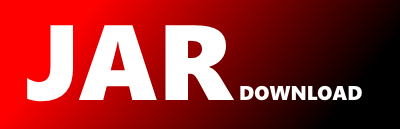
java-ec.com.unbound.common.crypto.ec.math.UInt384 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unbound-java-provider Show documentation
Show all versions of unbound-java-provider Show documentation
This is a collection of JAVA libraries that implement Unbound cryptographic classes for JAVA provider, PKCS11 wrapper, cryptoki, and advapi
package com.unbound.common.crypto.ec.math;
public abstract class UInt384
{
public static final int[] ONE = {1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0};
private static final long LM = 0xffffffffL;
private static long U64(int x) { return x & LM; }
private static long LO(long x) { return x & LM; }
private static long HI(long x) { return x >>> 32; }
public static void copy(int[] r, int[] x)
{
r[0] = x[0]; r[1] = x[1]; r[ 2] = x[ 2]; r[ 3] = x[ 3];
r[4] = x[4]; r[5] = x[5]; r[ 6] = x[ 6]; r[ 7] = x[ 7];
r[8] = x[8]; r[9] = x[9]; r[10] = x[10]; r[11] = x[11];
}
public static void cmov(int[] r, int cond, int[] x) { cmov(r, cond, r, x); } // r = cond ? x : r
public static void cmov(int[] r, int cond, int[] x, int[] y) // r = cond ? y : x
{
cond = -(cond & 1);
r[ 0] = ((x[ 0] ^ y[ 0]) & cond) ^ x[ 0];
r[ 1] = ((x[ 1] ^ y[ 1]) & cond) ^ x[ 1];
r[ 2] = ((x[ 2] ^ y[ 2]) & cond) ^ x[ 2];
r[ 3] = ((x[ 3] ^ y[ 3]) & cond) ^ x[ 3];
r[ 4] = ((x[ 4] ^ y[ 4]) & cond) ^ x[ 4];
r[ 5] = ((x[ 5] ^ y[ 5]) & cond) ^ x[ 5];
r[ 6] = ((x[ 6] ^ y[ 6]) & cond) ^ x[ 6];
r[ 7] = ((x[ 7] ^ y[ 7]) & cond) ^ x[ 7];
r[ 8] = ((x[ 8] ^ y[ 8]) & cond) ^ x[ 8];
r[ 9] = ((x[ 9] ^ y[ 9]) & cond) ^ x[ 9];
r[10] = ((x[10] ^ y[10]) & cond) ^ x[10];
r[11] = ((x[11] ^ y[11]) & cond) ^ x[11];
}
public static void shr(int[] r, int[] x, int shift)
{
r[ 0] = (x[ 0] >>> shift) | (x[ 1]<<(32-shift));
r[ 1] = (x[ 1] >>> shift) | (x[ 2]<<(32-shift));
r[ 2] = (x[ 2] >>> shift) | (x[ 3]<<(32-shift));
r[ 3] = (x[ 3] >>> shift) | (x[ 4]<<(32-shift));
r[ 4] = (x[ 4] >>> shift) | (x[ 5]<<(32-shift));
r[ 5] = (x[ 5] >>> shift) | (x[ 6]<<(32-shift));
r[ 6] = (x[ 6] >>> shift) | (x[ 7]<<(32-shift));
r[ 7] = (x[ 7] >>> shift) | (x[ 8]<<(32-shift));
r[ 8] = (x[ 8] >>> shift) | (x[ 9]<<(32-shift));
r[ 9] = (x[ 9] >>> shift) | (x[10]<<(32-shift));
r[10] = (x[10] >>> shift) | (x[11]<<(32-shift));
r[11] = x[11] >>> shift;
}
public static int add(int[] r, int[] x, int[] y, int carry)
{
long c = carry;
c += (x[ 0] & 0xffffffffL) + (y[ 0] & 0xffffffffL); r[ 0] = (int)c; c >>>= 32;
c += (x[ 1] & 0xffffffffL) + (y[ 1] & 0xffffffffL); r[ 1] = (int)c; c >>>= 32;
c += (x[ 2] & 0xffffffffL) + (y[ 2] & 0xffffffffL); r[ 2] = (int)c; c >>>= 32;
c += (x[ 3] & 0xffffffffL) + (y[ 3] & 0xffffffffL); r[ 3] = (int)c; c >>>= 32;
c += (x[ 4] & 0xffffffffL) + (y[ 4] & 0xffffffffL); r[ 4] = (int)c; c >>>= 32;
c += (x[ 5] & 0xffffffffL) + (y[ 5] & 0xffffffffL); r[ 5] = (int)c; c >>>= 32;
c += (x[ 6] & 0xffffffffL) + (y[ 6] & 0xffffffffL); r[ 6] = (int)c; c >>>= 32;
c += (x[ 7] & 0xffffffffL) + (y[ 7] & 0xffffffffL); r[ 7] = (int)c; c >>>= 32;
c += (x[ 8] & 0xffffffffL) + (y[ 8] & 0xffffffffL); r[ 8] = (int)c; c >>>= 32;
c += (x[ 9] & 0xffffffffL) + (y[ 9] & 0xffffffffL); r[ 9] = (int)c; c >>>= 32;
c += (x[10] & 0xffffffffL) + (y[10] & 0xffffffffL); r[10] = (int)c; c >>>= 32;
c += (x[11] & 0xffffffffL) + (y[11] & 0xffffffffL); r[11] = (int)c; c >>>= 32;
return (int)c;
}
public static int add(int[] r, int[] x, int[] y)
{
return add(r, x, y, 0);
}
public static int add(int[] r, int[] x, int y)
{
long c = 0;
c += (x[ 0] & 0xffffffffL) + (y& 0xffffffffL); r[ 0] = (int)c; c >>>= 32;
c += (x[ 1] & 0xffffffffL); r[ 1] = (int)c; c >>>= 32;
c += (x[ 2] & 0xffffffffL); r[ 2] = (int)c; c >>>= 32;
c += (x[ 3] & 0xffffffffL); r[ 3] = (int)c; c >>>= 32;
c += (x[ 4] & 0xffffffffL); r[ 4] = (int)c; c >>>= 32;
c += (x[ 5] & 0xffffffffL); r[ 5] = (int)c; c >>>= 32;
c += (x[ 6] & 0xffffffffL); r[ 6] = (int)c; c >>>= 32;
c += (x[ 7] & 0xffffffffL); r[ 7] = (int)c; c >>>= 32;
c += (x[ 8] & 0xffffffffL); r[ 8] = (int)c; c >>>= 32;
c += (x[ 9] & 0xffffffffL); r[ 9] = (int)c; c >>>= 32;
c += (x[10] & 0xffffffffL); r[10] = (int)c; c >>>= 32;
c += (x[11] & 0xffffffffL); r[11] = (int)c; c >>>= 32;
return (int)c;
}
public static int sub(int[] r, int[] x)
{
return sub(r, r, x);
}
public static int sub(int[] r, int[] x, int[] y)
{
long c = 0;
c += (x[ 0] & 0xffffffffL) - (y[ 0] & 0xffffffffL); r[ 0] = (int)c; c >>= 32;
c += (x[ 1] & 0xffffffffL) - (y[ 1] & 0xffffffffL); r[ 1] = (int)c; c >>= 32;
c += (x[ 2] & 0xffffffffL) - (y[ 2] & 0xffffffffL); r[ 2] = (int)c; c >>= 32;
c += (x[ 3] & 0xffffffffL) - (y[ 3] & 0xffffffffL); r[ 3] = (int)c; c >>= 32;
c += (x[ 4] & 0xffffffffL) - (y[ 4] & 0xffffffffL); r[ 4] = (int)c; c >>= 32;
c += (x[ 5] & 0xffffffffL) - (y[ 5] & 0xffffffffL); r[ 5] = (int)c; c >>= 32;
c += (x[ 6] & 0xffffffffL) - (y[ 6] & 0xffffffffL); r[ 6] = (int)c; c >>= 32;
c += (x[ 7] & 0xffffffffL) - (y[ 7] & 0xffffffffL); r[ 7] = (int)c; c >>= 32;
c += (x[ 8] & 0xffffffffL) - (y[ 8] & 0xffffffffL); r[ 8] = (int)c; c >>= 32;
c += (x[ 9] & 0xffffffffL) - (y[ 9] & 0xffffffffL); r[ 9] = (int)c; c >>= 32;
c += (x[10] & 0xffffffffL) - (y[10] & 0xffffffffL); r[10] = (int)c; c >>= 32;
c += (x[11] & 0xffffffffL) - (y[11] & 0xffffffffL); r[11] = (int)c; c >>= 32;
return (int)c & 1;
}
public static boolean equ(int[] x, int[] y)
{
int c = 0;
c |= (x[ 0] ^ y[ 0]);
c |= (x[ 1] ^ y[ 1]);
c |= (x[ 2] ^ y[ 2]);
c |= (x[ 3] ^ y[ 3]);
c |= (x[ 4] ^ y[ 4]);
c |= (x[ 5] ^ y[ 5]);
c |= (x[ 6] ^ y[ 6]);
c |= (x[ 7] ^ y[ 7]);
c |= (x[ 8] ^ y[ 8]);
c |= (x[ 9] ^ y[ 9]);
c |= (x[10] ^ y[10]);
c |= (x[11] ^ y[11]);
return c==0;
}
public static void setZero(int[] x)
{
setInt(x, 0);
}
public static void setInt(int[] x, int y)
{
x[ 0] = y; x[ 1] = 0; x[ 2] = 0; x[ 3] = 0;
x[ 4] = 0; x[ 5] = 0; x[ 6] = 0; x[ 7] = 0;
x[ 8] = 0; x[ 9] = 0; x[10] = 0; x[11] = 0;
}
public static int isZero(int[] x)
{
return isInt(x, 0);
}
public static int isInt(int[] x, int y)
{
int r = 0;
r |= x[ 0] ^ y; r |= x[ 1]; r |= x[ 2]; r |= x[ 3];
r |= x[ 4]; r |= x[ 5]; r |= x[ 6]; r |= x[ 7];
r |= x[ 8]; r |= x[ 9]; r |= x[10]; r |= x[11];
return (r==0) ? -1 : 0;
}
//public static void mul(int[] r, int[] x, int[] y) { Comba.mul8(r, x, y); }
//public static void sqr(int[] r, int[] x) { Comba.sqr8(r, x); }
public static int[] alloc() { return new int[12]; }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy