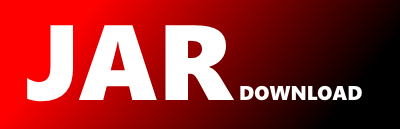
java-ec.com.unbound.common.crypto.ec.math.Comba Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.common.crypto.ec.math;
public class Comba
{
private static final long LMASK = 0xffffffffL;
private static long U64(int x) { return x & LMASK; }
private static long LO(long x) { return x & LMASK; }
private static long HI(long x) { return x >>> 32; }
private static final class Triple
{
long w0=0, w1=0, w2=0;
private int shift()
{
int res = (int)w0;
w0 = w1; w1 = w2; w2 = 0;
return res;
}
void muladd(int x, int y)
{
long m = U64(x) * U64(y);
long mLo = LO(m);
long mHi = HI(m);
long carry;
w0 += mLo; carry = HI(w0); w0 &= LMASK;
w1 += mHi+carry; carry = HI(w1); w1 &= LMASK;
w2 += carry; w2 &= LMASK;
}
void muladd_2(int x, int y)
{
long m = U64(x) * U64(y);
long mLo = LO(m);
long mHi = HI(m);
long carry;
long t0 = w0 + mLo; carry = HI(t0); t0 &= LMASK;
long t1 = w1 + mHi + carry; carry = HI(t1); t1 &= LMASK;
long carry2;
w0 = t0 + mLo; carry2 = HI(w0); w0 &= LMASK;
w1 = t1 + mHi + carry2; carry2 = HI(w1); w1 &= LMASK;
w2 = w2 + carry + carry2; w2 &= LMASK;
}
}
//8x8 Squaring
public static void sqr8(int[] r, int[] x) // r[16], x[8]
{
Triple w = new Triple();
w.muladd (x[ 0], x[ 0]);
r[ 0] = w.shift();
w.muladd_2(x[ 0], x[ 1]);
r[ 1] = w.shift();
w.muladd_2(x[ 0], x[ 2]);
w.muladd (x[ 1], x[ 1]);
r[ 2] = w.shift();
w.muladd_2(x[ 0], x[ 3]);
w.muladd_2(x[ 1], x[ 2]);
r[ 3] = w.shift();
w.muladd_2(x[ 0], x[ 4]);
w.muladd_2(x[ 1], x[ 3]);
w.muladd (x[ 2], x[ 2]);
r[ 4] = w.shift();
w.muladd_2(x[ 0], x[ 5]);
w.muladd_2(x[ 1], x[ 4]);
w.muladd_2(x[ 2], x[ 3]);
r[ 5] = w.shift();
w.muladd_2(x[ 0], x[ 6]);
w.muladd_2(x[ 1], x[ 5]);
w.muladd_2(x[ 2], x[ 4]);
w.muladd (x[ 3], x[ 3]);
r[ 6] = w.shift();
w.muladd_2(x[ 0], x[ 7]);
w.muladd_2(x[ 1], x[ 6]);
w.muladd_2(x[ 2], x[ 5]);
w.muladd_2(x[ 3], x[ 4]);
r[ 7] = w.shift();
w.muladd_2(x[ 1], x[ 7]);
w.muladd_2(x[ 2], x[ 6]);
w.muladd_2(x[ 3], x[ 5]);
w.muladd (x[ 4], x[ 4]);
r[ 8] = w.shift();
w.muladd_2(x[ 2], x[ 7]);
w.muladd_2(x[ 3], x[ 6]);
w.muladd_2(x[ 4], x[ 5]);
r[ 9] = w.shift();
w.muladd_2(x[ 3], x[ 7]);
w.muladd_2(x[ 4], x[ 6]);
w.muladd (x[ 5], x[ 5]);
r[10] = w.shift();
w.muladd_2(x[ 4], x[ 7]);
w.muladd_2(x[ 5], x[ 6]);
r[11] = w.shift();
w.muladd_2(x[ 5], x[ 7]);
w.muladd (x[ 6], x[ 6]);
r[12] = w.shift();
w.muladd_2(x[ 6], x[ 7]);
r[13] = w.shift();
w.muladd (x[ 7], x[ 7]);
r[14] = (int)w.w0;
r[15] = (int)w.w1;
}
// 8x8 Multiplication
public static void mul8(int[] r, int[] x, int[] y) // r[16], x[8], y[8]
{
Triple w = new Triple();
w.muladd(x[ 0], y[ 0]);
r[ 0] = w.shift();
w.muladd(x[ 0], y[ 1]);
w.muladd(x[ 1], y[ 0]);
r[ 1] = w.shift();
w.muladd(x[ 0], y[ 2]);
w.muladd(x[ 1], y[ 1]);
w.muladd(x[ 2], y[ 0]);
r[ 2] = w.shift();
w.muladd(x[ 0], y[ 3]);
w.muladd(x[ 1], y[ 2]);
w.muladd(x[ 2], y[ 1]);
w.muladd(x[ 3], y[ 0]);
r[ 3] = w.shift();
w.muladd(x[ 0], y[ 4]);
w.muladd(x[ 1], y[ 3]);
w.muladd(x[ 2], y[ 2]);
w.muladd(x[ 3], y[ 1]);
w.muladd(x[ 4], y[ 0]);
r[ 4] = w.shift();
w.muladd(x[ 0], y[ 5]);
w.muladd(x[ 1], y[ 4]);
w.muladd(x[ 2], y[ 3]);
w.muladd(x[ 3], y[ 2]);
w.muladd(x[ 4], y[ 1]);
w.muladd(x[ 5], y[ 0]);
r[ 5] = w.shift();
w.muladd(x[ 0], y[ 6]);
w.muladd(x[ 1], y[ 5]);
w.muladd(x[ 2], y[ 4]);
w.muladd(x[ 3], y[ 3]);
w.muladd(x[ 4], y[ 2]);
w.muladd(x[ 5], y[ 1]);
w.muladd(x[ 6], y[ 0]);
r[ 6] = w.shift();
w.muladd(x[ 0], y[ 7]);
w.muladd(x[ 1], y[ 6]);
w.muladd(x[ 2], y[ 5]);
w.muladd(x[ 3], y[ 4]);
w.muladd(x[ 4], y[ 3]);
w.muladd(x[ 5], y[ 2]);
w.muladd(x[ 6], y[ 1]);
w.muladd(x[ 7], y[ 0]);
r[ 7] = w.shift();
w.muladd(x[ 1], y[ 7]);
w.muladd(x[ 2], y[ 6]);
w.muladd(x[ 3], y[ 5]);
w.muladd(x[ 4], y[ 4]);
w.muladd(x[ 5], y[ 3]);
w.muladd(x[ 6], y[ 2]);
w.muladd(x[ 7], y[ 1]);
r[ 8] = w.shift();
w.muladd(x[ 2], y[ 7]);
w.muladd(x[ 3], y[ 6]);
w.muladd(x[ 4], y[ 5]);
w.muladd(x[ 5], y[ 4]);
w.muladd(x[ 6], y[ 3]);
w.muladd(x[ 7], y[ 2]);
r[ 9] = w.shift();
w.muladd(x[ 3], y[ 7]);
w.muladd(x[ 4], y[ 6]);
w.muladd(x[ 5], y[ 5]);
w.muladd(x[ 6], y[ 4]);
w.muladd(x[ 7], y[ 3]);
r[10] = w.shift();
w.muladd(x[ 4], y[ 7]);
w.muladd(x[ 5], y[ 6]);
w.muladd(x[ 6], y[ 5]);
w.muladd(x[ 7], y[ 4]);
r[11] = w.shift();
w.muladd(x[ 5], y[ 7]);
w.muladd(x[ 6], y[ 6]);
w.muladd(x[ 7], y[ 5]);
r[12] = w.shift();
w.muladd(x[ 6], y[ 7]);
w.muladd(x[ 7], y[ 6]);
r[13] = w.shift();
w.muladd(x[ 7], y[ 7]);
r[14] = (int)w.w0;
r[15] = (int)w.w1;
}
/*
static void fromBigInteger(int[] dst, BigInteger x)
{
byte[] bin = x.toByteArray();
if (bin.length
© 2015 - 2025 Weber Informatics LLC | Privacy Policy