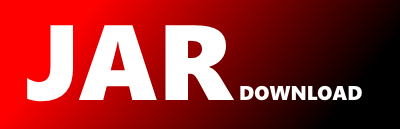
java-ec.com.unbound.common.crypto.ec.SecP384R1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.common.crypto.ec;
public class SecP384R1 extends Curve
{
private static final long P0 = 0xffffffffL;
private static final long P1 = 0x00000000L;
private static final long P2 = 0x00000000L;
private static final long P3 = 0xffffffffL;
private static final long P4 = 0xfffffffeL;
private static final long P5 = 0xffffffffL;
private static final long P6 = 0xffffffffL;
private static final long P7 = 0xffffffffL;
private static final long P8 = 0xffffffffL;
private static final long P9 = 0xffffffffL;
private static final long P10 = 0xffffffffL;
private static final long P11 = 0xffffffffL;
private static final int[] P = {(int)P0, (int)P1, (int)P2, (int)P3, (int)P4, (int)P5, (int)P6, (int)P7, (int)P8, (int)P9, (int)P10, (int)P11};
private static final int[] MONT_ONE = {0x00000001, 0xffffffff, 0xffffffff, 0x00000000, 0x00000001, 0x00000000, 0x00000000, 0x00000000, 0x00000000, 0x00000000, 0x00000000, 0x00000000};
private static final int[] MONT_RR = {0x00000001, 0xfffffffe, 0x00000000, 0x00000002, 0x00000000, 0xfffffffe, 0x00000000, 0x00000002, 0x00000001, 0x00000000, 0x00000000, 0x00000000};
private static final int[] B = {0xd3ec2aef, 0x2a85c8ed, 0x8a2ed19d, 0xc656398d, 0x5013875a, 0x0314088f, 0xfe814112, 0x181d9c6e, 0xe3f82d19, 0x988e056b, 0xe23ee7e4, 0xb3312fa7};
private static final int[] Gx = {0x72760ab7, 0x3a545e38, 0xbf55296c, 0x5502f25d, 0x82542a38, 0x59f741e0, 0x8ba79b98, 0x6e1d3b62, 0xf320ad74, 0x8eb1c71e, 0xbe8b0537, 0xaa87ca22};
private static final int[] Gy = {0x90ea0e5f, 0x7a431d7c, 0x1d7e819d, 0x0a60b1ce, 0xb5f0b8c0, 0xe9da3113, 0x289a147c, 0xf8f41dbd, 0x9292dc29, 0x5d9e98bf, 0x96262c6f, 0x3617de4a};
private static final int[] q = {0xccc52973, 0xecec196a, 0x48b0a77a, 0x581a0db2, 0xf4372ddf, 0xc7634d81, 0xffffffff, 0xffffffff, 0xffffffff, 0xffffffff, 0xffffffff, 0xffffffff};
protected SecP384R1()
{
super(384, P, 1, MONT_ONE, MONT_RR, -3, B, Gx, Gy, q);
}
private static SecP384R1 instance = null;
private static Point infinityInstance = null;
static SecP384R1 getInstance()
{
if (instance==null) instance = new SecP384R1();
return instance;
}
@Override
public Point infinity()
{
if (infinityInstance==null) infinityInstance = new Point(getInstance());
return infinityInstance;
}
@Override
protected void add(int[] r, int[] x, int[] y, IntPool pool)
{
//int[] temp = IntPool.alloc(pool, IntPool.BASE+0);//alloc();
//int carry = UInt256.add(temp, x, y);
int t0, t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11;
int r0, r1, r2, r3, r4, r5, r6, r7, r8, r9, r10, r11;
long c, b;
c = (x[ 0] & 0xffffffffL) +(y[ 0] & 0xffffffffL); t0 = (int)c; c >>>= 32; b = (t0 & 0xffffffffL) - 0xffffffffL; r0 = (int)b; b >>= 32;
c += (x[ 1] & 0xffffffffL) +(y[ 1] & 0xffffffffL); t1 = (int)c; c >>>= 32; b += (t1 & 0xffffffffL); r1 = (int)b; b >>= 32;
c += (x[ 2] & 0xffffffffL) +(y[ 2] & 0xffffffffL); t2 = (int)c; c >>>= 32; b += (t2 & 0xffffffffL); r2 = (int)b; b >>= 32;
c += (x[ 3] & 0xffffffffL) +(y[ 3] & 0xffffffffL); t3 = (int)c; c >>>= 32; b += (t3 & 0xffffffffL) - 0xffffffffL; r3 = (int)b; b >>= 32;
c += (x[ 4] & 0xffffffffL) +(y[ 4] & 0xffffffffL); t4 = (int)c; c >>>= 32; b += (t4 & 0xffffffffL) - 0xfffffffeL; r4 = (int)b; b >>= 32;
c += (x[ 5] & 0xffffffffL) +(y[ 5] & 0xffffffffL); t5 = (int)c; c >>>= 32; b += (t5 & 0xffffffffL) - 0xffffffffL; r5 = (int)b; b >>= 32;
c += (x[ 6] & 0xffffffffL) +(y[ 6] & 0xffffffffL); t6 = (int)c; c >>>= 32; b += (t6 & 0xffffffffL) - 0xffffffffL; r6 = (int)b; b >>= 32;
c += (x[ 7] & 0xffffffffL) +(y[ 7] & 0xffffffffL); t7 = (int)c; c >>>= 32; b += (t7 & 0xffffffffL) - 0xffffffffL; r7 = (int)b; b >>= 32;
c += (x[ 8] & 0xffffffffL) +(y[ 8] & 0xffffffffL); t8 = (int)c; c >>>= 32; b += (t8 & 0xffffffffL) - 0xffffffffL; r8 = (int)b; b >>= 32;
c += (x[ 9] & 0xffffffffL) +(y[ 9] & 0xffffffffL); t9 = (int)c; c >>>= 32; b += (t9 & 0xffffffffL) - 0xffffffffL; r9 = (int)b; b >>= 32;
c += (x[10] & 0xffffffffL) +(y[10] & 0xffffffffL); t10 = (int)c; c >>>= 32; b += (t10 & 0xffffffffL) - 0xffffffffL; r10 = (int)b; b >>= 32;
c += (x[11] & 0xffffffffL) +(y[11] & 0xffffffffL); t11 = (int)c; c >>>= 32; b += (t11 & 0xffffffffL) - 0xffffffffL; r11 = (int)b; b >>= 32;
int carry = (int)c;
int borrow = (int)b;// & 1;
//int borrow = subP(r, temp);
//cmov(r, ~carry & borrow, temp);
int cond = ~carry & borrow;
cond = -(cond & 1);
r[ 0] = ((r0 ^ t0) & cond) ^ r0;
r[ 1] = ((r1 ^ t1) & cond) ^ r1;
r[ 2] = ((r2 ^ t2) & cond) ^ r2;
r[ 3] = ((r3 ^ t3) & cond) ^ r3;
r[ 4] = ((r4 ^ t4) & cond) ^ r4;
r[ 5] = ((r5 ^ t5) & cond) ^ r5;
r[ 6] = ((r6 ^ t6) & cond) ^ r6;
r[ 7] = ((r7 ^ t7) & cond) ^ r7;
r[ 8] = ((r8 ^ t8) & cond) ^ r8;
r[ 9] = ((r9 ^ t9) & cond) ^ r9;
r[10] = ((r10 ^ t10) & cond) ^ r10;
r[11] = ((r11 ^ t11) & cond) ^ r11;
}
@Override
protected void sub(int[] r, int[] x, int[] y, IntPool pool)
{
int t0, t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11;
int r0, r1, r2, r3, r4, r5, r6, r7, r8, r9, r10, r11;
long c, b;
b = (x[ 0] & 0xffffffffL) - (y[ 0] & 0xffffffffL); t0 = (int)b; b >>= 32; c = (t0 & 0xffffffffL) + 0xffffffffL; r0 = (int)c; c >>>= 32;
b += (x[ 1] & 0xffffffffL) - (y[ 1] & 0xffffffffL); t1 = (int)b; b >>= 32; c += (t1 & 0xffffffffL); r1 = (int)c; c >>>= 32;
b += (x[ 2] & 0xffffffffL) - (y[ 2] & 0xffffffffL); t2 = (int)b; b >>= 32; c += (t2 & 0xffffffffL); r2 = (int)c; c >>>= 32;
b += (x[ 3] & 0xffffffffL) - (y[ 3] & 0xffffffffL); t3 = (int)b; b >>= 32; c += (t3 & 0xffffffffL) + 0xffffffffL; r3 = (int)c; c >>>= 32;
b += (x[ 4] & 0xffffffffL) - (y[ 4] & 0xffffffffL); t4 = (int)b; b >>= 32; c += (t4 & 0xffffffffL) + 0xfffffffeL; r4 = (int)c; c >>>= 32;
b += (x[ 5] & 0xffffffffL) - (y[ 5] & 0xffffffffL); t5 = (int)b; b >>= 32; c += (t5 & 0xffffffffL) + 0xffffffffL; r5 = (int)c; c >>>= 32;
b += (x[ 6] & 0xffffffffL) - (y[ 6] & 0xffffffffL); t6 = (int)b; b >>= 32; c += (t6 & 0xffffffffL) + 0xffffffffL; r6 = (int)c; c >>>= 32;
b += (x[ 7] & 0xffffffffL) - (y[ 7] & 0xffffffffL); t7 = (int)b; b >>= 32; c += (t7 & 0xffffffffL) + 0xffffffffL; r7 = (int)c; c >>>= 32;
b += (x[ 8] & 0xffffffffL) - (y[ 8] & 0xffffffffL); t8 = (int)b; b >>= 32; c += (t8 & 0xffffffffL) + 0xffffffffL; r8 = (int)c; c >>>= 32;
b += (x[ 9] & 0xffffffffL) - (y[ 9] & 0xffffffffL); t9 = (int)b; b >>= 32; c += (t9 & 0xffffffffL) + 0xffffffffL; r9 = (int)c; c >>>= 32;
b += (x[10] & 0xffffffffL) - (y[10] & 0xffffffffL); t10 = (int)b; b >>= 32; c += (t10 & 0xffffffffL) + 0xffffffffL; r10 = (int)c; c >>>= 32;
b += (x[11] & 0xffffffffL) - (y[11] & 0xffffffffL); t11 = (int)b; b >>= 32; c += (t11 & 0xffffffffL) + 0xffffffffL; r11 = (int)c;
int borrow = (int)b & 1;
int cond = borrow-1;
r[ 0] = ((r0 ^ t0 ) & cond) ^ r0;
r[ 1] = ((r1 ^ t1 ) & cond) ^ r1;
r[ 2] = ((r2 ^ t2 ) & cond) ^ r2;
r[ 3] = ((r3 ^ t3 ) & cond) ^ r3;
r[ 4] = ((r4 ^ t4 ) & cond) ^ r4;
r[ 5] = ((r5 ^ t5 ) & cond) ^ r5;
r[ 6] = ((r6 ^ t6 ) & cond) ^ r6;
r[ 7] = ((r7 ^ t7 ) & cond) ^ r7;
r[ 8] = ((r8 ^ t8 ) & cond) ^ r8;
r[ 9] = ((r9 ^ t9 ) & cond) ^ r9;
r[10] = ((r10 ^ t10) & cond) ^ r10;
r[11] = ((r11 ^ t11) & cond) ^ r11;
}
@Override
protected void prepareMontMul(long m, long[] mp)
{
long c = -m;
c = (c & 0xffffffffL) | ((m + (c>>32)) << 32);
mp[0]=c;
mp[1]=0;
mp[2]=0;
mp[3]=c;
mp[4]=m * 0xfffffffeL;
mp[5]=c;
mp[6]=c;
mp[7]=c;
mp[8]=c;
mp[9]=c;
mp[10]=c;
mp[11]=c;
//for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy