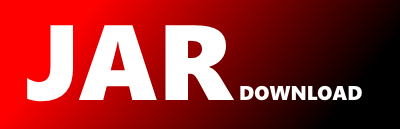
java-ec.com.unbound.common.crypto.ec.math.UInt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.common.crypto.ec.math;
import java.math.BigInteger;
public abstract class UInt
{
public static void toBytes(int length, int[] x, byte[] out, int offset, int bytes)
{
for (int i=0; i> 8 ) & 0xff);
if (bytes==0) break; bytes--; out[offset+bytes] = (byte)((v >> 16) & 0xff);
if (bytes==0) break; bytes--; out[offset+bytes] = (byte)((v >> 24) & 0xff);
}
for (int i=0; ilength*4)
{
while (bytes>0 && bin[offset]==0) { bytes--; offset++; }
}
for (int i=0; i>> shift) | (x[i+1]<<(32-shift));
r[length-1] = x[length-1] >>> shift;
}
public static int add(int length, int[] r, int[] x)
{
return add(length, r, r, x, 0);
}
public static int add(int length, int[] r, int[] x, int[] y, int carry)
{
long c = carry;
for (int i=0; i>>= 32;
}
return (int)c;
}
public static int add(int length, int[] r, int[] x, int[] y)
{
return add(length, r, x, y, 0);
}
public static int add(int length, int[] r, int y)
{
return add(length, r, r, y);
}
public static int add(int length, int[] r, int[] x, int y)
{
long c = (x[0] & 0xffffffffL) + (y & 0xffffffffL); r[0] = (int)c; c >>>= 32;
for (int i=1; i>>= 32;
}
return (int)c;
}
public static int sub(int length, int[] r, int[] x)
{
return sub(length, r, r, x);
}
public static int sub(int length, int[] r, int[] x, int[] y)
{
return sub(length, r, x, y, 0);
}
public static int sub(int length, int[] r, int[] x, int[] y, int carry)
{
long c = carry;
for (int i=0; i>= 32;
}
return (int)c & 1;
}
public static int sub(int length, int[] r, int y)
{
return sub(length, r, r, y);
}
public static int sub(int length, int[] r, int[] x, int y)
{
long c = 0;
c += (x[0] & 0xffffffffL) - (y & 0xffffffffL);
r[0] = (int)c;
c >>= 32;
for (int i=1; i>= 32;
}
return (int)c & 1;
}
public static boolean equ(int length, int[] x, int[] y)
{
int c = 0;
for (int i=0; i>> 32) + (lo >>> 32);
r[i] = (int)lo;
}
return (int)carry;
}
private static int addmul(int length, int[] r, int offset, int[] a, int b)
{
long carry = 0;
long bb = b & 0xffffffffL;
for (int i=0; i>>= 32) + (lo >>> 32);
lo = (lo & 0xffffffffL) + (r[offset+i] & 0xffffffffL);
carry += (lo >>> 32);
r[offset+i] = (int)lo;
}
return (int)carry;
}
public static void mul(int length, int[] r, int[] x, int[] y)
{
r[length] = mul(length, r, x, y[0]);
for (int i=1; i> 1
int[] a = alloc(length); copy(length, a, x); // a = x
int[] b = alloc(length); copy(length, b, p); // b = p
int[] u = alloc(length); setInt(length, u, 1); // u = 1
int[] v = r; setInt(length, v, 0); // v = 0
int[] temp = alloc(length);
for (int i=0; i>> 32;
A >>>= 32;
for (int index=1; index>> 32;
x = (x & 0xffffffffL) + (rr[index] & 0xffffffffL);
xHi += x >>> 32;
x = A + (x & 0xffffffffL);
A = xHi + (x >>> 32);
x2 = m * (p[index] & 0xffffffffL);
xHi = x2 >>> 32;
x = (x & 0xffffffffL) + (x2 & 0xffffffffL);
xHi += x >>> 32;
x = B + (x & 0xffffffffL);
B = xHi + (x >>> 32);
rr[index-1] = (int)x;
}
B += ((A + rs) & 0xffffffffL);
rs = (A >>> 32) + (B >>> 32);
rr[length-1] = (int)B;
}
int cond = (int)rs;
int[] r_minus_p = temp_r_minus_p;
if (r_minus_p==null) r_minus_p = alloc(length);
int borrow = sub(length, r_minus_p, rr, p);
cond |= 1-borrow;
cond |= isZero(length, r_minus_p);
cmov(length, r, cond, rr, r_minus_p);
}
/*
public static void main(String[] args)
{
int length = 8;
BigInteger pp = SunEC.getSecP256r1().p;
int[] p = alloc(length); fromBigInteger(length, p, pp);
int[] x = alloc(length); for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy