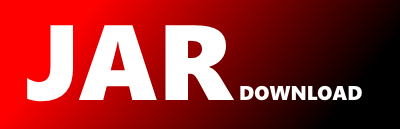
com.github.uuidcode.builder.html.Node Maven / Gradle / Ivy
package com.github.uuidcode.builder.html;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class Node {
public static final String INDENT = " ";
private List childNodeList = new ArrayList<>();
private List attributeList = new ArrayList<>();
private String tagName;
private Node parentNode;
private boolean requiresEndTag = true;
public boolean getRequiresEndTag() {
return this.requiresEndTag;
}
public T setRequiresEndTag(boolean requiresEndTag) {
this.requiresEndTag = requiresEndTag;
return (T) this;
}
public List getChildNodeList() {
return childNodeList;
}
public Node getParentNode() {
return this.parentNode;
}
public Node setParentNode(Node parentNode) {
this.parentNode = parentNode;
return this;
}
public T add(Node node) {
if (node == null) {
return (T) this;
}
node.setParentNode(this);
this.childNodeList.add(node);
return (T) this;
}
public T add(Node... node) {
return add(Arrays.asList(node));
}
public T add(List nodeList) {
nodeList.stream().forEach(this::add);
return (T) this;
}
public T setTagName(String tagName) {
this.tagName = tagName;
return (T) this;
}
public String html() {
return Utils.joiningWithLineSeparator(this.contentList());
}
public List contentList() {
List contentList = new ArrayList<>();
contentList.add(Utils.startTag(this.tagName + this.attribute()));
contentList.addAll(this.getChildContentList());
if (this.requiresEndTag) {
contentList.add(Utils.endTag(this.tagName));
}
return this.shrink(contentList);
}
private String attribute() {
if (this.attributeList.size() == 0) {
return "";
}
return " " + this.attributeList.stream()
.map(Attribute::toString)
.collect(Collectors.joining(" "));
}
private List getChildContentList() {
Stream> listStream = this.childNodeList.stream()
.map(Node::contentList);
return listStream
.map(this::shrink)
.map(this::processIndent)
.flatMap(List::stream)
.collect(Collectors.toList());
}
private List processIndent(List list) {
if (this.childNodeList.size() <= 1 && list.size() < 3) {
return list;
}
return prependIndent(list);
}
private List prependIndent(List list) {
return list.stream()
.map(this::prependIndent)
.collect(Collectors.toList());
}
private String prependIndent(String content) {
return INDENT + content;
}
private String joining(List list) {
return list.stream().collect(Collectors.joining());
}
private List joiningAndAsList(List list) {
return Arrays.asList(this.joining(list));
}
public List shrink(List list) {
if (list.size() <= 3) {
return this.joiningAndAsList(list);
}
return list;
}
public T addAttribute(Attribute newAttribute) {
this.attributeList.stream()
.filter(attribute -> attribute.getName().equals(newAttribute.getName()))
.findFirst()
.map(attribute -> attribute.getValueSet().addAll(newAttribute.getValueSet()))
.orElseGet(() ->this.attributeList.add(newAttribute));
return (T) this;
}
public T setId(String id) {
this.attributeList.add(Attribute.of("id", id));
return (T) this;
}
public T addClass(String className) {
return this.addAttribute(Attribute.of("class", className));
}
public T addClass(String... className) {
for (String name : className) {
this.addClass(name);
}
return (T) this;
}
public T addStyle(String style) {
return this.addAttribute(Attribute.of("style", style));
}
public T setType(String type) {
this.attributeList.add(Attribute.of("type", type));
return (T) this;
}
public T setPlaceholder(String placeholder) {
this.attributeList.add(Attribute.of("placeholder", placeholder));
return (T) this;
}
public T setName(String name) {
this.attributeList.add(Attribute.of("name", name));
return (T) this;
}
public T setValue(String value) {
this.attributeList.add(Attribute.of("value", value));
return (T) this;
}
public T setHref(String href) {
this.attributeList.add(Attribute.of("href", href));
return (T) this;
}
public T setRel(String rel) {
this.attributeList.add(Attribute.of("rel", rel));
return (T) this;
}
public T setFor(String value) {
this.attributeList.add(Attribute.of("for", value));
return (T) this;
}
public T stylesheet() {
return this.setRel("stylesheet");
}
public T password() {
return this.setType("password");
}
public T text() {
return this.setType("text");
}
public T submit() {
return this.setType("submit");
}
public T hidden() {
return this.setType("hidden");
}
public Node setDisabled(boolean disabled) {
if (disabled) {
this.attributeList.add(Attribute.of("disabled"));
}
return (T) this;
}
public T setSrc(String src) {
this.attributeList.add(Attribute.of("src", src));
return (T) this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy