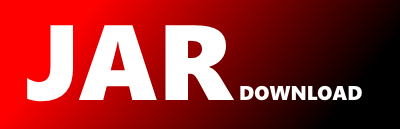
org.unipop.schema.property.StaticPropertySchema Maven / Gradle / Ivy
package org.unipop.schema.property;
import org.apache.tinkerpop.gremlin.process.traversal.P;
import org.unipop.query.predicates.PredicatesHolder;
import org.unipop.query.predicates.PredicatesHolderFactory;
import org.unipop.schema.property.type.PropertyType;
import java.util.Collections;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
public class StaticPropertySchema implements PropertySchema {
protected final String key;
protected final String value;
public StaticPropertySchema(String key, String value) {
this.key = key;
this.value = value;
}
@Override
public String getKey() {
return key;
}
@Override
public Map toProperties(Map source) {
return Collections.singletonMap(key, this.value);
}
@Override
public Map toFields(Map prop) {
Object o = prop.get(this.key);
if(o == null || o.equals(this.value)) return Collections.emptyMap();
return null;
}
@Override
public Set toFields(Set propertyKeys) {
return Collections.emptySet();
}
@Override
public Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy