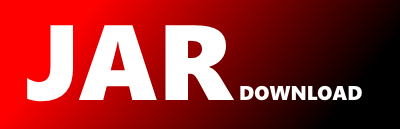
org.unipop.util.ConversionUtils Maven / Gradle / Ivy
package org.unipop.util;
import org.apache.tinkerpop.gremlin.structure.util.ElementHelper;
import org.json.JSONArray;
import org.json.JSONObject;
import java.util.*;
import java.util.function.BiFunction;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
public class ConversionUtils {
public static Stream asStream(Iterator sourceIterator) {
return asStream(sourceIterator, false);
}
public static Stream asStream(Iterator sourceIterator, boolean parallel) {
Iterable iterable = () -> sourceIterator;
return StreamSupport.stream(iterable.spliterator(), parallel);
}
public static Set toSet(JSONObject config, String key) {
JSONArray objects = config.optJSONArray(key);
if (objects != null) {
HashSet hashSet = new HashSet<>(objects.length());
for (int i = 0; i < objects.length(); i++)
hashSet.add((T) objects.get(i));
return hashSet;
}
Object opt = config.opt(key);
if (opt != null) return Collections.singleton((T) opt);
return Collections.EMPTY_SET;
}
public static Map asMap(Object[] keyValues){
Map map = new HashMap<>();
if (keyValues != null) {
ElementHelper.legalPropertyKeyValueArray(keyValues);
for (int i = 0; i < keyValues.length; i = i + 2) {
String key = keyValues[i].toString();
Object value = keyValues[i + 1];
ElementHelper.validateProperty(key,value);
if (map.containsKey(key)){
Object o = map.get(key);
List list = o instanceof List ? ((List) o) : new ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy