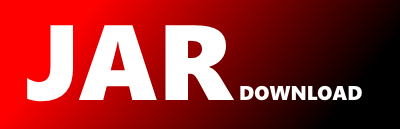
uncle.proxy.ProxyChain Maven / Gradle / Ivy
package uncle.proxy;
import net.sf.cglib.proxy.MethodProxy;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
/**
* Created by hujianbo on 2018/1/19.
*/
public class ProxyChain {
private final Class> targetClass;
private final Object targetObject;
private final Method targetMethod;
private final MethodProxy methodProxy;
private final Object[] methodParams;
private List proxyList = new ArrayList();
private int proxyIndex = 0;
public ProxyChain(Class> targetClass, Object targetObject, Method targetMethod, MethodProxy methodProxy, Object[] methodParams, List proxyList) {
this.targetClass = targetClass;
this.targetObject = targetObject;
this.targetMethod = targetMethod;
this.methodProxy = methodProxy;
this.methodParams = methodParams;
this.proxyList = proxyList;
}
public Class> getTargetClass() {
return targetClass;
}
public Method getTargetMethod() {
return targetMethod;
}
public Object[] getMethodParams() {
return methodParams;
}
public Object doProxyChain() throws Throwable {
Object methodResult;
if (proxyIndex < proxyList.size()) {
methodResult = proxyList.get(proxyIndex++).doProxy(this);
} else {
methodResult = methodProxy.invokeSuper(targetObject, methodParams);
}
return methodResult;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy