ec.com.unbound.common.crypto.ec.Point Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.common.crypto.ec;
import com.unbound.common.crypto.ec.math.UInt;
import java.math.BigInteger;
import java.security.spec.ECPoint;
public final class Point
{
final Curve curve;
final int[] x = new int[Curve.MAX_LEN];
final int[] y = new int[Curve.MAX_LEN];
final int[] z = new int[Curve.MAX_LEN];
Point()
{
this.curve = null;
}
Point(Curve curve)
{
this.curve = curve;
}
public Point(Curve curve, BigInteger xx, BigInteger yy)
{
this.curve = curve;
int[] x = curve.alloc(); UInt.fromBigInteger(curve.length, x, xx);
int[] y = curve.alloc(); UInt.fromBigInteger(curve.length, y, yy);
curve.init(this, x, y);
}
Point(Curve curve, int[] x, int[] y)
{
this.curve = curve;
curve.init(this, x, y);
}
BigInteger getX()
{
int[] temp = curve.alloc();
curve.getCoord(this, temp, null);
return UInt.toBigInteger(curve.length, temp);
}
BigInteger getY()
{
int[] temp = curve.alloc();
curve.getCoord(this, null, temp);
return UInt.toBigInteger(curve.length, temp);
}
byte[] toOct()
{
int l = toOct(null, 0);
byte[] out = new byte[l];
toOct(out, 0);
return out;
}
byte[] toCompressedOct()
{
int l = toCompressedOct(null, 0);
byte[] out = new byte[l];
toCompressedOct(out, 0);
return out;
}
int toOct(byte[] out, int offset)
{
int bytes = curve.getBytes();
if (out!=null) curve.toOct(this, out, offset);
return 1 + bytes*2;
}
int toCompressedOct(byte[] out, int offset)
{
int bytes = curve.getBytes();
if (out!=null) curve.toCompressedOct(this, out, offset);
return 1 + bytes;
}
boolean isInfinity()
{
return 0 != UInt.isZero(curve.length, z);
}
boolean isOnCurve()
{
return curve.isOnCurve(this);
}
@Override
public boolean equals(Object other)
{
if (this==other) return true;
if (other==null) return false;
if ((other instanceof Point))
{
return curve.equ(this, ((Point)other));
}
if (other instanceof ECPoint)
{
return curve.equ(this, (ECPoint)other);
}
return false;
}
public Point dbl()
{
Point r = new Point(curve);
curve.dbl(r, this, null);
return r;
}
public Point add(Point p)
{
Point r = new Point(curve);
curve.add(r, this, p, null);
return r;
}
public Point mul(BigInteger xx)
{
int[] x = curve.alloc();
UInt.fromBigInteger(curve.length, x, xx);
return mul(x);
}
Point mul(int[] x)
{
Point r = new Point(curve);
curve.mul(r, this, x);
return r;
}
static void copy(Point r, Point p)
{
System.arraycopy(p.x, 0, r.x, 0, Curve.MAX_LEN);
System.arraycopy(p.y, 0, r.y, 0, Curve.MAX_LEN);
System.arraycopy(p.z, 0, r.z, 0, Curve.MAX_LEN);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy