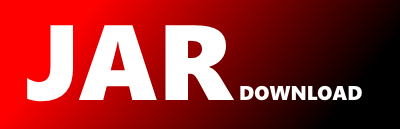
org.unipop.rest.util.matchers.Matcher Maven / Gradle / Ivy
package org.unipop.rest.util.matchers;
import org.apache.tinkerpop.gremlin.process.traversal.step.util.HasContainer;
import org.json.JSONObject;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
/**
* Created by sbarzilay on 12/7/16.
*/
public interface Matcher {
boolean match(HasContainer hasContainer);
String execute(HasContainer hasContainer);
default Map toMap(HasContainer hasContainer) {
HashMap map = new HashMap<>();
String[] split = hasContainer.getKey().split("\\.");
map.put("key", split[split.length - 1]);
Object value = hasContainer.getValue();
if (value instanceof String)
map.put("value", "\"" + value + "\"");
else if (value instanceof Collection) {
String finalString = "[%s]";
List values = ((Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy