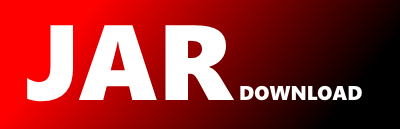
org.unipop.schema.property.CoalescePropertySchema Maven / Gradle / Ivy
package org.unipop.schema.property;
import org.apache.tinkerpop.gremlin.process.traversal.step.util.HasContainer;
import org.json.JSONArray;
import org.json.JSONObject;
import org.unipop.query.predicates.PredicatesHolder;
import org.unipop.query.predicates.PredicatesHolderFactory;
import org.unipop.schema.property.type.PropertyType;
import org.unipop.util.PropertySchemaFactory;
import java.util.*;
/**
* Created by sbarzilay on 8/8/16.
*/
public class CoalescePropertySchema implements ParentSchemaProperty {
protected final String key;
protected final List children;
public CoalescePropertySchema(String key, List schemas) {
this.key = key;
this.children = schemas;
}
@Override
public Collection getChildren() {
return children;
}
@Override
public String getKey() {
return key;
}
@Override
public Map toProperties(Map source) {
Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy